Open SonyStone opened 2 years ago
I've started working on a TypeScript flavor of ganja.js
. There's no documentation yet, but I hope the code is slightly more readable:
https://github.com/frostburn/ts-geometric-algebra
I agree. The examples in CoffeeShop are incomprehensible and unintuitive to me. It doesn't look like code at all. I'm not even referring to operator overloading, but the formatting, naming and other conventions. I'm going to give an example.
For example, to take a line from the 3D PGA Slicing sample:
var facedata = faces.map(x=>{ var f; return [f=x[0]&x[1]&x[2],(f<<(x[0]&x[1])),(f<<(x[1]&x[2])),(f<<(x[2]&x[0]))]});
I have no idea who is the target audience of this code. Is it for newcomers? There are lots of unfamiliar operators with no spacing at all between them.
Let's add some line-breaks:
var facedata = faces.map(x => {
var f;
return [
f = x[0] & x[1] & x[2],
(f << (x[0] & x[1])),
(f << (x[1] & x[2])),
(f << (x[2] & x[0]))
];
});
I'm still not sure this does what I think it does. There is an undefined f
that is only defined within the return statement. What does the assignment statement return? It's not a common pattern in JS code, certainly not the most obvious. Why not define it on the same line with the declaration with modern syntax, i.e. const f = x[0] & x[1] & x[2]
?
And what does f
even stand for? Or x
for that matter? f
sounds like it could be a face, but maybe x
is the face since it is the parameter of map over faces (?)
And what about this line?
var contour=[], minicontour=[]; faces.forEach((x,i)=>{
Never have I seen a forEach
starting from the same line with var
(a var with multiple definitions no less).
var [f,e1,e2,e3]=facedata[i], l=(f^cut),
p1=(l^e1),p2=(l^e2),p3=(l^e3),
p12=(p1&e2).s>=0,p13=(p1&e3).s>=0,p23=(p2&e3).s<0, res=[];
Again so much happening within just one var-statement. Destructuring, variable definitions with short names and with very little spacing between. Why not instead:
p12
etc.The project is cool, but there's no way I can use it when I have such a difficult time reading the example code
I like to refer to it as GanjaScript, because obviously we do AST manipulation for operator overloading and scientific notation overloading etc.
Once you do AST manipulation and change semantics of different stuff I have a hard time to still call it JavaScript - it's GanjaScript.
The operator overloading in itself is a hacky quick solution, so it may or may not work (I opened issues and PR's for that).
It often caused me lots of wasted time to work with GanjaScript, so I just decided to remove it entirely - no ambiguity, no stupid lexer/parser issues because you added one space too much somewhere, clear method calls without remembering an operator-to-method table at the expense of a few more characters. Good deal for me.
The code you refer to is mostly just the "boring" stuff, like who cares about 3D OBJ model parser loading code, you find that in dozens of engines. You could write it over 20 lines, but then it would just make the examples longer and longer without adding too much meaning.
But since we use ESM, we could of course just make use of import
and have a util library that is readable and it's still just one line of code (importing a function).
But then again, this repo hasn't been to active lately. So fork it and improve what you want for yourself - it's a great exercise to warm up to GA in itself. And also learn amazing JavaScript tricks you may find nowhere else. Steven knows what he is doing and it's all free after all.
Sorry if my message came up too aggressive
The code you refer to is mostly just the "boring" stuff, like who cares about 3D OBJ model parser loading code,
My complaints do not refer to the OBJ parsing at all. I avoided commenting that for the reasons you just mentioned. Instead I referred to the interesting code, such as that facedata
and then the code inside the graph.
So fork it and improve what you want for yourself - it's a great exercise to warm up to GA in itself.
That's what I've started doing actually. I started reading and cleaning that slicing example, and trying to explain all the operations with pen and tablet. It's very helpful.
It's not just this site though. This same example was used in the SIGGRAPH 2019 talk GA for Computer Graphics. The snippet there looked like this (almost exactly the same as :
var contour=[];
faces.forEach((x,i)=>{
var [f,e1,e2,e3]=facedata[i], res=[];
l=(f^cut), p1=(l^e1), p2=(l^e2), p3=(l^e3),
p12=(p1&e2).s>=0,
p13=(p1&e3).s>=0,
p23=(p2&e3).s<0
if (p1.e123 && (p12 == p13)) res.push(p1);
if (p2.e123 && (p12 == p23)) res.push(p2);
if (p3.e123 && (p13 != p23)) res.push(p3);
if (res.length==2) contour.push(res)
})
The slide had a lot of space to spare (both vertically and horizontally), so I can't fathom why the code was written this tersely. Maybe it wasn't targeted for programmers? It took me a while to realize that in this case e1, e2 and e3 are edges and not elements of the basis.
Code golf hits programmers and mathematicians alike :sweat_smile:
Hello, I hope I'm not being rude, but I'm trying to get into geometric algebra to try to use it in computer graphics/game engine. But after studying, when it all comes to code, articles and lectures refer to this library. And this library source code is extremely incomprehensible, with all its operator overloads and math like code. Are there any simple examples in the form of simple functions of these operators, so I can understand what is going on in them in general and add it to my own code?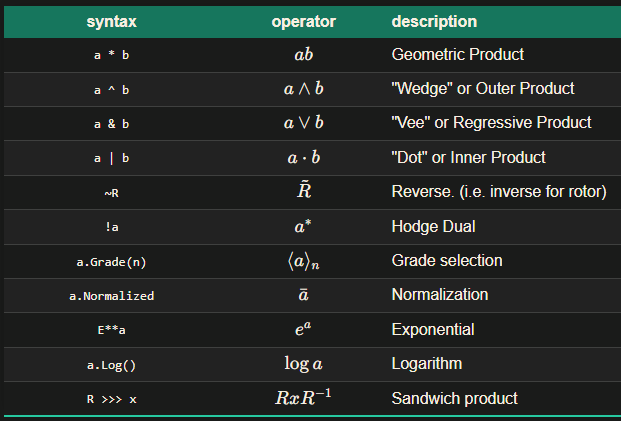