Open Sushisource opened 6 years ago
This is a bug. Could you provide more details on the exact location and dimensions of the walls for the example that fails? That would help me reproduce the bug.
While fixing #210, I found a bug that may be related to this issue. @Sushisource Could you please check if your examples still fail with ncollide2d v0.16.1 ?
@sebcrozet Thanks, I'll give that a shot. Might take me a bit because I'm using ggez for the rendering and they are pointing at older nalgebra still which is causing some compat issues. As soon as they update or I work around them I'll let you know.
@sebcrozet Was able to get everything compiling and this is indeed fixed in 0.16.1. Thanks!
I've been trying to use a time-of-impact query to slide two compound shapes together, so they are exactly touching, and then move them one wall-thickness along the normal so the walls overlap completely, but I've not had much success. After re-runing a contact query the penetration depths are always nearly exactly correct, but I'll still end up sometimes with things not quite lining up just right visually.
Would that approach be expected to work? I think I may just switch to an explicit grid for this particular task. Thanks again!
@Sushisource Since ncollide does not implement a dedicated box/box algorithm for distance and penetration depth computation yet, there might be some cases where the generic iterative algorithms don't yield the exact results because of lack of convergence. So you may be experiencing this.
If you can give me a way to reproduce the bug your are experiencing (for example if your code and the steps to reproduce the issue are available somehow), I could take a look and see if there is still a bug somewhere on ncollide.
I have found that penetration queries seem to only sometimes work for compound shapes against compound shapes. I'm not sure what the circumstance is when they do work, but I have at least one test that consistently fails where it should seemingly return a penetration value:
In this test the north wall of the first room overlaps the south wall of the second. The walls are 0.2 thick.
But the test fails.
For how the "Room" is constructed:
Here is a picture of the rooms where walls are a not-fully-opaque green, so you can see the overlap as a slightly brighter green: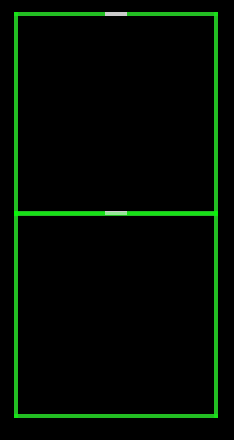
Am I doing something wrong here? Or is this a bug for compound shapes?