Closed tmarenko closed 2 years ago
Thank you @tmarenko . I went down a rabbit hole of trying to upgrade to the latest python, nox, then upgraded packages and back to where I started. So I haven't gotten a chance to take a look at this. I'm back to an operational state and will give this a go.
Sorry that I didn't provide this earlier. In this archive you will find unittests for core functions and UI elements: https://drive.google.com/file/d/1Gg8NP5AQwvTlJSA5-a4FOeCfVU2LzPZh/view?usp=sharing I didn't store this in main repo because screenshots had private account information. Extract archive's content into the project's folder and run test either through Pycharm: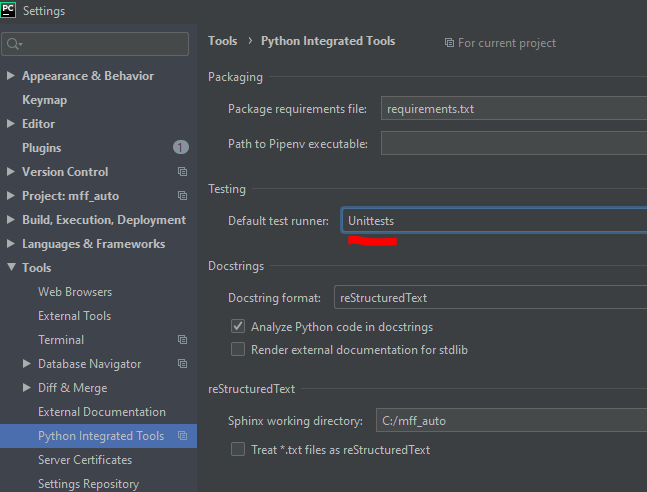
or run
run_tests.py
If you run tests fromrun_test.py
look atresult.log
in the same folder.The most valuable test suite is
test_tessract.py
which tries to recognize UI element on different resolutions from screenshotsunittests/test_images/ui_images
folder. I know that some of screenshot are outdated by now. You can delete them from the folder and recapture again. To capture a propper screenshot update this methods invideo_capture.py
:and run game mode with required UI with enabled video capture. As you can see:
this lines make sure that image would be stored only if UI was recognized and there is already no screenshot in the folder. This method helps to recapture multiple UI elements at one run but you can write your own function if you want to capture just one element from current screen:
I used
emulator._get_screen().copy().save
because the screenshot would be the same structure as the bot sees the screen. Also all of the screenshots were saved in 1080p with HIGH quality graphics. It helps to tune threshold parameter for every resolution.If you have any question about test cases and their logic feel free to ask.