Closed Vensim closed 3 years ago
Model output fixed upon inspection of Issue 17's comments.
Replace
c_code = port(model, pretty_print=True)
with
c_code = port(model, pretty_print=True, optimize=False)
Sent request.
@eloquentarduino I suggest changing the code in the original file. It seems lots of people ask the same question including me at the beginning. Thanks.
@eloquentarduino Is this the same issue as was posted here?
I see on updated example of Sine , optimize=False
is missing, did adding it fix it for you?
Publishing EloquentTinyML==2.4.4 with optimize=False
in every Python example. I will migrate the tinymlgen.port
function into my new everywhereml
package.
The output of the serial comms is.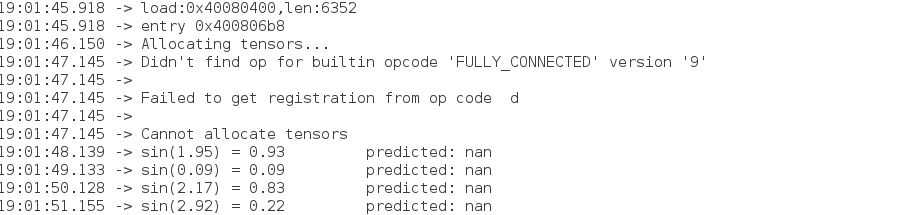
What I've tried : Increasing and decreasing Tensor arena size, still same error.
Haven't done much changes to the model generation other than outputting straight to a c file :
Here are my files and the model that's being used. Python script :
Arduino IDE code :
sine_model.h