Closed vined-underscore closed 1 year ago
Sending the help command in a DM doesn't spam it instantly but sending it in a channel does.
For some reason removing this:
if msg.author == self.bot.user:
if msg.content.startswith(f"{main.prefix}help"):
await msg.edit(delete_after = 15.5)
in the on_command event fixed it.
Summary
When using a custom help command in discord.py-self 1.9.2, it used to work normally, but after updating to 2.0.0a2, it stopped working and using an
on_command
event it just spams the command in the logging. (After a while it spams the help command on Discord too)Reproduction Steps
Overriding the default help command and loading it as a cog.
Code
on_command
event:Help command:
Expected Results
Help command sends once.
Actual Results
The help command gets spammed in the logging and after a few minutes it gets spammed in the chat too.
System Information
Checklist
Additional Information
There is no traceback. I am using selfcord but it also did the same thing with discord.py-self 2.0.0a2.
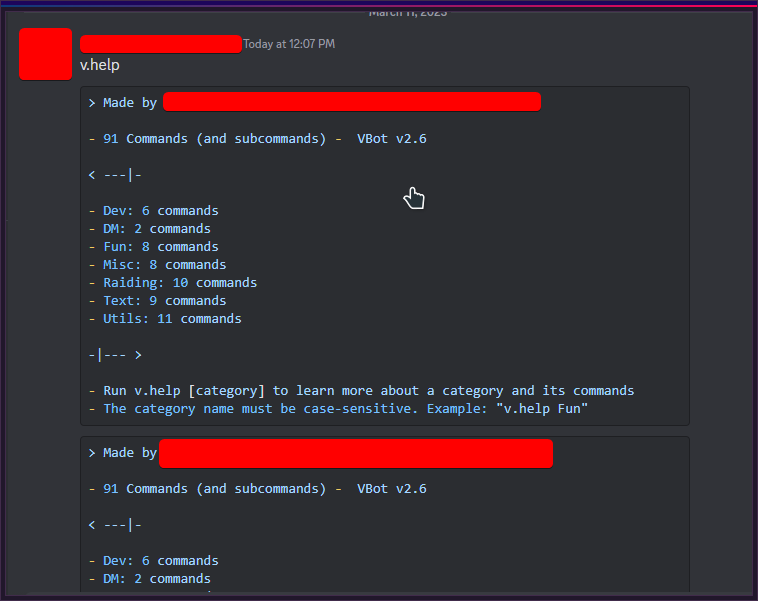