Open weizzh opened 2 years ago
hello, sorry I can't reproduce your abnormal HANDSHAKE signals by your code. but I found something doubtable:
CS
line, so if transmit just time out on slave, the post trans callbk will not be called, then the HANDSHEKE
line will always high and have no positive edge to trig msp430 start an transaction. if you controll the handshake io manually, it still run after timeout.data rady
io on msp430 with a low level, it may cheat slave a ready signal at the boot of msp430, however the msp430 is not ready to start a transaction, in this case that msp430 cheat with a ready signal but not start the transaction will trig slave into timeout (I reproduced your first issue in that case)spi_slave_transmit
will block, but it will time out at first time in your code because you set positive edge(will trig msp430 start trans) after spi_slave_transmit
, then every time call spi_slave_transmit
you actually get the results before this transaction, so the function return immediately.good luck for you.
Thanks. While awating your response, I found a workaroud to solve these data error: I put ad related io isr and tasks on core 1. I don't know why, bu it worked. Now I'm faced another problem, this ad seemes too "sensitive": I added some print() in related task, then I watched lots of jitter; When other tasks go wrong, for example, sa card write error or wifi instablity, huge jitter appears.
spi_slave_transmit will block, but it will time out at first time in your code because you set positive edge(will trig msp430 start trans) after spi_slave_transmit, then every time call spi_slave_transmit you actually get the results before this transaction, so the function return immediately.
that's to say, though data seems like normal, the spi transactions accually work in error timing sequense.This can cause spi transactions to be affected by something others easily?
Answers checklist.
General issue report
Hi: my sdk:IDF 4.4, my hardware:ESP32-S3
I use esp32-s3 as spi slave to receive data from an msp430 controller. Basically the application is modified according to the spi_slave receiver example code. The msp430 shall produce an pulse(the DATAREADY io, see the pic below) every 3.4ms. After detecting this signal, esp32-s3 will pull down the HANDSHAKE line. The msp430 will start an spi transaction, which length is 196 bytes. Esp32 put these data into bufferes, when one of them is full, the data will be transmitted through wifi.
At first I only got timeout results. Then I tried to controll the handshake io manually(see the code below), and this time I got two timeout errors at the beginning and following transactions are ok. Well now, I find there is still a bug there: very accasionally(about several seconds or more), the several bytes at the beginning of one transation is "overwritten" by the fowllowing transation. With the help of a logic analyzer, I found some abnornal handshape signals that may caused this problem.
This is one abnormal handshake signal, witch last much longer than normal ones.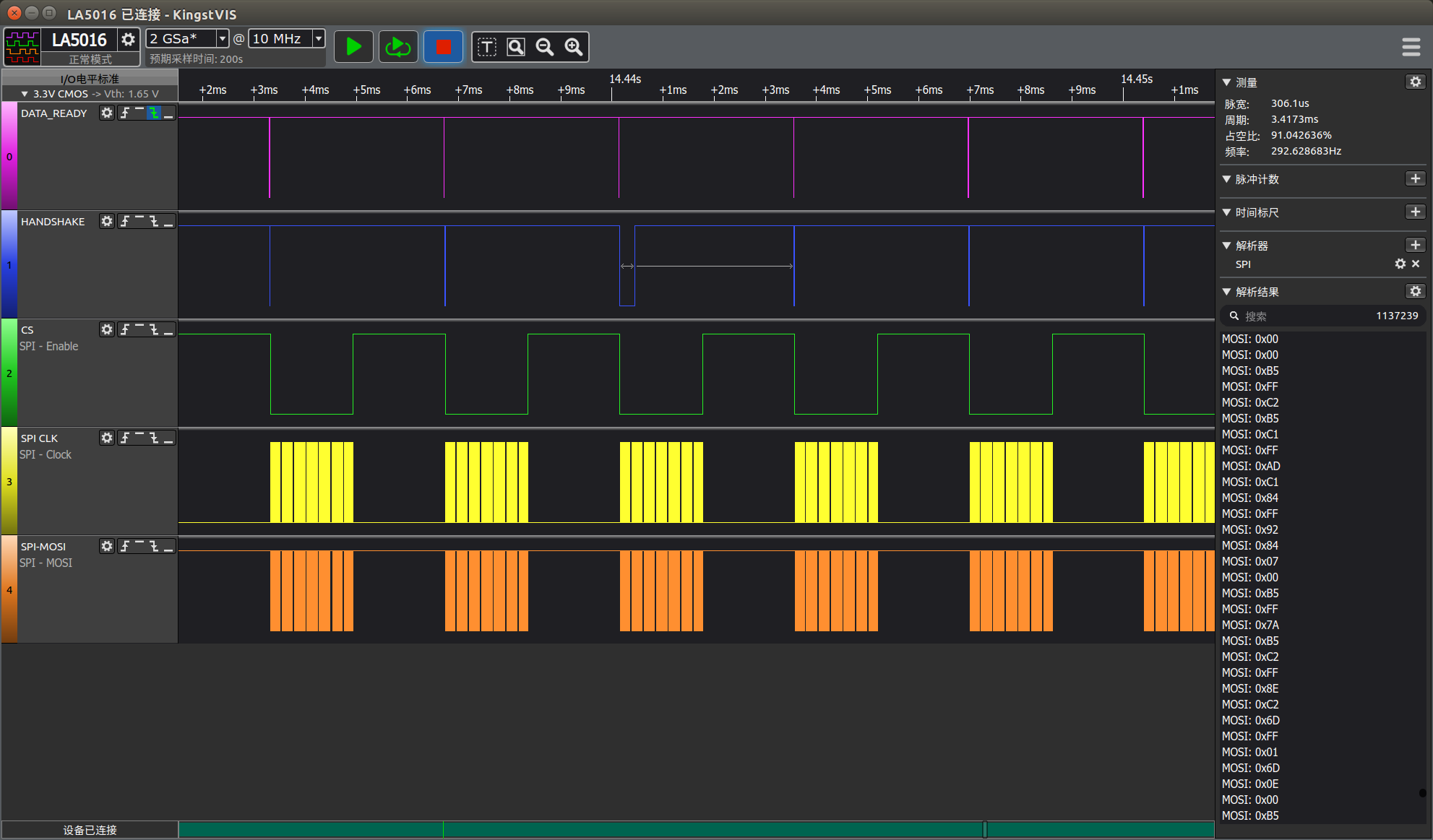
Zoom in these signals: normal signals: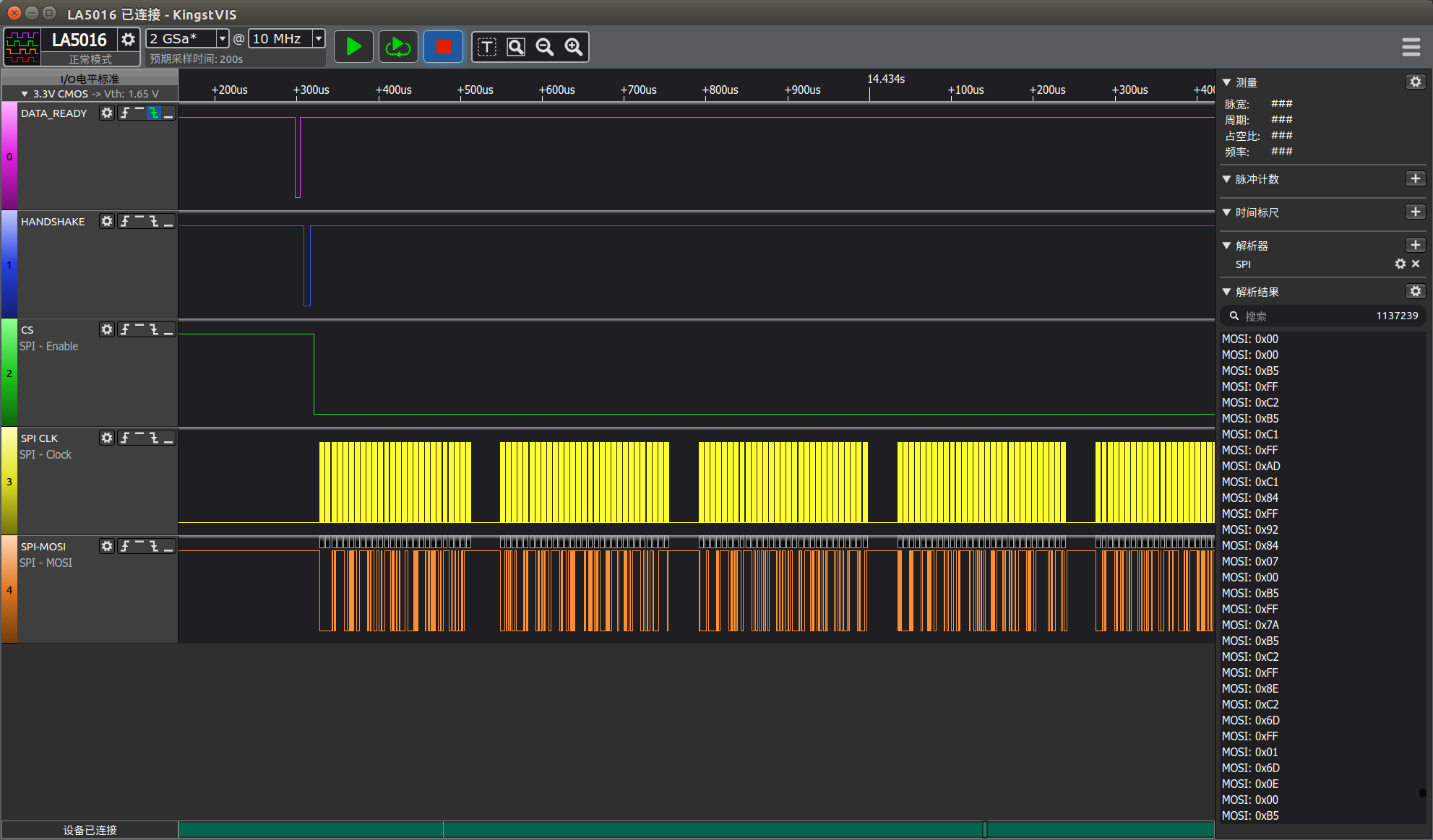
abnormal handshake signals: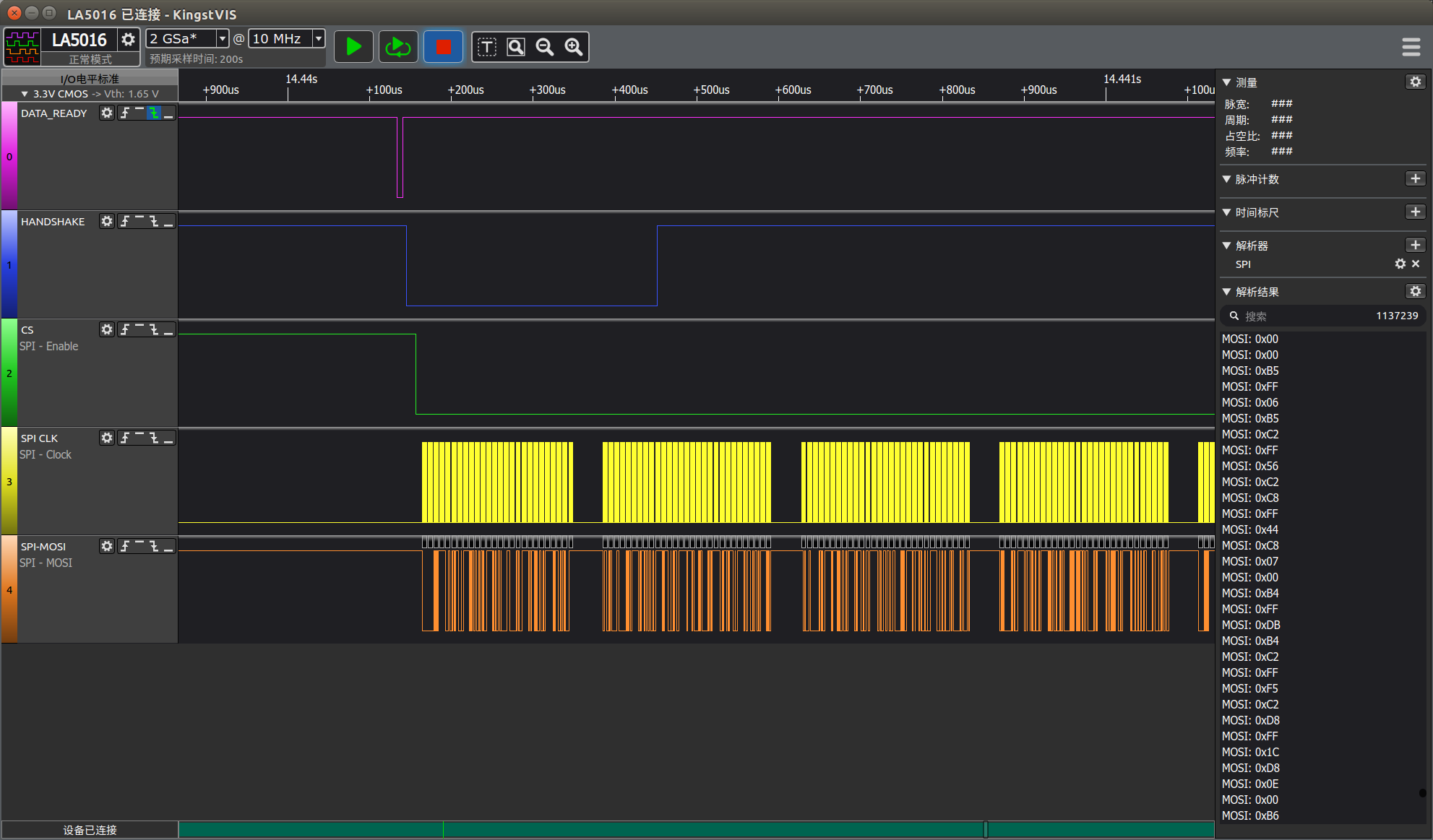
After some calculation, now I'm very sure that when abnormal handshake signales happen, in the handshake "low" duration, those bytes being transed "overwrite" the begining bytes of the former transaction. My code:
some init config:
My questions: 1: can give some hint why setup/post transaction callback functions fail? 2:spi_slave_transmit will block or unblock? The handshake is quicklly pulled up after merely several us, so the func will not unblock? 3: Why sometimes handshake signals last much more time? My guess is the task(I have set the priority of 19) is just pre-empted by something between
4: is this bug related to spi queues? How can I fix this if setup/post callback functions still not work?