Closed calebmsword closed 3 years ago
Welp, I found my mistake.
The issue was finding the button before the state changed (I assign button
in the callback sent to beforeEach). If I simply query for the button after invoking the textChanges, then the mocked function is called with the new state:
const id = 'id';
const name = 'name';
it('inputing text in boxes and pressing button should have desired side effects', () => {
id_input.invoke('onChangeText')(id);
name_input.invoke('onChangeText')(name);
button = wrapper
.find(Text)
.findWhere( node =>
node.text().toLowerCase().includes('add')
&& node.text().toLowerCase().includes('client')
&& ( typeof node.prop('onPress') !== 'undefined' )
)
.last(); // finding button here instead of in beforeEach is the secret sauce
button.invoke('onPress')();
expect(mockAddNewClient).toHaveBeenCalledWith(id, name);
})
This change makes the test pass :)
EDIT: It is clear after solving this problem that the state of the component was, in fact, updating, but that I accidentally performed assertions on a ReactWrapper that I did not invoke any updates to.
Current behavior
I have a React Native functional component that renders two
TextInput
components. EachTextInput
has anonChangeText
prop whose listener calls the setter returned from theuseState
hook to change one of two variables. However, when trying to run unit tests on this component, invokingonChangeText
never results in any state change.The component:
Essentially, the component has two text boxes whose values determine the state (the name and id of a new "Client" object that the component allows the user to create). The component also renders a button that, when pressed, calls a handler that takes two arguments, which are the two state variables of the component. A realistic application of this component would make an API call to create a new Client in the Client database.
The test:
Result of test
Actual Component behavior
Typing two words in the text boxes and pressing the "button" logs the expected string to console.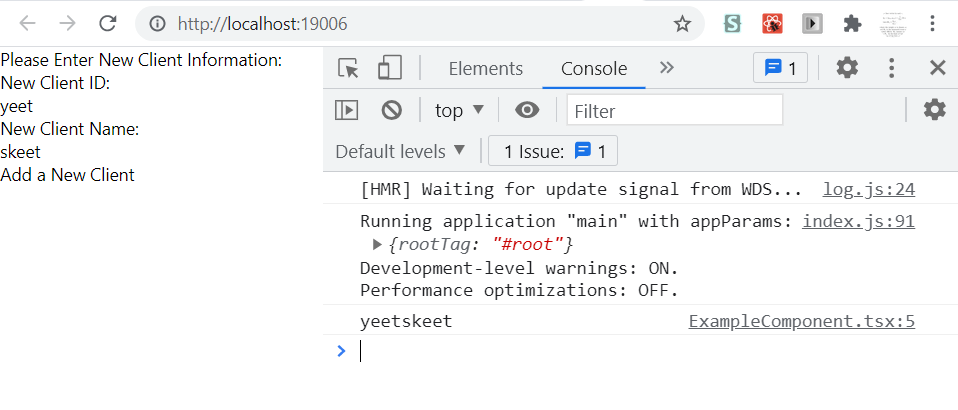
Expected behavior
Invoking
onChangeText
(or simulating changeText) on aTextInput
rendered in a mounted functional React Native component should result in state change; i.e., in the test shown above, we expectmockAddNewClient
to have been called with"yeet"
and"skeet"
instead of the initial values of the component state.Your environment
The application was built with React Native in a project initialized with Expo in a managed workflow. My tests are run using git bash on Windows 10.
You can access a repository that demonstrates this issue here. Feel free to clone the project if you wish.
I am currently mounting the component. I have also tried using
shallow
but it does not work either. If I have to use a method other thaninvoke
to make this work, that is fine--all I care about is being able to test state changes caused by theonTextChange
handler in a functional React Native component. Any suggestions would be appreciated.API
Version
Adapter