Open jakublabno opened 1 year ago
Thank you for providing a code example! That makes it easier to understand the situation.
As a first step, could you please try to simplify the code a bit more?
DateTimeTrait
, and do you need it to show the problem?
Bug Report
Summary
Invalid parameters binding while persisting new entity with OneToOneRelation
Current behavior
I have two entities:
Invoice
andInvoiceNumeration
While I'm creating
Invoice
entity, doctrine throws a pdo exceptionWhen checking deeper, it seems id is not being passed to PDO, I see null there.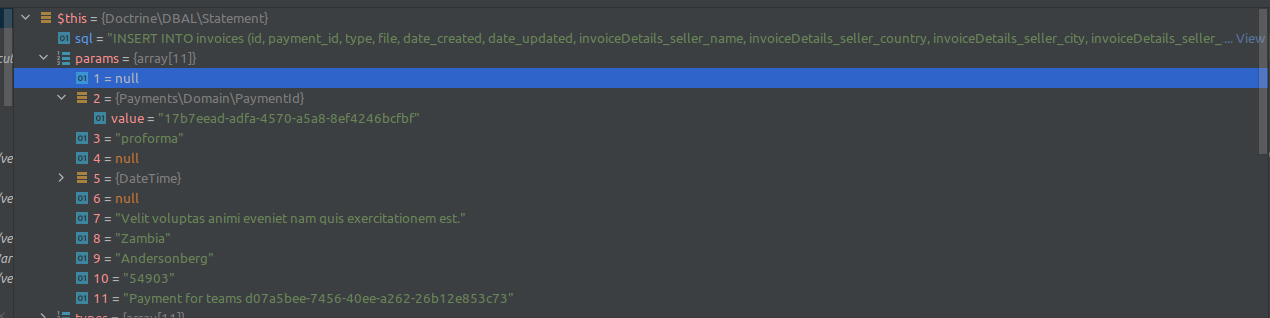
How to reproduce
Here is DDL:
Of course, everything worked before adding OneToOne and JoinColumn definition. Maybe I'm doing something wrong?
I would not use JoinColumn, because it seems not be necessary, but when I don't it throws another exception
However, it's the same type as in
Invoice
Entity Id