Open EPS-Lac opened 2 years ago
Similar issue on Xamarin https://github.com/xamarin/Xamarin.Forms/issues/11446 opened July 2022 assigned to @PureWeen
Ah, thanks! I did look through the MAUI issues to see if there is already a ticket for this, but didn't think of checking the Xamarin.Forms. But since the other one is still open (created almost two years ago) I don't think this will get any attention... At least not in Xamarin.Forms anymore. Hopefully there will be a fix at some point for MAUI.
verified repro on IOS15.4.
Same on Android.
I also have both set to invisible Shell.NavBarIsVisible="false" Shell.TabBarIsVisible="false"
and add Click/Tap on "More" both gets visible.
.NET 7.0.5 Android 9.0
Verified this issue with Visual Studio Enterprise 17.8.0 Preview 1.0. Can repro on iOS platform with sample code.
related #18193
Will be fixed by https://github.com/dotnet/maui/pull/22655
Description
Context
I have a project where I want to use a MAUI Shell application with a TabBar. Since the customization of the default Shell.TabBar is extremely limited I created a custom TabBar control and hide the Shell.TabBar on all pages. I still did add the pages to the in the AppShell.xaml though and noticed a strange behaviour that most likely is a bug.
Bug Description
When there are more than 5 elements in a Shell.TabBar, the additional tabs are added to a "more" button/tab (as supposed to and described in the docs). With the Shell.TabBarIsVisible and the Shell.NavBarIsVisible set to false, both elements are hidden from the user in the normal case, BUT if you navigate to a route that would be placed under the "more" button, the TabBar becomes visible and the "more" menu is opened up, instead of just opening the page and keep the TabBar hidden. In addition, once you click on the intended page again inside the "more" menu that pops up to get to that page, the NavBar (< more) is visible again.
This only happens on iOS, on Android everything works as I would expect (the page opens up, TabBar and More menu stay hidden, NavBar is hidden as well).
Screenshots SampleProject
There are 6 Tabs in the sample project, all having the MainPage.xml as their page to simplify the code, with six Buttons to navigate to the different pages when the TabBar is hidden. Note: The iOS screenshots are done using the iOS simulator, but it's the same when using a real iOS (tested with iPhone 12).
With TabBar and NavBar visible (for reference):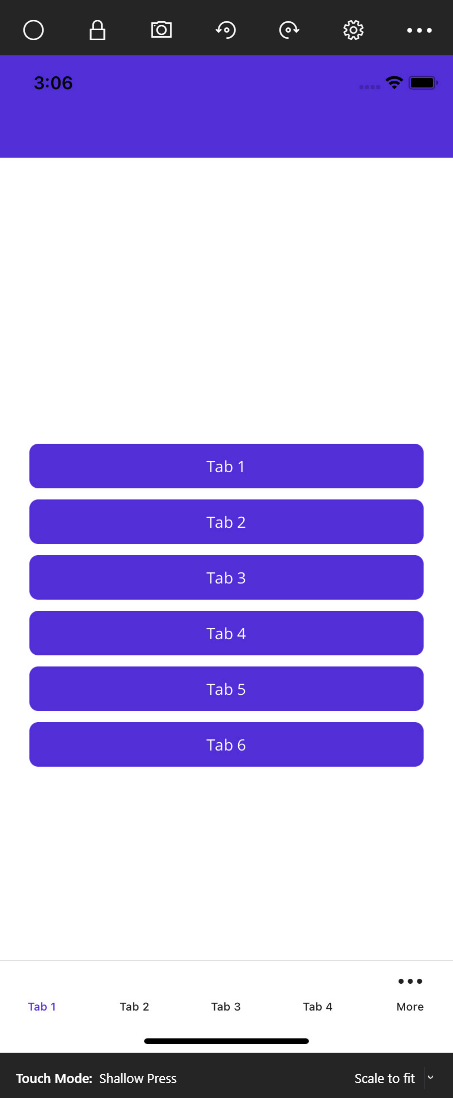
With TabBar and NavBar hidden while clicking on any tab from 1-4: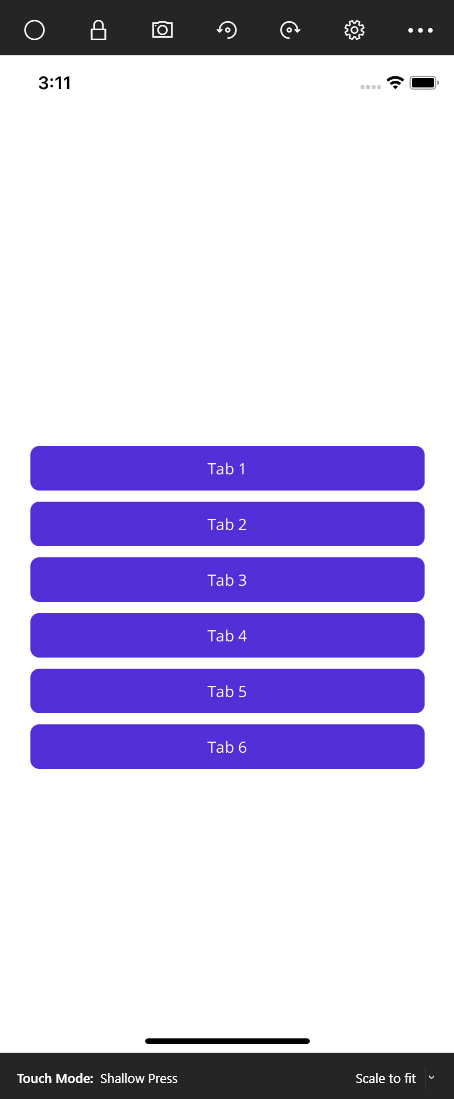
When clicking on tab 5 or 6 (still with TabBar and NavBar hidden):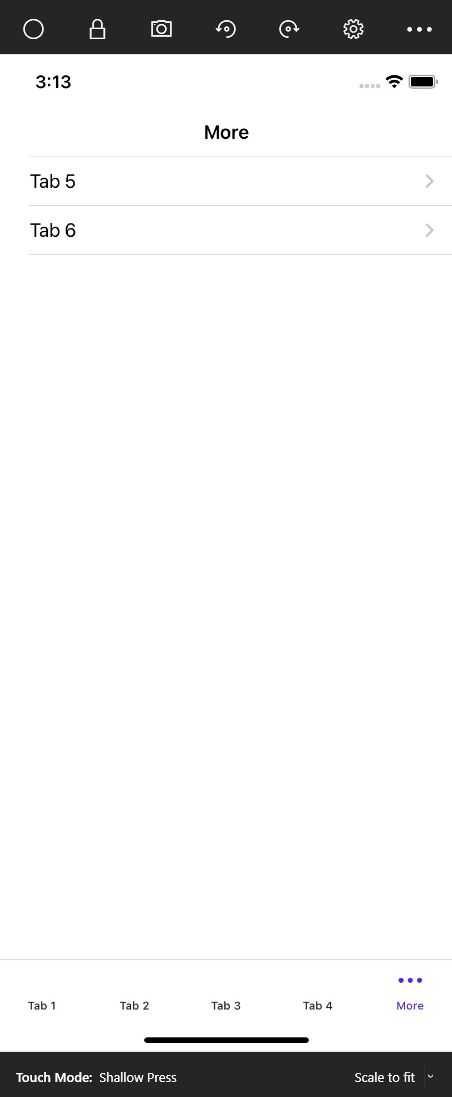
After clicking on tab 5 in the "more" menu that opened up (see previous screenshot), while in tab 5:
When you click the "back" navigation you get to the "more" menu again and can press another tab from there. As soon as you're back on tab 1-4 the TabBar and NavBar are hidden again. It's just within those "more" tabs.
For Android, all Tabs from 1-6 behave and look the same. No "more" menu popping up, NavBar and TabBar stay hidden as expected. Tab 5 on Android (same for all tabs):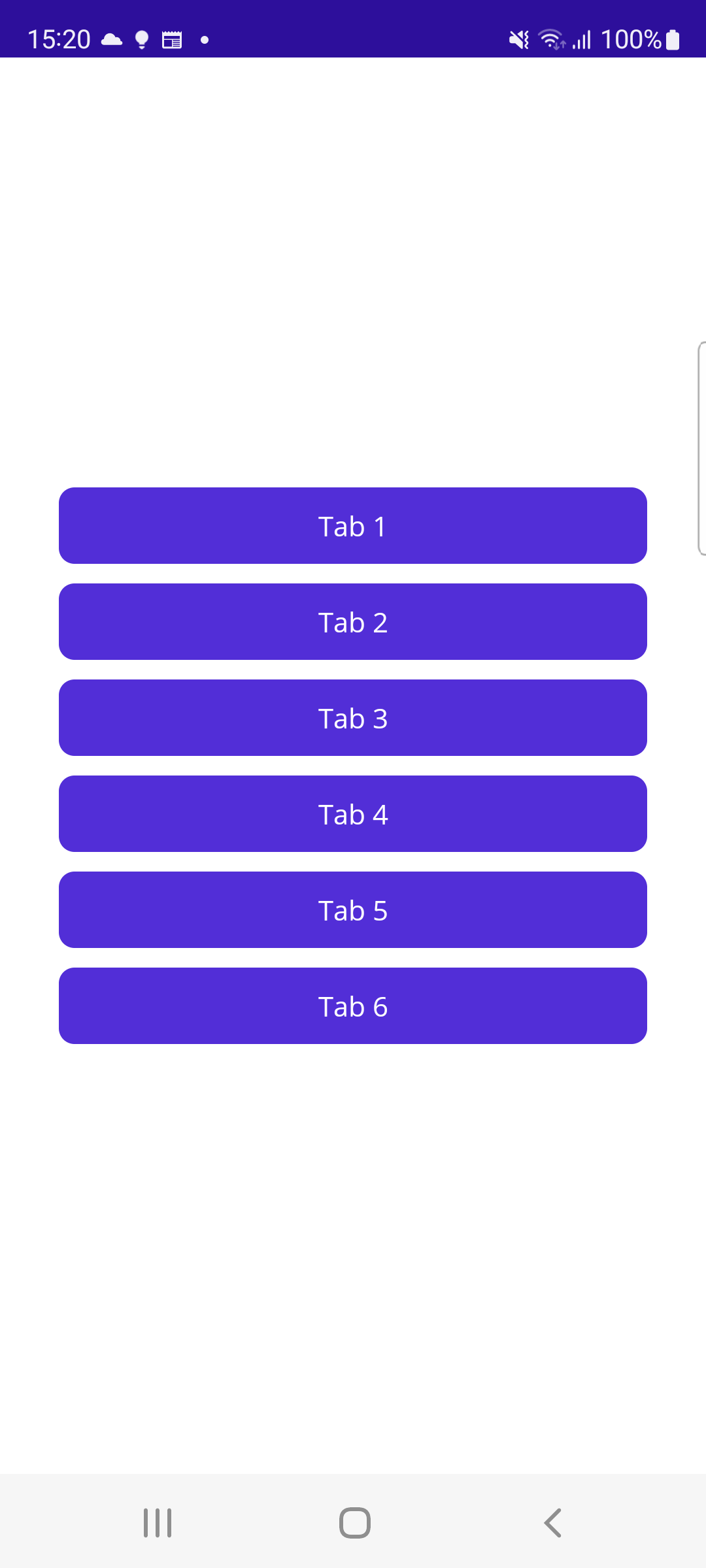
Steps to Reproduce
AppShell.xaml
MainPage.xaml
MainPage.xaml.cs
Version with bug
6.0 (current)
Last version that worked well
Unknown/Other
Affected platforms
iOS
Affected platform versions
iOS 15.5
Did you find any workaround?
Keep the items in the TabBar less than 5, so no "more" tab menu is required. Add pages that don't need to be in the TabBar (in my case all, since I have the TabBar hidden all the time anyways) as simple ShellItems outside of the TabBar (you might need to keep at least 1 page in the TabBar for it to still work, not sure.)
For my project, I've added them in the AppShell.xaml.cs like this:
And in the AppShell.xaml I have a TabBar named TabBar:
Relevant log output
No response