Open tmssngr opened 1 year ago
This is the screenshot when using FillLayout instead.
If using FillLayout and invoking table.computeSize(SWT.DEFAULT, SWT.DEFAULT);
before showing the shell, the same behavior as with GridLayout
occurs.
I see this on both X11 and Wayland backends.
Here is a simpler reproducer:
public class TableWithoutHeader {
public static void main(String[] args) {
final Display display = new Display();
final Shell shell = new Shell(display);
shell.setLayout(new GridLayout(1, false));
final Table table = new Table(shell, SWT.VIRTUAL | SWT.FULL_SELECTION | SWT.H_SCROLL | SWT.V_SCROLL);
table.setLayoutData(new GridData(SWT.FILL, SWT.FILL, true, true));
// XXX This is the tell-tale line
table.setHeaderVisible(false);
final TableColumn column = new TableColumn(table, SWT.NONE);
column.setText("Hello");
column.setResizable(false);
column.setWidth(100);
table.setItemCount(50);
shell.setSize(400, 500);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
}
The bug is that the scrollbar always leaves room for the header even when column headers are set to non-visible. If you change table.setHeaderVisible(false);
above to true
then you can see the header fits exactly into the space left by the scrollbar.
Potential workaround: Using TableColumn
is not mandatory -- if you don't need column headers you can add TableItems
directly. See the SWT Snippet 35 for an example of this: https://github.com/eclipse-platform/eclipse.platform.swt/blob/master/examples/org.eclipse.swt.snippets/src/org/eclipse/swt/snippets/Snippet35.java
It looks like a table.computeSize(SWT.DEFAULT, SWT.DEFAULT);
before the table.setHeaderVisible(false);
eliminates this misbehavior for me.
Describe the bug The attached snippet places a single-column table inside a shell and used GridLayout for that. When moving the mouse over the table, it becomes noticeable, that the vertical scrollbar is not on the top though Item 0 is shown. Using FillLayout instead of the GridLayout and this effect does not occur. Most likely the table behaves differently if its preferred size has been requested before by the GridLayout.
To Reproduce
Expected behavior The vertical scrollbar should be at the top under all conditions when showing the first item.
Screenshots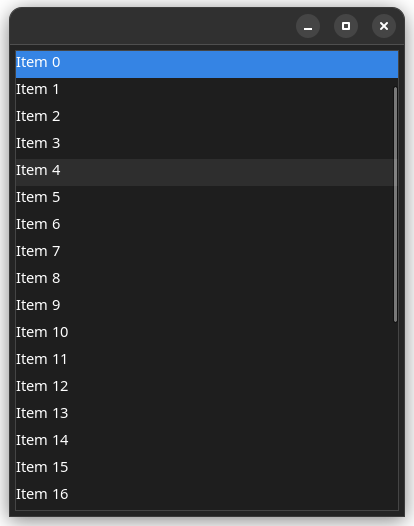
Environment:
Select the platform(s) on which the behavior is seen:
Additional OS info (e.g. OS version, Linux Desktop, etc) Reproduced with latest Manjaro with Gnome 3.24.34, default Adw-dark theme.
JRE/JDK version Java 17
Version since I have not bisected.
Workaround (or) Additional context None.