Closed antomys closed 2 years ago
Instead of the StringBuilder
it could be based on ValueStringBuilder
.
This does essentially the same as your proposal, but encapsulated within in a type, so the usage is more streamlined and virtually the same as now.
Yes, you are right, @gfoidl. It would be much better to use ValueStringBuilder
. But as i was investigating new possibilities of improving QueryBuilder
, I was unable to use ValueStringBuilder
because of internal
modifier. As they will be in same assembly, it would be better to use it and not add additional proposed by me overhead
ValueStringBuilder
can be used by adding it manually to the csproj from the shared source folder like we do here:
Thanks @halter73 for hint! I will do a PR with a suggestion and link this issue to it
Is there an existing issue for this?
Is your feature request related to a problem? Please describe the problem.
This is not a problem, just a suggestion for improving QueryBuilder by using stack, arrayPool and span manipulations
Describe the solution you'd like
Suggesting to use stackalloc or ArrayPool while building query for improving performance. Also storing count of overall values.
QueryBuilder as is:
QueryBuilde could be:
Additional context
Why so? Benefits:
StringBuilder
every timeDrawbacks:
Count
that holds amount of entities inside of aList<KeyValuePair>
.Count += parameters.Sum(pair => pair.Key.Length + pair.Value.Length);
in a constructor with a parameter ofList<KeyValuePair>
can be somewhat irrational.=
and& or ?
As for benchmark results:
NOTE: QueryAspNetCore - current AspNet solution. QueryCustomBuilder - implementation with suggested solution
This approach gives is faster by approx 13% for 1 query and for average 2 approx 18%. Speaking about memory, for 1 query we have 45% less used memory and for 2 - 55%
See plots:
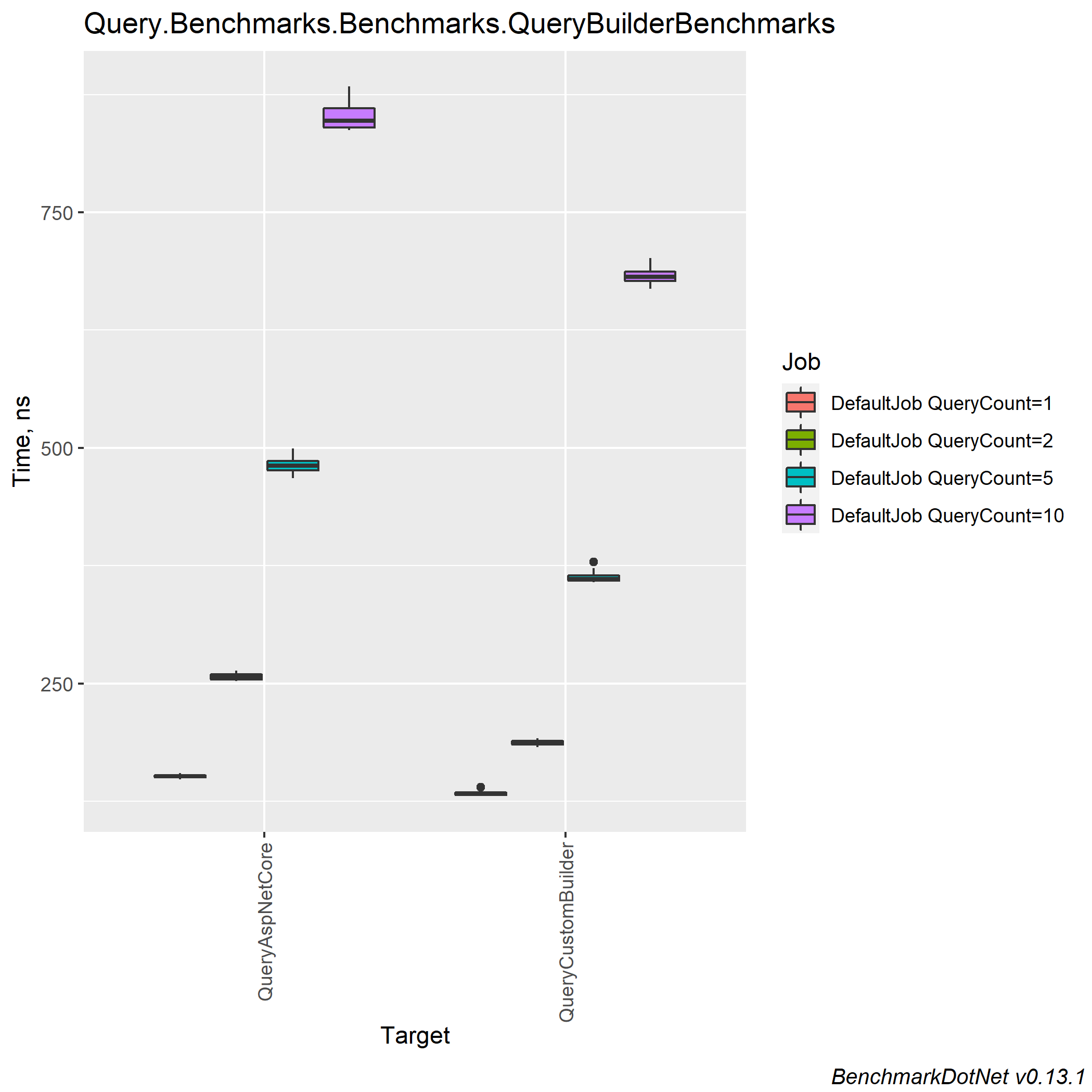