Closed dragon-fire-fly closed 1 year ago
Firstly, the model was updated to allow the user field to be blank.
class Project(models.Model):
user = models.ManyToManyField(User, blank=True)
p_language = models.ManyToManyField(ProgrammingLanguage)
title = models.CharField(
help_text="What is the title for your project?", max_length=100
)
description = models.TextField(
help_text="Enter your project description here", blank=True, null=True
)
def __str__(self):
return f"<Project name: {self.title}>"
Next, an if statement was added to check whether there is a user associated with the project. If so, the user is displayed and a link to that user's profile is provided. If not. the user is informed that the selected project does not have an owner.
<div class="row">
<div class="col-md-8 offset-md-3">
<p><strong>Title:</strong> {{ project.title }}</p>
<p><strong>Description:</strong> {{ project.description }}</p>
{% if project.user.values.0.username %}
<p><strong>Project Owner:</strong><a href="{% url 'app_home:profile-detail-view' project.user.values.0.username %}">{{ project.user.values.0.username }}</a></p>
{% else %}
<p><strong>Project Owner:</strong>This project no longer has an owner</p>
{% endif %}
<p><strong>Programming Languages:</strong>
{% for language in project.p_language.values %}
{{ language.language }}
{% endfor %}</p>
</div>
</div>
Describe the bug When users are deleted, their projects still appear on the "project overview" page but a
NoReverseMatch
exception occurs as the project owner cannot be found.To Reproduce Steps to reproduce the behavior:
Expected behavior Projects should still be able to be viewed even if the project owner no longer exists
Screenshots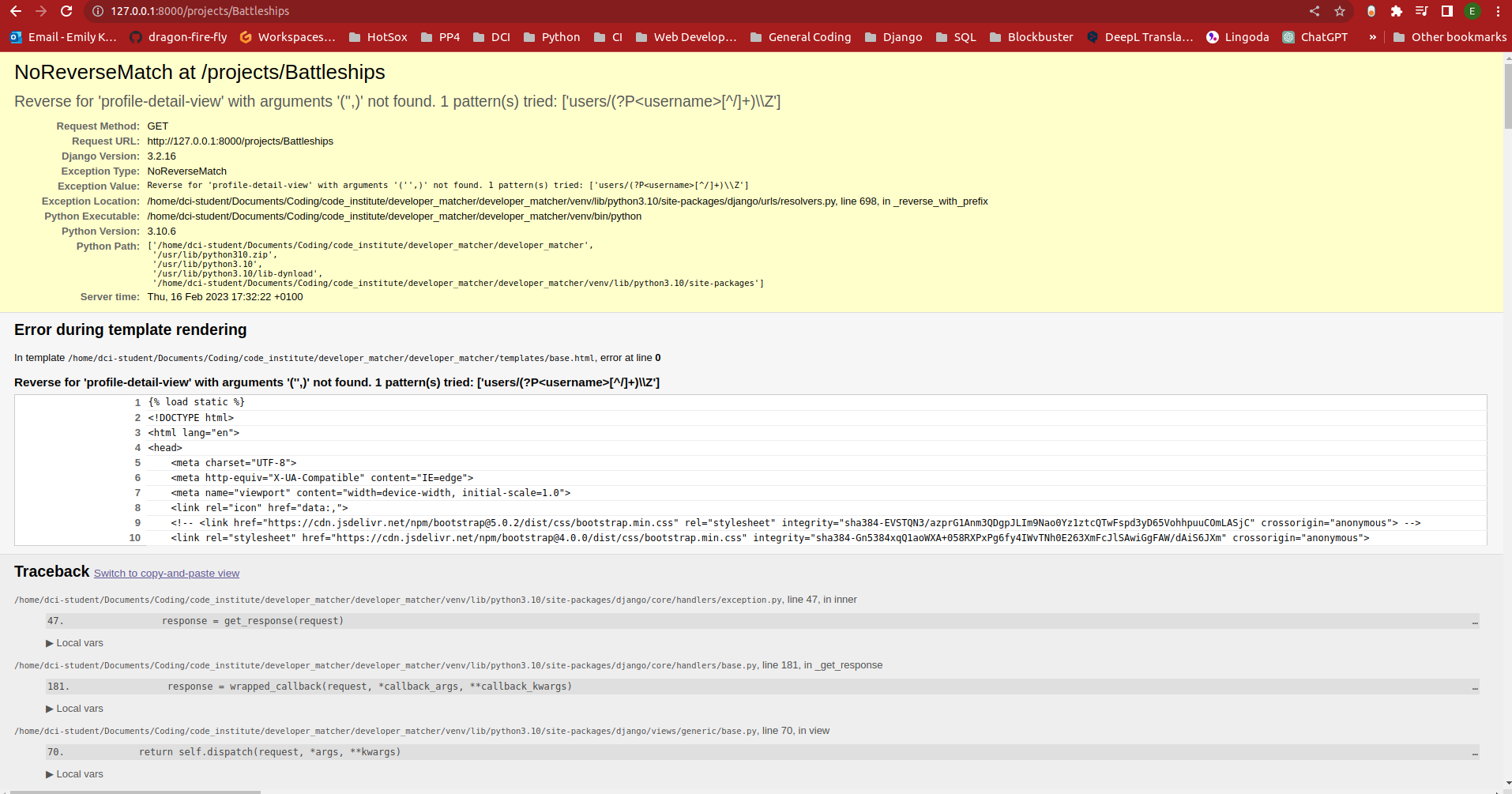
Desktop (please complete the following information):
Additional context The code suspected to be responsible for the reverse lookup error: