Open alexandrevribeiro opened 2 years ago
Related to https://github.com/dotnet/efcore/issues/16926
For tracking queries we need to select the owner (the document in this case), however this shouldn't be necessary for non-tracking queries
+1 for this. We're observing the same issue with a rational provider (we're using Npgsql.EntityFrameworkCore.PostgreSQL v7.0).
I would also +1 this as well
I'm using Microsoft.EntityFrameworkCore.Cosmos 6.0.1 to perform queries against a Cosmos DB, but the problem I'm facing is that whenever I include in the
Select
any property that is either an object or a collection/array (mapped as "OwnsOne" and "OwnsMany"), instead of querying only the selected properties, it actually selects the entire document for EF to be able to select the complex/collection property.Code sample
Entities
Entity type configuration
Queries sample and Results
Query sample 1) Selecting only simple properties
Query sample:
Result:
Note that only the
c["id"]
andc["identifierNumber"]
were selected, as expected.Query sample 2) Selecting a complex object property (city)
Query sample:
Result:
In this case, the entire document is being selected (represented in the result as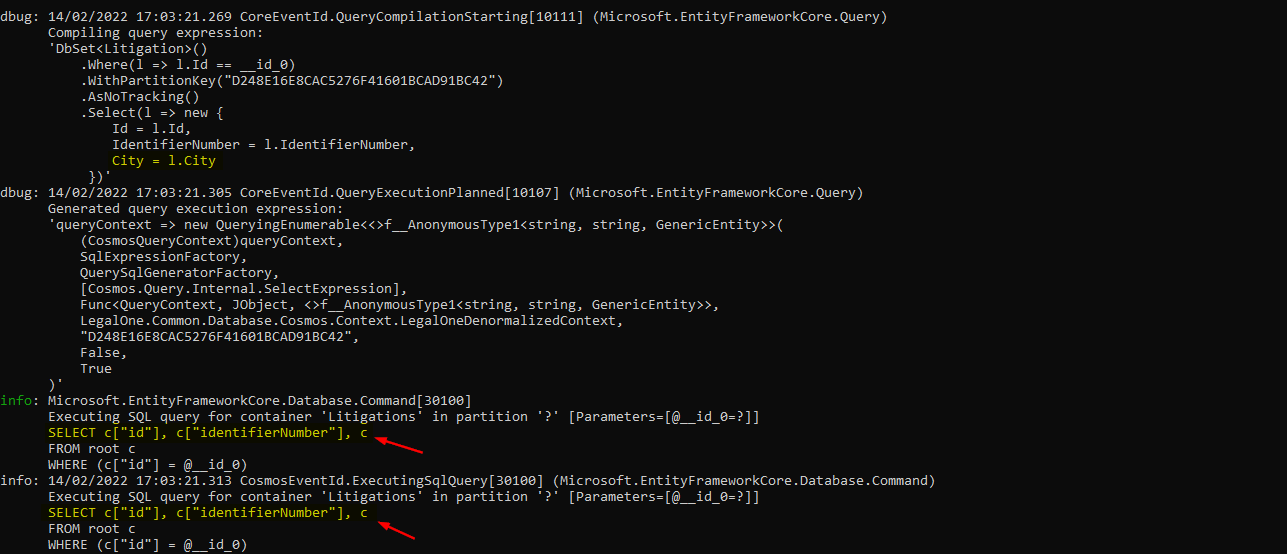
c
), which shouldn't happen. If I copy and past this query on Azure Portal Cosmos Data Explorer, I can see it is indeed returning the entire document:Query sample 3) Selecting a collection/array property (subjects)
Query sample:
Result:
The same behavior happening when selecting a complex object is also happening with a collection property: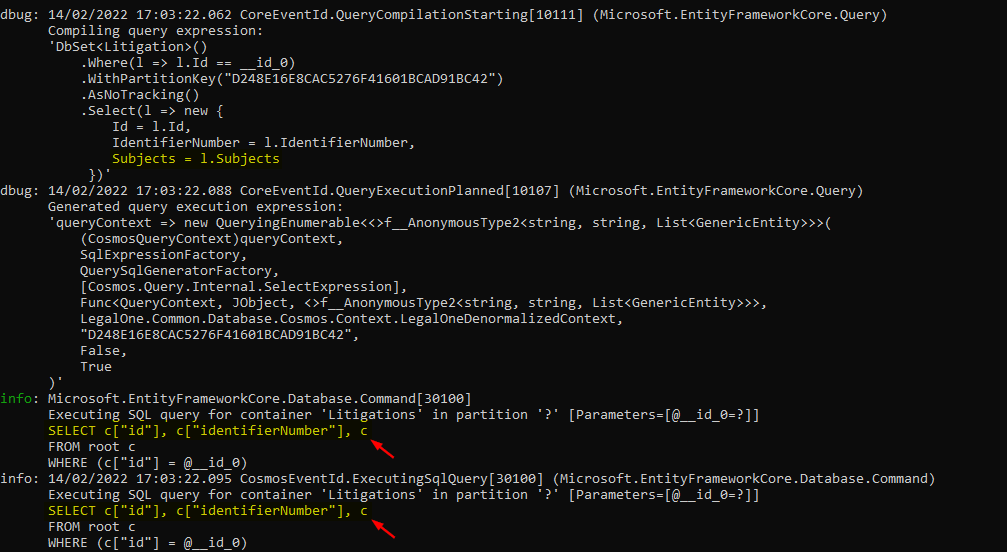
Provider and version information
EF Core version: 6.0.1 Database provider: Microsoft.EntityFrameworkCore.Cosmos 6.0.1 Target framework: .NET 6.0 Operating system: Windows IDE: Visual Studio Professional 2022