Open diskra opened 1 year ago
same problem, any progress please ?
@diskra Thanks for the issue, and my apologies for the delay looking at this.
Can you (or @farzin-khaleghian) help me reproduce here? When I attempt to run your code:
npx tsc index.ts
node dist/index.js
I get this error:
Error: When a manual configuration is not provided, gateway requires an Apollo configuration. See https://www.apollographql.com/docs/apollo-server/federation/managed-federation/ for more information. Manual configuration options include: `serviceList`, `supergraphSdl`, and `experimental_updateServiceDefinitions`.
at ApolloGateway.load (/Users/trentm/el/apm-agent-nodejs10/tmp/issue3245/node_modules/@apollo/gateway/dist/index.js:264:23)
at SchemaManager.start (/Users/trentm/el/apm-agent-nodejs10/tmp/issue3245/node_modules/apollo-server-core/dist/utils/schemaManager.js:42:65)
at ApolloServer._start (/Users/trentm/el/apm-agent-nodejs10/tmp/issue3245/node_modules/apollo-server-core/dist/ApolloServer.js:195:50)
at ApolloServer.start (/Users/trentm/el/apm-agent-nodejs10/tmp/issue3245/node_modules/apollo-server-core/dist/ApolloServer.js:180:27)
at startGateway (/Users/trentm/el/apm-agent-nodejs10/tmp/issue3245/dist/index.js:81:18)
at startApolloServer (/Users/trentm/el/apm-agent-nodejs10/tmp/issue3245/dist/index.js:89:12)
at Object.<anonymous> (/Users/trentm/el/apm-agent-nodejs10/tmp/issue3245/dist/index.js:91:1)
at Module._compile (node:internal/modules/cjs/loader:1196:14)
at Object.Module._extensions..js (node:internal/modules/cjs/loader:1250:10)
at Module.load (node:internal/modules/cjs/loader:1074:32)
at Function.Module._load (node:internal/modules/cjs/loader:909:12)
at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12)
at node:internal/main/run_main_module:22:47
Or I could run with NODE_ENV=local
if I had a "./supergraph.graphql" file. I stopped in the Apollo supergraph docs quickstart before installing the rover
tool or creating an Apollo account.
I'm still looking at your debug log. It looks like the transaction.name was set properly in the GraphQL instrumentation, and then later when the transaction was ended that was overwritten with the POST /graphql
express-route-based info. I think this may require a change to the APM agent to deal with prioritization of setting the transaction.name when multiple instrumentations are in play (in this case 'graphql' and 'express').
@trentm My bad for not including the supergraph schema. I will post a sample below, however, not sure if it would actually work without the service linked actually running. With all that said, this PR that you merged in recently is actually the end goal https://github.com/elastic/apm-agent-nodejs/pull/3203 and as soon as it's available on npm, I will immediately give it a shot and see if everything is working as expected and if it is, this issue would be irrelevant for me/us personally. With alll that said, here is the supergraph
schema
@link(url: "https://specs.apollo.dev/link/v1.0")
@link(url: "https://specs.apollo.dev/join/v0.3", for: EXECUTION)
{
query: Query
}
directive @join__enumValue(graph: join__Graph!) repeatable on ENUM_VALUE
directive @join__field(graph: join__Graph, requires: join__FieldSet, provides: join__FieldSet, type: String, external: Boolean, override: String, usedOverridden: Boolean) repeatable on FIELD_DEFINITION | INPUT_FIELD_DEFINITION
directive @join__graph(name: String!, url: String!) on ENUM_VALUE
directive @join__implements(graph: join__Graph!, interface: String!) repeatable on OBJECT | INTERFACE
directive @join__type(graph: join__Graph!, key: join__FieldSet, extension: Boolean! = false, resolvable: Boolean! = true, isInterfaceObject: Boolean! = false) repeatable on OBJECT | INTERFACE | UNION | ENUM | INPUT_OBJECT | SCALAR
directive @join__unionMember(graph: join__Graph!, member: String!) repeatable on UNION
directive @link(url: String, as: String, for: link__Purpose, import: [link__Import]) repeatable on SCHEMA
scalar join__FieldSet
enum join__Graph {
BACKOFFICE_SUBGRAPH @join__graph(name: "backoffice-subgraph", url: "http://backoffice-subgraph:3000/graphql")
}
scalar link__Import
enum link__Purpose {
"""
`SECURITY` features provide metadata necessary to securely resolve fields.
"""
SECURITY
"""
`EXECUTION` features provide metadata necessary for operation execution.
"""
EXECUTION
}
type Query
@join__type(graph: BACKOFFICE_SUBGRAPH)
{
_backoffice: Boolean
}
Thanks. As you suspected, I can get very far with a local repro because I don't have a http://backoffice-subgraph:3000/graphql
running.
Looking again at the debug log you posted, I misinterpreted it earlier. I think I see why the original transaction is not getting a graphql-y name.
First, let's consider a single GraphQL service -- i.e. not one using Apollo Gateway. When you call it via curl -X POST http://localhost:3000/graphql?...
what happens is:
graphql.execute(...)
to make a GraphQL call. At this step the APM agent's http
instrumentation creates a unnamed Transaction
for the HTTP request, and its apollo-server-core
instrumentation sets a boolean on that transaction say "this request is for graphql-related stuff, but I'll wait for the graphql
instrumentation to set a good transaction name from the parsed query.graphql.execute(...)
call is made. The APM agent's graphql
instrumentation creates a GraphQL Span
named GraphQL: ...
, and it uses the parsed query info to set a transaction name.Now take the Apollo Gateway case:
graph-gateway-1
in your log), receives the HTTP request. The APM agent's http
instrumentation creates an unnamed Transaction
, and its apollo-server-core
instrumentation sets the boolean marker.buyer-subgraph:3000
, which eventually returns.POST /graphql
.The difference here is that the APM agent in the graph-gateway-1
service has no instrumentation that handles the parsed graphql query. graphql.execute(...)
is not used in this service, so currently the APM agent doesn't have anything looking at a parsed GraphQL query to use to set a GraphQL-y transaction.name.
To support this I think we'd need the APM agent to instrument the appropriate part of @apollo/gateway
to have parsed query information to use to set a good transaction.name. If you have suggests as to the inner workings of @apollo/gateway
that would help. The thing that would most help would be a small repro case (in a small github repository or where ever) that showed a small Apollo Gateway service and a downstream Apollo Server app that it calls out to.
@trentm Thank you for that detailed explanation, I certainly learned a lot from it. Unfortunately, I don't know much about the inner workings of Apollo's core. I just setup a very stripped down dockerized setup for you to play with, if you wish to do so, https://github.com/diskra/trent-dejan
I just setup a very stripped down dockerized setup for you to play with
Thanks!
So far, I get some errors. First in yarn
:
error Couldn't find package "@rockethomes/rh-digital-commons@1.7.0" required by "@eapi/graphql-helper@1.0.0" on the "npm" registry.
in yarn run tsbuild
:
$ tsc --build --clean && tsc --build
libraries/graphql-helper/src/custom-scalar.ts:1:20 - error TS2307: Cannot find module 'moment' or its corresponding type declarations.
1 import moment from "moment";
~~~~~~~~
libraries/graphql-helper/src/graph.ts:9:33 - error TS2307: Cannot find module 'graphql-middleware' or its corresponding type declarations.
9 import { applyMiddleware } from "graphql-middleware";
~~~~~~~~~~~~~~~~~~~~
libraries/graphql-helper/src/graph.ts:10:37 - error TS2307: Cannot find module '@apollo/subgraph' or its corresponding type declarations.
10 import { buildSubgraphSchema } from "@apollo/subgraph";
~~~~~~~~~~~~~~~~~~
Found 3 errors.
error Command failed with exit code 1.
and I'll have to install rover
.
Full log here: https://gist.github.com/trentm/9824fb78b0278413cda48d77420acf27
Ideas?
@trentm very sorry about that. I just pushed up a few commits that remove the @rockethomes/rh-digital-commons@1.7.0 package, as well as remove the need for rover installation. Pull down the latest and let me know if you run into any more issues.
@trentm tested out this just now https://github.com/elastic/apm-agent-nodejs/pull/3336 and apollo server 4 works as expected, without a gateway. With a gateway, the gateway shows is transaction type "request" instead of graphql but when the gateway sends the request down the chain, to the other services (apollo servers), it shows as graphql type.
Does not work
const server = new ApolloServer({
gateway,
introspection: true,
plugins: getPlugins(true),
includeStacktraceInErrorResponses: process.env.NODE_ENV !== "prod",
});
Works
const server = new ApolloServer({
schema: shieldedSchema,
plugins: getPlugins(),
includeStacktraceInErrorResponses: process.env.NODE_ENV !== "prod",
});
package.json
"@apollo/gateway": "^2.3.3",
"@apollo/subgraph": "^2.3.3",
"@apollo/server": "^4.4.0",
"elastic-apm-node": "^3.46.0"
Here are the debug logs.
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.281Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"intercepted request event call to http.Server.prototype.emit for /graphql"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.282Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start trace {\"trans\":\"1d74fa67b3eace88\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"unnamed\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.282Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(1d74fa, 'unnamed')> )","ecs":{"version":"1.6.0"},"message":"supersedeWithTransRunContext(<Trans 1d74fa67b3eace88>)"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.286Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start span {\"span\":\"f84a72e128bdc513\",\"parent\":\"1d74fa67b3eace88\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"unnamed\",\"type\":\"external\",\"subtype\":\"http\",\"action\":null}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.287Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"intercepted call to http.request {\"id\":\"1d74fa67b3eace88\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.287Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"request details: {\"protocol\":\"http:\",\"host\":\"buyer-subgraph:3000\",\"id\":\"1d74fa67b3eace88\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.293Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"intercepted request event call to http.Server.prototype.emit for /graphql"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.296Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"continue trace {\"trans\":\"e3926bbdc5dc1a61\",\"parent\":\"f84a72e128bdc513\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"unnamed\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.296Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'unnamed')> )","ecs":{"version":"1.6.0"},"message":"supersedeWithTransRunContext(<Trans e3926bbdc5dc1a61>)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.301Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"intercepted call to graphql.execute"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.301Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start span {\"span\":\"884f32ce212d09ba\",\"parent\":\"e3926bbdc5dc1a61\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"GraphQL: Unknown Query\",\"type\":\"db\",\"subtype\":\"graphql\",\"action\":\"execute\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.302Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"setting default transaction name: ZipCode__buyer_subgraph__0 zipCode (/graphql) {\"trans\":\"e3926bbdc5dc1a61\",\"parent\":\"f84a72e128bdc513\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.302Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start span {\"span\":\"824c2d5014385942\",\"parent\":\"884f32ce212d09ba\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE Query.zipCode\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.303Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode')]> )","ecs":{"version":"1.6.0"},"message":"supersedeWithSpanRunContext(<Span 824c2d5014385942>)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.303Z","log":{"logger":"elastic-apm-node"},"method":"POST","path":"/local-location/_search","ecs":{"version":"1.6.0"},"message":"intercepted call to @elastic/elasticsearch.Transport.prototype.request"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.304Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start span {\"span\":\"541b6ad6c16c885b\",\"parent\":\"824c2d5014385942\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"Elasticsearch: POST /local-location/_search\",\"type\":\"db\",\"subtype\":\"elasticsearch\",\"action\":\"request\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.304Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"intercepted call to https.request {\"id\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.425Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"ended span {\"span\":\"541b6ad6c16c885b\",\"parent\":\"824c2d5014385942\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"Elasticsearch: POST /local-location/_search\",\"type\":\"db\",\"subtype\":\"elasticsearch\",\"action\":\"request\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.425Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode')]> )","ecs":{"version":"1.6.0"},"message":"addEndedSpan(Elasticsearch: POST /local-location/_search)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.425Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"encoding span {\"span\":\"541b6ad6c16c885b\",\"parent\":\"824c2d5014385942\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"Elasticsearch: POST /local-location/_search\",\"type\":\"db\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.426Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"ended span {\"span\":\"824c2d5014385942\",\"parent\":\"884f32ce212d09ba\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE Query.zipCode\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.426Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode')]> )","ecs":{"version":"1.6.0"},"message":"addEndedSpan(RESOLVE Query.zipCode)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.426Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"encoding span {\"span\":\"824c2d5014385942\",\"parent\":\"884f32ce212d09ba\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE Query.zipCode\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.427Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start span {\"span\":\"d517817294c9e20a\",\"parent\":\"824c2d5014385942\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.id\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.427Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode', ended),Span(d51781, 'RESOLVE ZipCode.id')]> )","ecs":{"version":"1.6.0"},"message":"supersedeWithSpanRunContext(<Span d517817294c9e20a>)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.427Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start span {\"span\":\"b1653a3148159b2d\",\"parent\":\"d517817294c9e20a\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.name\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.427Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode', ended),Span(d51781, 'RESOLVE ZipCode.id'),Span(b1653a, 'RESOLVE ZipCode.name')]> )","ecs":{"version":"1.6.0"},"message":"supersedeWithSpanRunContext(<Span b1653a3148159b2d>)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.427Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start span {\"span\":\"858495df2341dca9\",\"parent\":\"b1653a3148159b2d\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.webUrl\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.427Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode', ended),Span(d51781, 'RESOLVE ZipCode.id'),Span(b1653a, 'RESOLVE ZipCode.name'),Span(858495, 'RESOLVE ZipCode.webUrl')]> )","ecs":{"version":"1.6.0"},"message":"supersedeWithSpanRunContext(<Span 858495df2341dca9>)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.428Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"start span {\"span\":\"7e67142623d5c54f\",\"parent\":\"858495df2341dca9\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.webName\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.428Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode', ended),Span(d51781, 'RESOLVE ZipCode.id'),Span(b1653a, 'RESOLVE ZipCode.name'),Span(858495, 'RESOLVE ZipCode.webUrl'),Span(7e6714, 'RESOLVE ZipCode.webName')]> )","ecs":{"version":"1.6.0"},"message":"supersedeWithSpanRunContext(<Span 7e67142623d5c54f>)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.428Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"ended span {\"span\":\"d517817294c9e20a\",\"parent\":\"824c2d5014385942\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.id\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.428Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode', ended)]> )","ecs":{"version":"1.6.0"},"message":"addEndedSpan(RESOLVE ZipCode.id)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.428Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"encoding span {\"span\":\"d517817294c9e20a\",\"parent\":\"824c2d5014385942\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.id\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.429Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"ended span {\"span\":\"b1653a3148159b2d\",\"parent\":\"d517817294c9e20a\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.name\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.429Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode', ended),Span(d51781, 'RESOLVE ZipCode.id', ended)]> )","ecs":{"version":"1.6.0"},"message":"addEndedSpan(RESOLVE ZipCode.name)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.429Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"encoding span {\"span\":\"b1653a3148159b2d\",\"parent\":\"d517817294c9e20a\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.name\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.429Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"ended span {\"span\":\"858495df2341dca9\",\"parent\":\"b1653a3148159b2d\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.webUrl\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.429Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode', ended),Span(d51781, 'RESOLVE ZipCode.id', ended),Span(b1653a, 'RESOLVE ZipCode.name', ended)]> )","ecs":{"version":"1.6.0"},"message":"addEndedSpan(RESOLVE ZipCode.webUrl)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.429Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"encoding span {\"span\":\"858495df2341dca9\",\"parent\":\"b1653a3148159b2d\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.webUrl\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.430Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"ended span {\"span\":\"7e67142623d5c54f\",\"parent\":\"858495df2341dca9\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.webName\",\"type\":\"custom\",\"subtype\":null,\"action\":null}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.430Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)'), [Span(884f32, 'GraphQL: ZipCode__buyer_subgraph__0 zipCode'),Span(824c2d, 'RESOLVE Query.zipCode', ended),Span(d51781, 'RESOLVE ZipCode.id', ended),Span(b1653a, 'RESOLVE ZipCode.name', ended),Span(858495, 'RESOLVE ZipCode.webUrl', ended)]> )","ecs":{"version":"1.6.0"},"message":"addEndedSpan(RESOLVE ZipCode.webName)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.430Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"encoding span {\"span\":\"7e67142623d5c54f\",\"parent\":\"858495df2341dca9\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.webName\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.432Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"ended span {\"span\":\"884f32ce212d09ba\",\"parent\":\"e3926bbdc5dc1a61\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"GraphQL: ZipCode__buyer_subgraph__0 zipCode\",\"type\":\"db\",\"subtype\":\"graphql\",\"action\":\"execute\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.432Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(e3926b, 'ZipCode__buyer_subgraph__0 zipCode (/graphql)')> )","ecs":{"version":"1.6.0"},"message":"addEndedSpan(GraphQL: ZipCode__buyer_subgraph__0 zipCode)"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.433Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"encoding span {\"span\":\"884f32ce212d09ba\",\"parent\":\"e3926bbdc5dc1a61\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"GraphQL: ZipCode__buyer_subgraph__0 zipCode\",\"type\":\"db\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.434Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"setting transaction result {\"trans\":\"e3926bbdc5dc1a61\",\"parent\":\"f84a72e128bdc513\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"result\":\"HTTP 2xx\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.434Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending span {\"span\":\"541b6ad6c16c885b\",\"parent\":\"824c2d5014385942\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"Elasticsearch: POST /local-location/_search\",\"type\":\"db\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.435Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending span {\"span\":\"824c2d5014385942\",\"parent\":\"884f32ce212d09ba\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE Query.zipCode\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.435Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending span {\"span\":\"d517817294c9e20a\",\"parent\":\"824c2d5014385942\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.id\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.435Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending span {\"span\":\"b1653a3148159b2d\",\"parent\":\"d517817294c9e20a\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.name\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.435Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending span {\"span\":\"858495df2341dca9\",\"parent\":\"b1653a3148159b2d\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.webUrl\",\"type\":\"custom\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.435Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"intercepted http.ClientRequest response event {\"id\":\"1d74fa67b3eace88\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.435Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending span {\"span\":\"7e67142623d5c54f\",\"parent\":\"858495df2341dca9\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"RESOLVE ZipCode.webName\",\"type\":\"custom\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.435Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending span {\"span\":\"884f32ce212d09ba\",\"parent\":\"e3926bbdc5dc1a61\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"GraphQL: ZipCode__buyer_subgraph__0 zipCode\",\"type\":\"db\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.436Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"intercepted http.IncomingMessage end event {\"id\":\"1d74fa67b3eace88\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.436Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"ended span {\"span\":\"f84a72e128bdc513\",\"parent\":\"1d74fa67b3eace88\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"POST buyer-subgraph:3000\",\"type\":\"external\",\"subtype\":\"http\",\"action\":\"POST\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.436Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<Transaction(1d74fa, 'POST unknown route (unnamed)')> )","ecs":{"version":"1.6.0"},"message":"addEndedSpan(POST buyer-subgraph:3000)"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.437Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"encoding span {\"span\":\"f84a72e128bdc513\",\"parent\":\"1d74fa67b3eace88\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"POST buyer-subgraph:3000\",\"type\":\"external\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.437Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending span {\"span\":\"f84a72e128bdc513\",\"parent\":\"1d74fa67b3eace88\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"name\":\"POST buyer-subgraph:3000\",\"type\":\"external\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.436Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<> )","ecs":{"version":"1.6.0"},"message":"addEndedTransaction(ZipCode__buyer_subgraph__0 zipCode (/graphql))"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.437Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending transaction {\"trans\":\"e3926bbdc5dc1a61\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\"}"}
eapi-buyer-subgraph-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.437Z","log":{"logger":"elastic-apm-node"},"trans":"e3926bbdc5dc1a61","name":"ZipCode__buyer_subgraph__0 zipCode (/graphql)","parent":"f84a72e128bdc513","trace":"d454cb70374972d40ea22f98d767cdca","type":"graphql","result":"HTTP 2xx","duration":140.661,"ecs":{"version":"1.6.0"},"message":"ended transaction"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.439Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"setting transaction result {\"trans\":\"1d74fa67b3eace88\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\",\"result\":\"HTTP 2xx\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.440Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"setting default transaction name: POST /graphql {\"trans\":\"1d74fa67b3eace88\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.440Z","log":{"logger":"elastic-apm-node"},"ctxmgr":"AsyncLocalStorageRunContextManager( RunContext<> )","ecs":{"version":"1.6.0"},"message":"addEndedTransaction(POST /graphql)"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.441Z","log":{"logger":"elastic-apm-node"},"ecs":{"version":"1.6.0"},"message":"sending transaction {\"trans\":\"1d74fa67b3eace88\",\"trace\":\"d454cb70374972d40ea22f98d767cdca\"}"}
eapi-graph-gateway-1 | {"log.level":"debug","@timestamp":"2023-05-31T17:25:53.441Z","log":{"logger":"elastic-apm-node"},"trans":"1d74fa67b3eace88","name":"POST /graphql","trace":"d454cb70374972d40ea22f98d767cdca","type":"request","result":"HTTP 2xx","duration":158.37,"ecs":{"version":"1.6.0"},"message":"ended transaction"}
With a gateway, the gateway shows is transaction type "request" instead of graphql but when the gateway sends the request down the chain, to the other services (apollo servers), it shows as graphql type.
@diskra Yes, that fits my understanding. If we want the incoming requests to the Apollo Gateway-using server to be marked as GraphQL requests, then we'll need to add new instrumentation of the @apollo/gateway
library.
Sorry that I haven't had a chance to get back to this one yet.
@trentm all good, we are all busy. I get it :)
I won't be able to learn @apollo/gateway
and dig into this for this milestone.
Hello ! Same issue here, any work arround while this is being fixed ?
Describe the bug
I have a graphql app setup using Apollo Gateway and apollo server express. The transactions get reported to APM but they get reported as normal http /graphql transaction and not as graphQL transaction type in the dropdown.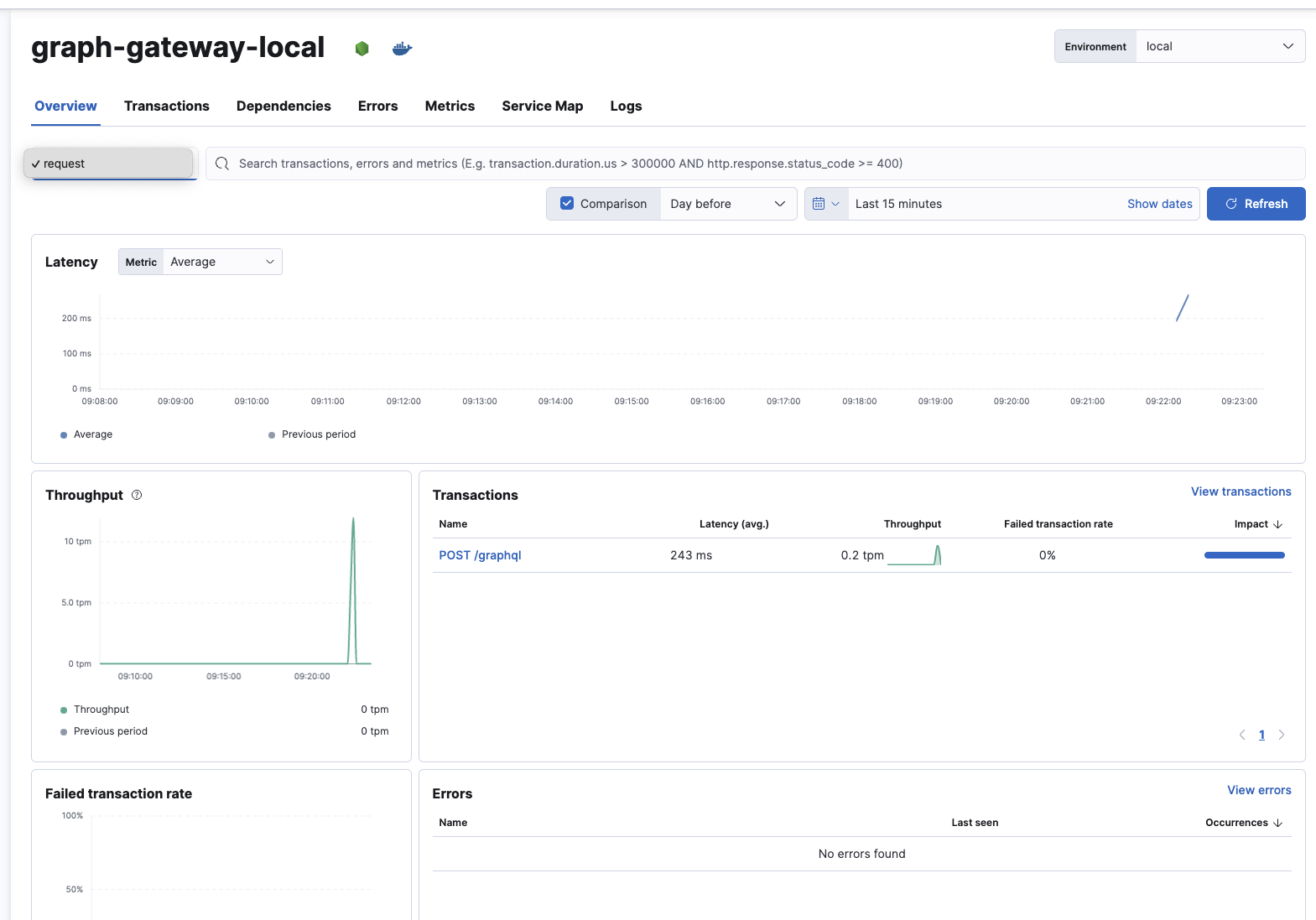
Environment (please complete the following information)
package.json
index.ts
Debug Logs