Open kierancrown opened 1 year ago
I'm looking for this solution too 😔
I hope this solution could help you,
In my case, This issue was when I added data that could be updated after the first render, The solution was I create a new component that home this data and wrapped it on horizontal scrollView and It works
Describe the bug I'm attempting to to create a component where the user can scroll back through data and as they do so more data loads in and gets appended to the data array. However when I do this I noticed that the items change order. I'm looking for a way to have the new items load in but keep the current index. I attempted this by calling the
scrollTo
function with animation set to false just after adding the new data. However this causes the component to render twice and the user see's a flash during this process. I'm wondering if there is a way to achieve this without flashing.Here is a basic example of the code I'm using:
To Reproduce Steps to reproduce the behavior:
react-native-carousel
projectExpected behavior The component to not flash when adding data and updating the index (some way to freeze the updates...)
Screenshots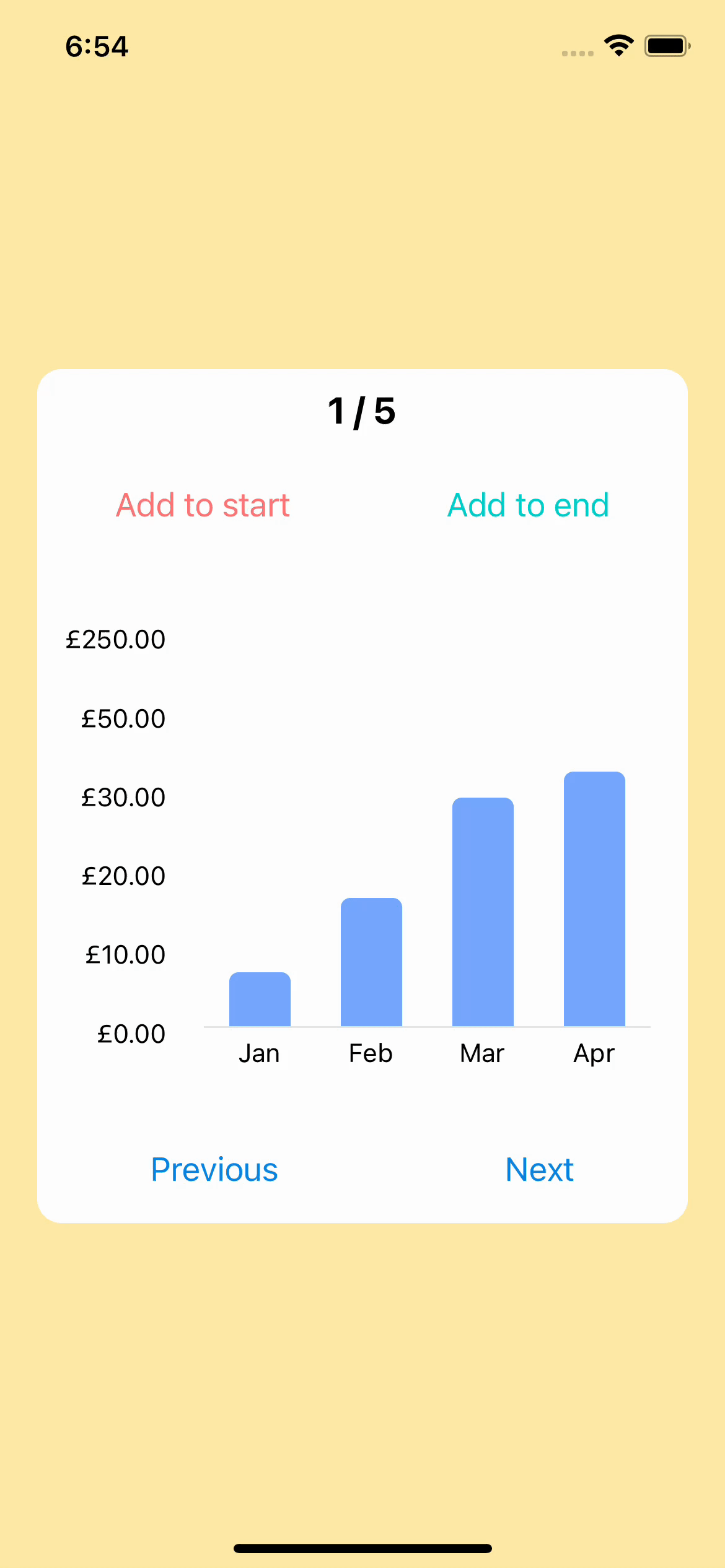
Versions (please complete the following information):
Smartphone (please complete the following information):