Closed dtetreau251 closed 3 years ago
Week 3 Step 8 ⬤⬤⬤⬤⬤⬤⬤⬤◯ | 🕐 Estimated completion: 10-20 minutes
Modify the deepsecrets
Azure Function to
deepsecrets/index.js
to the deepsecrets
branch❗ Make sure to return your message in this format:
Thanks 😊! Stored your secret "insert_user's_message". 😯 Someone confessed that: "a_random_secret"
To test your work, send your Twilio number a text message. You should still receive a text back that contains a message similar to the one below, but now the second secret returned will be random, and no longer the most recent secret stored!
Thanks 😊! Stored your secret "insert_user's_message". 😯 Someone confessed that: "a_random_secret"
💡 Yay! This means you've successfully queried for and returned a random secret.
In your createDocument
function, you'll first want to modify the original SQL query to now query for all secrets inside your container.
Next, you'll want to select a random secret by:
Math.floor()
and Math.random()
):bulb: Make sure to change your responseMessage to return the correct index of items
.
Please complete after you've viewed the Week 3 livestream! If you haven't yet watched it but want to move on, just close this issue and come back to it later.
Help us improve BitCamp Serverless - thank you for your feedback! Here are some questions you may want to answer:
How was the content? Good! Did it help you? Yes! It was great learning about databases. Was it too challenging or too easy? Just right. Did it help you complete the week's homework? Yes. How was the pace? Was it hard to follow along? No. Just verbiage changes needed at times in the instructions to make things more clear. Did we go too slow? No. If you could add/improve something to/in the livestream, what would it be? Refine instructions
What was confusing about this week? If you could change or add something to this week, what would you do? Your feedback is valued and appreciated!
What was confusing about this week? ENDPOINT and KEY should be COSMOS_ENDPOINT and COSMOS_KEY in instructions If you could change or add something to this week, what would you do? Refine verbiage.
Go ahead and merge this branch to main
to move on. Great work finishing this section!
⚠️ If you receive a
Conflicts
error, simply pressResolve conflicts
and you should be good to merge!
Week 3 Step 7 ⬤⬤⬤⬤⬤⬤⬤◯◯ | 🕐 Estimated completion: 20-30 minutes
Leaky secrets
✅ Task:
deepsecrets/index.js
to thedeepsecrets
branch❗ Make sure to return your message in this format:
🚧 Test Your Work
To test your work, send your Twilio number a text message. Once you do so, if you navigate to your Cosmos database account, go to Data Explorer, and the dropdowns underneath your new
SecretStorer
database, you should see an item that contains a message with your text message!Important Note: If you are triggering the function for the first time, you may see an error message like the one below:
Don't worry! Try sending another text message, and everything should work fine the second time around. This error occurs because you don't have anything stored in CosmosDB yet.
1. Create your Cosmos Databases
Before we do anything else, we'll want to create our Cosmos DB account.
:question: How do I create my Cosmos DB account?
1. Navigate to your Azure Portal and click on `Azure Cosmos DB` under Azure Services.  2. Click `Create Azure Cosmos DB Account`. 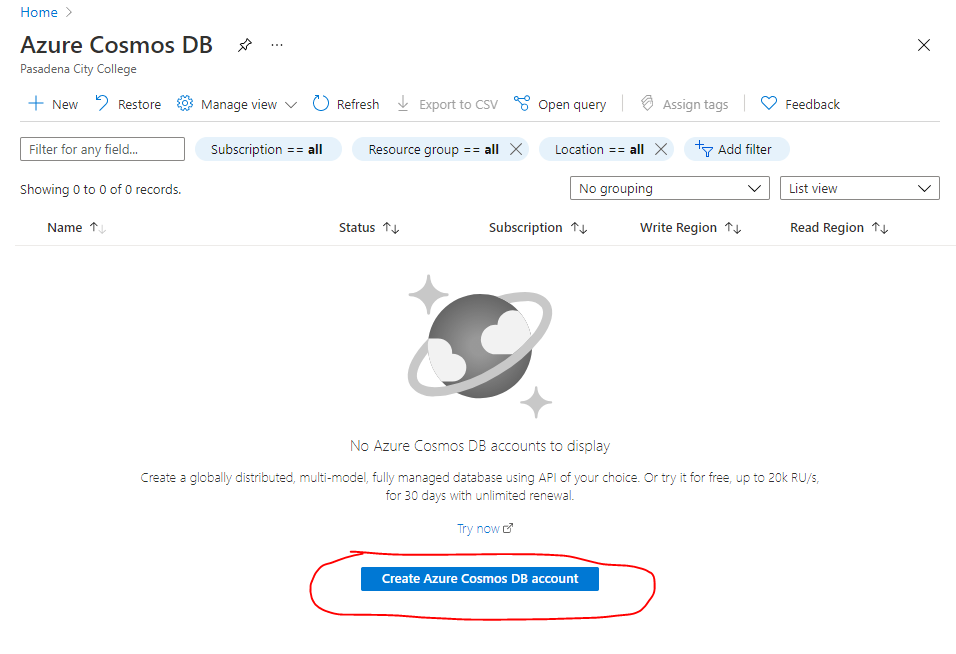 3. Under `Select API Option`, choose `Core (SQL) - Recommended`. 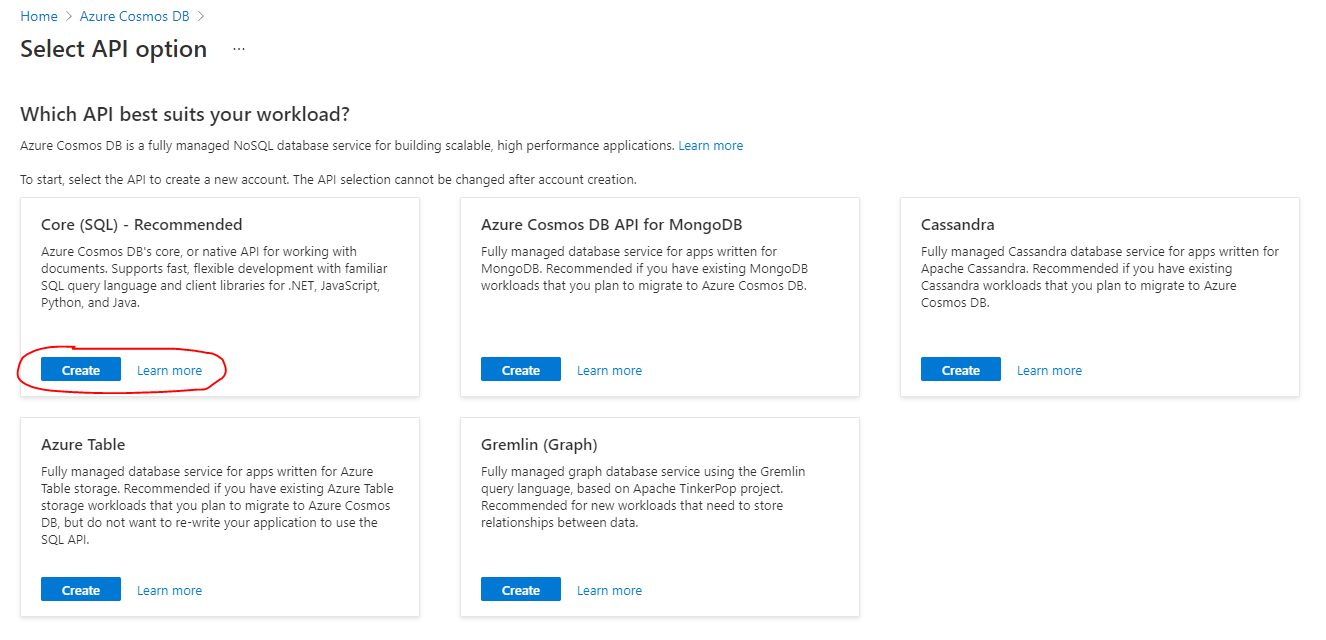 4. Fill in your account name, leave default options as they are, and click `Review + create`. 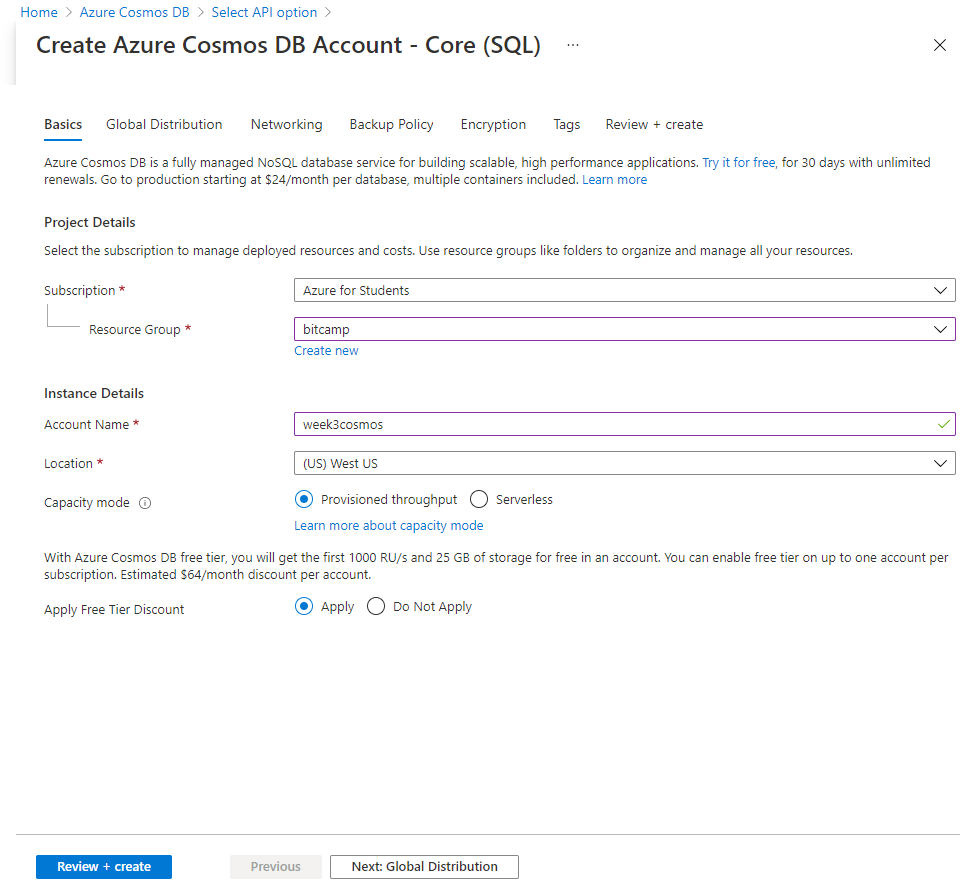 5. Click `Create` a final time. 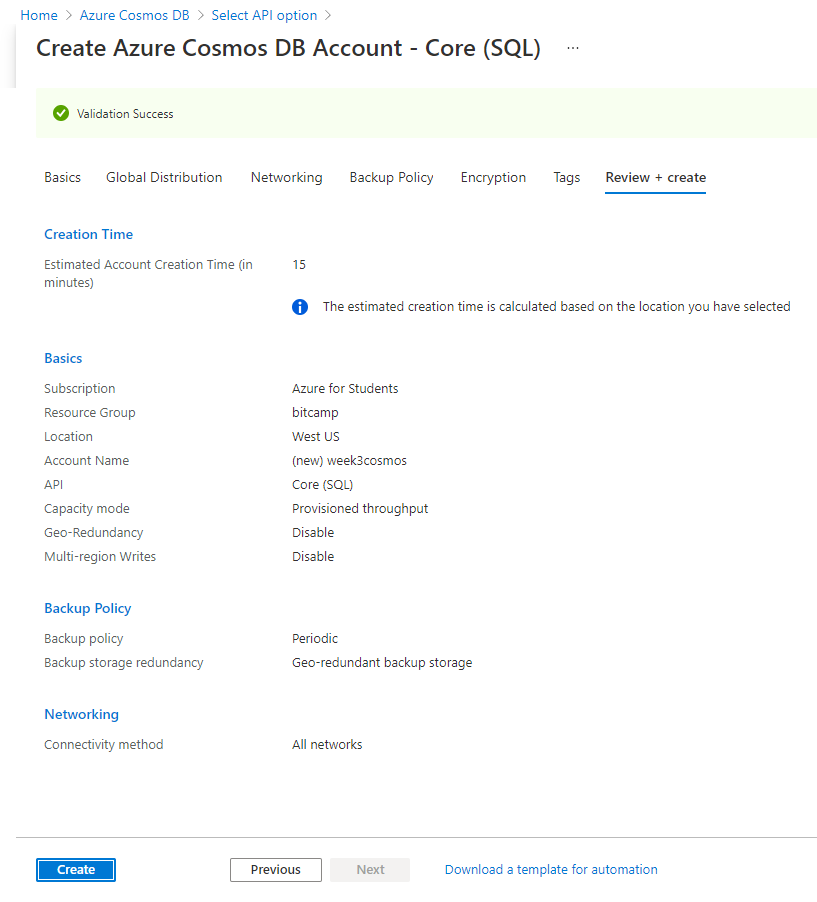 6. Once deployment is complete, click `Go to resource`. 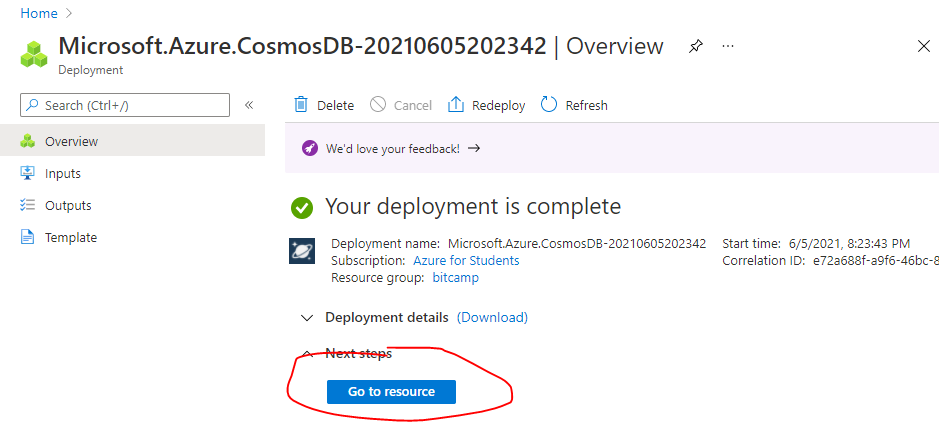1. Install @azure/cosmos
Now that your Azure Cosmos DB account is created, we should install the
npm
package@azure/cosmos
.🔧 1. Configuration
First, we need to instantiate a variable
CosmosClient
from the@azure/cosmos
package we just downloaded.Create a config object that contains all of the sensitive information that we need to manipulate our data.
Call it
config
, and place keys forendpoint
,key
,databaseId
,containerId
, andpartitionKey
.:question: What should my config object look like?
Here is an example of the config object. Make sure your databaseId, containerId, and partitionKey are correct. ```js const config = { endpoint: process.env.ENDPOINT, key: process.env.KEY, databaseId: "SecretStorer", containerId: "secrets", partitionKey: {kind: "Hash", paths: ["/secrets"]} }; ```2. The
create
FunctionNow, we want to write an asynchronous
create
function that takes in the parameter ofclient
(this will be ourCosmosClient
).This function will:
⭐️ Use the client to create a database with an id of config.databaseId if it does not exist.
```js const { database } = await client.databases.createIfNotExists({ id: config.databaseId }); ```⭐️ Use the client to create a container inside the database of ID config.databaseId. This container will have an ID of config.containerId and a key of config.partitionKey.
```js const { container } = await client .database(config.databaseId) .containers.createIfNotExists( { id: config.containerId, key: config.partitionKey }, { offerThroughput: 400 } ); ```📜 2. The
createDocument
FunctionNext, we need to create another asynchronous function called
createDocument
that will take in a parameter ofnewItem
.In its entirety, the function will:
⭐️ Use the global config object to create the database and container if they do not exist. (Hint: this uses the create function we just implemented!)
```js var { endpoint, key, databaseId, containerId } = config; const client = new CosmosClient({endpoint, key}); const database = client.database(databaseId); const container = database.container(containerId); await create(client, databaseId, containerId); ```⭐️ Create and execute a query that uses SQL language to fetch the most recent data document.
This SQL query requests for the "top 1" document when it is orderd by `c._ts`, or the timestamp, in descending order. ```js const querySpec = { query: "SELECT top 1 * FROM c order by c._ts desc" }; ``` Using the query `querySpec`, it will use the `container` we created to fetch the most recent document! ```js const { resources: items } = await container.items.query(querySpec).fetchAll(); ```⭐️ Create an document for your database that contains the information passed through the parameter `newItem`.
```js const {resource: createdItem} = await container.items.create(newItem); ```Finally, it should return your
items
object from the function which contains the most recent message.3. The Main Function
Instead of directly passing
queryObject.Body
(the SMS message) tocontext.res
:message
.createDocument
function you just coded to get youritems
object.:question: How do I create a new document with queryObject.Body?
```js const queryObject = querystring.parse(req.body); let message = queryObject.Body; let document = {"message" : message} let items = await createDocument(document) ```Your response message can then look something like: