Working on writing tests, and have a situation where I have reusable test code that I want to run with different fixtures (managing different user configurations). To manage this, I'm making an abstract *TestsBase class with all tests listed in it, and 2 wrapper classes with different fixture. Here is an example layout similar to what I'm trying to do (4 tests in base class):
Screenshot from VSCode
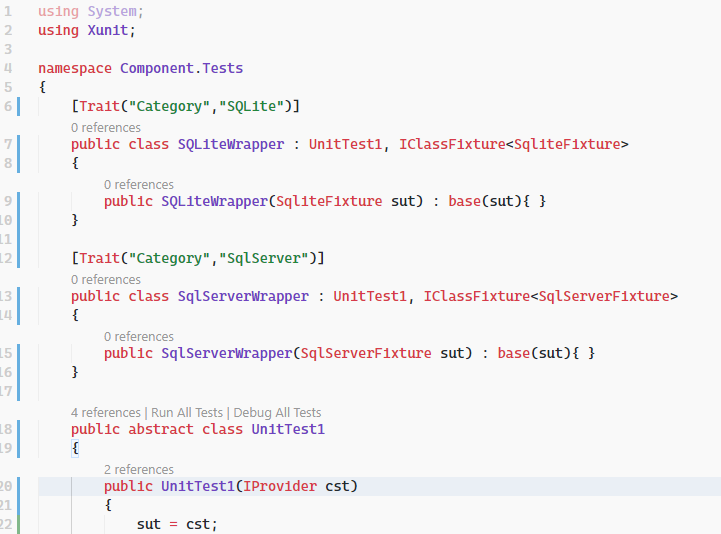
When I run tests via dotnet test or a test runner (VS Test Explorer, and this VS Code Extension (recommended by Joey R.), it discovers all tests (8 total), and the test explorer shows a correctly organized view:
Test Explorer Screenshot
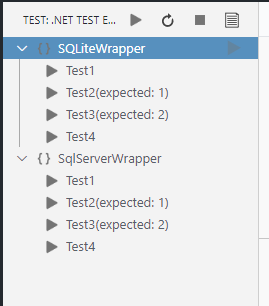
However, the Code Lens Run/Debug All Tests are not connecting to the wrappers with Fixtures (see first picture). The Run/Debug on the base class doesn't return any tests, since it is an abstract class.
Steps to Reproduce
Make an abstract class with 1/many public test in it (4 in example). Make 2 public wrapper classes (put traits/IClassFixtures on them to recreate). Running dotnet test will run 2x the amount of tests in your base class (8 tests ran in my example).
Code snippet
```
using System;
using Xunit;
namespace Component.Tests
{
[Trait("Category","SQLite")]
public class SQLiteWrapper : UnitTest1, IClassFixture
{
public SQLiteWrapper(SqliteFixture sut) : base(sut){ }
}
[Trait("Category","SqlServer")]
public class SqlServerWrapper : UnitTest1, IClassFixture
{
public SqlServerWrapper(SqlServerFixture sut) : base(sut){ }
}
public abstract class UnitTest1
{
public UnitTest1(IProvider cst)
{
sut = cst;
}
IProvider sut;
[Fact]
public void Test1()
{
Assert.Equal(1,1);
}
[Theory]
[InlineData(1)]
public void Test2(int expected)
{
Assert.Equal(expected,sut.MyInt);
}
[Theory]
[InlineData(2)]
public void Test3(int expected)
{
Assert.Equal(expected,sut.OurInt);
}
[Fact]
public void Test4()
{
int sum = sut.MyInt + sut.OurInt;
Assert.Equal(3,sum);
}
}
}
```
Expected Behavior
Code Lens Run/Debug All Tests Option should connect to derived classes that inherit from a class with Tests in it.
Actual Behavior
Code Lens only connects to the base class, even if it's abstract. It does not connect to the derived classes.
Logs
Didn't grab since I don't think it's necessary, but I can come back and upload if needed.
Environment information
VSCode version: 1.48.0
C# Extension: 1.23.1
Dotnet Information
.NET Core SDK (reflecting any global.json):
Version: 3.1.400
Commit: 035fb2aa2f
Runtime Environment:
OS Name: Windows
OS Version: 10.0.19041
OS Platform: Windows
RID: win10-x64
Base Path: C:\Program Files\dotnet\sdk\3.1.400\
Host (useful for support):
Version: 3.1.6
Commit: 3acd9b0cd1
.NET Core SDKs installed:
3.1.400 [C:\Program Files\dotnet\sdk]
.NET Core runtimes installed:
Microsoft.AspNetCore.All 2.1.20 [C:\Program Files\dotnet\shared\Microsoft.AspNetCore.All]
Microsoft.AspNetCore.App 2.1.20 [C:\Program Files\dotnet\shared\Microsoft.AspNetCore.App]
Microsoft.AspNetCore.App 3.1.6 [C:\Program Files\dotnet\shared\Microsoft.AspNetCore.App]
Microsoft.NETCore.App 2.1.20 [C:\Program Files\dotnet\shared\Microsoft.NETCore.App]
Microsoft.NETCore.App 3.1.6 [C:\Program Files\dotnet\shared\Microsoft.NETCore.App]
Microsoft.WindowsDesktop.App 3.1.6 [C:\Program Files\dotnet\shared\Microsoft.WindowsDesktop.App]
To install additional .NET Core runtimes or SDKs:
https://aka.ms/dotnet-download
Visual Studio Code Extensions
|Extension|Author|Version|
|---|---|---|
|cpptools|ms-vscode|0.29.0|
|csharp|ms-dotnettools|1.23.1|
|csharpextensions|jchannon|1.3.1|
|debugger-for-edge|msjsdiag|1.0.15|
|docomment|k--kato|0.1.18|
|dotnet-test-explorer|formulahendry|0.7.4|
|EditorConfig|EditorConfig|0.15.1|
|github-sharp-theme|joaomoreno|1.4.0|
|markdown-all-in-one|yzhang|3.2.0|
|remote-containers|ms-vscode-remote|0.134.0|
|remote-ssh|ms-vscode-remote|0.51.0|
|remote-ssh-edit|ms-vscode-remote|0.51.0|
|remote-wsl|ms-vscode-remote|0.44.4|
|rest-client|humao|0.24.1|
|vscode-arduino|vsciot-vscode|0.3.1|
|vscode-docker|ms-azuretools|1.5.0|
|vscode-drawio|hediet|0.7.2|
|vscode-remote-extensionpack|ms-vscode-remote|0.20.0|
|vscode-xml|redhat|0.13.0|
|vscodeintellicode|VisualStudioExptTeam|1.2.10|
|vsliveshare|ms-vsliveshare|1.0.2666|
|vsliveshare-audio|ms-vsliveshare|0.1.85|
|vsliveshare-pack|ms-vsliveshare|0.4.0|
|vsonline|ms-vsonline|1.0.2669|;
Unrelated note
This is honestly the smoothest "Report an issue" workflow I've ever experienced. Kudos.
Issue Description
Working on writing tests, and have a situation where I have reusable test code that I want to run with different fixtures (managing different user configurations). To manage this, I'm making an abstract *TestsBase class with all tests listed in it, and 2 wrapper classes with different fixture. Here is an example layout similar to what I'm trying to do (4 tests in base class):
Screenshot from VSCode
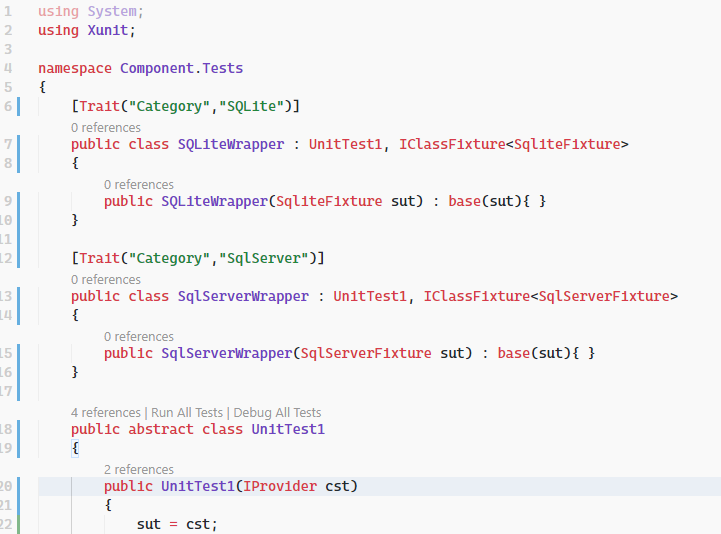When I run tests via dotnet test or a test runner (VS Test Explorer, and this VS Code Extension (recommended by Joey R.), it discovers all tests (8 total), and the test explorer shows a correctly organized view:
Test Explorer Screenshot
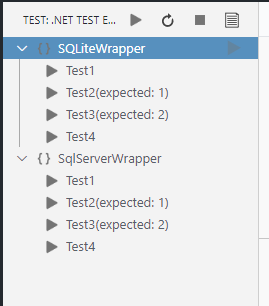However, the Code Lens Run/Debug All Tests are not connecting to the wrappers with Fixtures (see first picture). The Run/Debug on the base class doesn't return any tests, since it is an abstract class.
Steps to Reproduce
Make an abstract class with 1/many public test in it (4 in example). Make 2 public wrapper classes (put traits/IClassFixtures on them to recreate). Running dotnet test will run 2x the amount of tests in your base class (8 tests ran in my example).
Code snippet
``` using System; using Xunit; namespace Component.Tests { [Trait("Category","SQLite")] public class SQLiteWrapper : UnitTest1, IClassFixtureExpected Behavior
Code Lens Run/Debug All Tests Option should connect to derived classes that inherit from a class with Tests in it.
Actual Behavior
Code Lens only connects to the base class, even if it's abstract. It does not connect to the derived classes.
Logs
Didn't grab since I don't think it's necessary, but I can come back and upload if needed.
Environment information
VSCode version: 1.48.0 C# Extension: 1.23.1
Dotnet Information
.NET Core SDK (reflecting any global.json): Version: 3.1.400 Commit: 035fb2aa2f Runtime Environment: OS Name: Windows OS Version: 10.0.19041 OS Platform: Windows RID: win10-x64 Base Path: C:\Program Files\dotnet\sdk\3.1.400\ Host (useful for support): Version: 3.1.6 Commit: 3acd9b0cd1 .NET Core SDKs installed: 3.1.400 [C:\Program Files\dotnet\sdk] .NET Core runtimes installed: Microsoft.AspNetCore.All 2.1.20 [C:\Program Files\dotnet\shared\Microsoft.AspNetCore.All] Microsoft.AspNetCore.App 2.1.20 [C:\Program Files\dotnet\shared\Microsoft.AspNetCore.App] Microsoft.AspNetCore.App 3.1.6 [C:\Program Files\dotnet\shared\Microsoft.AspNetCore.App] Microsoft.NETCore.App 2.1.20 [C:\Program Files\dotnet\shared\Microsoft.NETCore.App] Microsoft.NETCore.App 3.1.6 [C:\Program Files\dotnet\shared\Microsoft.NETCore.App] Microsoft.WindowsDesktop.App 3.1.6 [C:\Program Files\dotnet\shared\Microsoft.WindowsDesktop.App] To install additional .NET Core runtimes or SDKs: https://aka.ms/dotnet-downloadVisual Studio Code Extensions
|Extension|Author|Version| |---|---|---| |cpptools|ms-vscode|0.29.0| |csharp|ms-dotnettools|1.23.1| |csharpextensions|jchannon|1.3.1| |debugger-for-edge|msjsdiag|1.0.15| |docomment|k--kato|0.1.18| |dotnet-test-explorer|formulahendry|0.7.4| |EditorConfig|EditorConfig|0.15.1| |github-sharp-theme|joaomoreno|1.4.0| |markdown-all-in-one|yzhang|3.2.0| |remote-containers|ms-vscode-remote|0.134.0| |remote-ssh|ms-vscode-remote|0.51.0| |remote-ssh-edit|ms-vscode-remote|0.51.0| |remote-wsl|ms-vscode-remote|0.44.4| |rest-client|humao|0.24.1| |vscode-arduino|vsciot-vscode|0.3.1| |vscode-docker|ms-azuretools|1.5.0| |vscode-drawio|hediet|0.7.2| |vscode-remote-extensionpack|ms-vscode-remote|0.20.0| |vscode-xml|redhat|0.13.0| |vscodeintellicode|VisualStudioExptTeam|1.2.10| |vsliveshare|ms-vsliveshare|1.0.2666| |vsliveshare-audio|ms-vsliveshare|0.1.85| |vsliveshare-pack|ms-vsliveshare|0.4.0| |vsonline|ms-vsonline|1.0.2669|;Unrelated note
This is honestly the smoothest "Report an issue" workflow I've ever experienced. Kudos.