Describe the bug
The attached code should generate 16 small arcs around a circle (every 1024 ez-degrees). However some positions (angles are not rendered, namely: 2048, 4096+2048, 2^13-2048, 2^13+2048)
To Reproduce
Run the attached code on a 5035.
Expected behavior
All arcs should be rendered.
Screenshots
Note image colors are incorrect due to issue #79
MANDATORY: Attachments
----------------------------------------------------------------------
-- ezLCD Meter
--
-- Exampel Code for testing AMeter Widget
--
-- Created 05/02/2023 - Jacob Christ
----------------------------------------------------------------------
gAppName = "EarthLCD Meter Demo Application"
----------------------------------------------------------------------
-- Serial Port Debug Code
----------------------------------------------------------------------
debug_port = 0
-- Event Handelers
-- Serial Port Event
function DebugPortReceiveFunction(byte)
ez.SerialTx(byte, 1, debug_port)
end
function DebugSetup(applicatin_name)
-- open the RS-232 port
ez.SerialOpen("DebugPortReceiveFunction", debug_port)
ez.SerialTx("**********************************************************************\r\n", 80, debug_port)
ez.SerialTx("* " .. applicatin_name .. "\r\n", 80, debug_port)
ez.SerialTx("**********************************************************************\r\n", 80, debug_port)
ez.SerialTx(ez.FirmVer .. "\r\n", 80, debug_port)
ez.SerialTx(ez.LuaVer .. "\r\n", 80, debug_port)
ez.SerialTx("S/N: " .. ez.SerialNo .. "\r\n", 80, debug_port)
ez.SerialTx(ez.Width .. "x" .. ez.Height .. "\r\n", 80, debug_port)
ez.SerialTx("Frames: " .. ez.NoOfFrames .. "\r\n", 80, debug_port)
end
----------------------------------------------------------------------
-- Button Processing Code
----------------------------------------------------------------------
-- Define the Button Event Handler
function ProcessButtons(id, event)
-- TODO: Insert your button processing code here
-- Display the image which corresponds to the event
if id == 0 and event == 1 then
screen_change = true
screen = id
if screen > screen_max then
screen = 0
end
str = "screen=" .. tostring(screen)
ez.SerialTx(str .. "\r\n", 80, debug_port)
end
ez.Button(id, event)
str = "id=" .. tostring(id) .. ", event=" .. tostring(event)
ez.SerialTx(str .. "\r\n", 80, debug_port)
end
function ButtonSetup()
-- Setup button(s)
ez.Button(0, 1, -1, -11, -1, 0, 0, 50, 50) -- Screen capture
-- ez.Button(2, 1, -1, -11, -1, 0, 0, 50, 40) -- Menu
-- ez.Button(3, 1, -1, -11, -1, 0, 80, 320, 150) -- Plot Area
-- Start to receive button events
ez.SetButtonEvent("ProcessButtons")
end
-----------------------------------------------------------------------------
-- LCD printBox
-----------------------------------------------------------------------------
-- x1, y1: Upper left location of printBox
--
-- x2: Upper right location of printBox
--
-- fg: Foreground color
--
-- bg: Background Color
--
-- font_height: Height of box in pixes (actual font height is 70% of this number)
function printBox(x1, y1, x2, fg, bg, font_height, str)
-- Erase Old Weight
local y2 = y1 + font_height
ez.BoxFill(x1,y1, x2,y2, bg) -- X, Y, Width, Height, Color
-- Display Line
ez.SetColor(fg)
ez.SetFtFont(fn, math.floor(font_height * 0.70)) -- Font Number, Height, Width
ez.SetXY(x1, y1)
print(str)
end
fn = 14
font_height = 240 / 8 -- = 30
screen = -1
screen_max = 2
screen_change = true
-----------------------------------------------------------------------------
-- Main
-----------------------------------------------------------------------------
function mainFunction()
DebugSetup(gAppName)
ButtonSetup()
ez.Cls(ez.RGB(0,0,0))
printBox( 0, 0, 160, ez.RGB(0xff, 0xff, 0xff), ez.RGB(0x00, 0x00, 0x80),font_height, "Hello")
printBox(160, 0, 320, ez.RGB(0xff, 0xff, 0xff), ez.RGB(0x80, 0x00, 0x00),font_height, "World")
local x = 160
local y = 120
local r = 70
ez.SetPenSize(10, 10)
ez.CircleFill(x, y, r, ez.RGB(0x80, 0x00, 0x80))
ez.SetColor(ez.RGB(0x00, 0x80, 0x00))
ez.AMeter(x, y, r, 0, 8192)
ez.SetColor(ez.RGB(0x00, 0x80, 0x80))
ez.AMeter(x, y, r, 4096, 8192+4096)
ez.SetColor(ez.RGB(0xff, 0xff, 0xff))
local theta_start = 0
local theta_stop = 16384
local theta_step = (theta_stop - theta_start) / 16
local tick_width = 1
for theta = theta_start, theta_stop, theta_step
do
str = string.format("%0.1f", theta)
printBox(0, 1 * font_height, 100, ez.RGB(0xff, 0xff, 0xff), ez.RGB(0x80, 0x80, 0x00), font_height, str)
if theta + tick_width <= 16384 then
ez.Arc(x, y, r, math.floor(theta), math.floor(theta) + tick_width)
ez.Wait_ms(500) -- Wait 5 seconds
end
end
ez.Wait_ms(1000) -- Wait 5 seconds
-- ez.SdScreenCapture()
-- ez.SetPinOut(100)
while 1 do
if screen_change == true then
if screen == 0 then
ez.SdScreenCapture()
end
screen_change = false
end
end
end
function errorHandler(errmsg)
local str
font_height = 240 / 16 -- = 60
str = debug.traceback()
printBox(0, 8 * font_height, 320, ez.RGB(0xff, 0xff, 0xff), ez.RGB(0x80, 0x00, 0x80), font_height, str)
ez.SerialTx(str .. "\r\n", 80, debug_port)
str = errmsg
printBox(0, 0 * font_height, 320, ez.RGB(0xff, 0xff, 0xff), ez.RGB(0x80, 0x00, 0x00), font_height, str)
ez.SerialTx(str .. "\r\n", 80, debug_port)
end
-- errorHandler function demo
-- illegal command ez.SetPinOut(100) will print out to ezLCD Display
-- Note: using this command will turn off the regular error messages to the Lua console
-- Call mainFunction() protected by errorHandler
rc, err = xpcall(function() mainFunction() end, errorHandler)
Describe the bug The attached code should generate 16 small arcs around a circle (every 1024 ez-degrees). However some positions (angles are not rendered, namely: 2048, 4096+2048, 2^13-2048, 2^13+2048)
To Reproduce Run the attached code on a 5035.
Expected behavior All arcs should be rendered.
Screenshots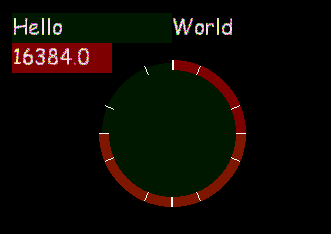
MANDATORY: Attachments