In the code example below, the analyzer assumes that the second if-condition int the set-sections in Model and Owner properties can never be met, considering this code to be "dead"
Create a simple class (see the code example below)
See CA1508 warning in the second if-branch in the Owner and Model properties
Sample
public class Vehicle
{
private string model;
private string owner;
public Vehicle(string model, string owner)
{
this.Model = model;
this.Owner = owner;
}
public string Model
{
get => this.model;
private set
{
if (value is null)
{
throw new ArgumentNullException(nameof(value));
}
if (string.IsNullOrWhiteSpace(value))
{
throw new ArgumentException($"Model cannot be empty or whitespace", nameof(value));
}
this.model = value;
}
}
public string Owner
{
get => this.owner;
set
{
if (value is null)
{
throw new ArgumentNullException(nameof(value));
}
if (string.IsNullOrWhiteSpace(value))
{
throw new ArgumentException($"Owner cannot be empty or whitespace", nameof(value));
}
this.owner = value;
}
}
}
Analyzer
Diagnostic ID: CA1508:
Avoid dead conditional code
Analyzer source
SDK: Built-in CA analyzers in .NET 5 SDK or later
Version: SDK 5.0.302
AND
NuGet Package: Microsoft.CodeAnalysis.NetAnalyzers
Version: 1.1.118 (Latest)
Describe the bug
In the code example below, the analyzer assumes that the second if-condition int the set-sections in Model and Owner properties can never be met, considering this code to be "dead"
Steps To Reproduce
Provide the steps to reproduce the behavior:
Sample
Expected behavior
No CA1508.
Actual behavior
CA1508 false positive.
Additional context
I went into this branch of code using a debugger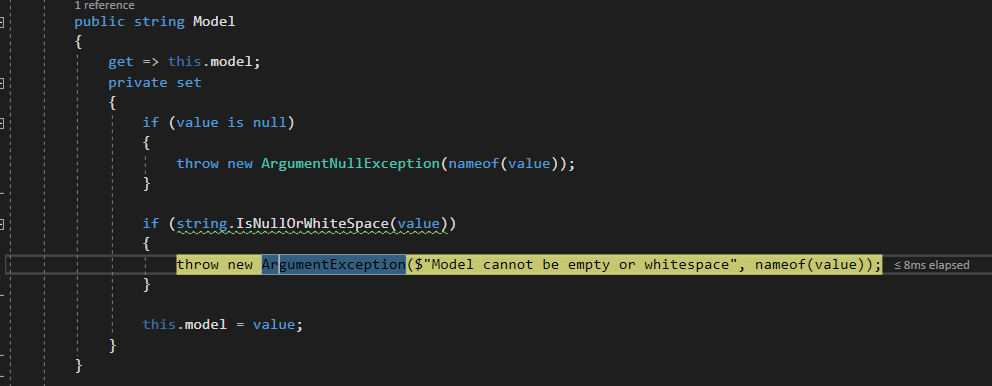