Describe the bug
Hello,
I'm trying to use a tooltip I created with react-laag inside a modal created with react-modal.
When I try to do that, with default behaviour, it's not working but instead throwing an Unhandled Runtime Error
Error: react-laag: Could not find a valid reference for the layer element. There might be 2 causes:
- Make sure that the 'ref' is set correctly on the layer element when isOpen: true. Also make sure your component forwards the ref with "forwardRef()".
- Make sure that you are actually rendering the layer when the isOpen prop is set to true
I'm using this tooltip in my web-app in other places, and it works perfectly in all other contexts. This is the first time I want to use it in a modal, and sadly it doesn't work.
To Reproduce
Steps to reproduce the behavior:
my app.ts looks something like that
import React from 'react'
import ReactModal from 'react-modal'
import { ModalProvider } from 'react-modal-hook'
ReactModal.setAppElement('#__next') // we are using next
const App = () => {
return (
<ModalProvider rootComponent={TransitionGroup}>
{children}
</ModalProvider>)
}
Describe the bug Hello, I'm trying to use a tooltip I created with react-laag inside a modal created with react-modal. When I try to do that, with default behaviour, it's not working but instead throwing an Unhandled Runtime Error
I'm using this tooltip in my web-app in other places, and it works perfectly in all other contexts. This is the first time I want to use it in a modal, and sadly it doesn't work.
To Reproduce Steps to reproduce the behavior:
my app.ts looks something like that
I call my modal with a hook:
and the Tooltip component is like this:
My Modal is provided by a hook and react-modal
where Modal is
Ultimately, I can call my tooltip with
The result is great when used in a regular screen: https://user-images.githubusercontent.com/33933332/142455947-bb7fb6a8-f6cc-4a42-b6cb-b858b627d247.mov
But sadly doesn't work when I use it in a modal, I get this screen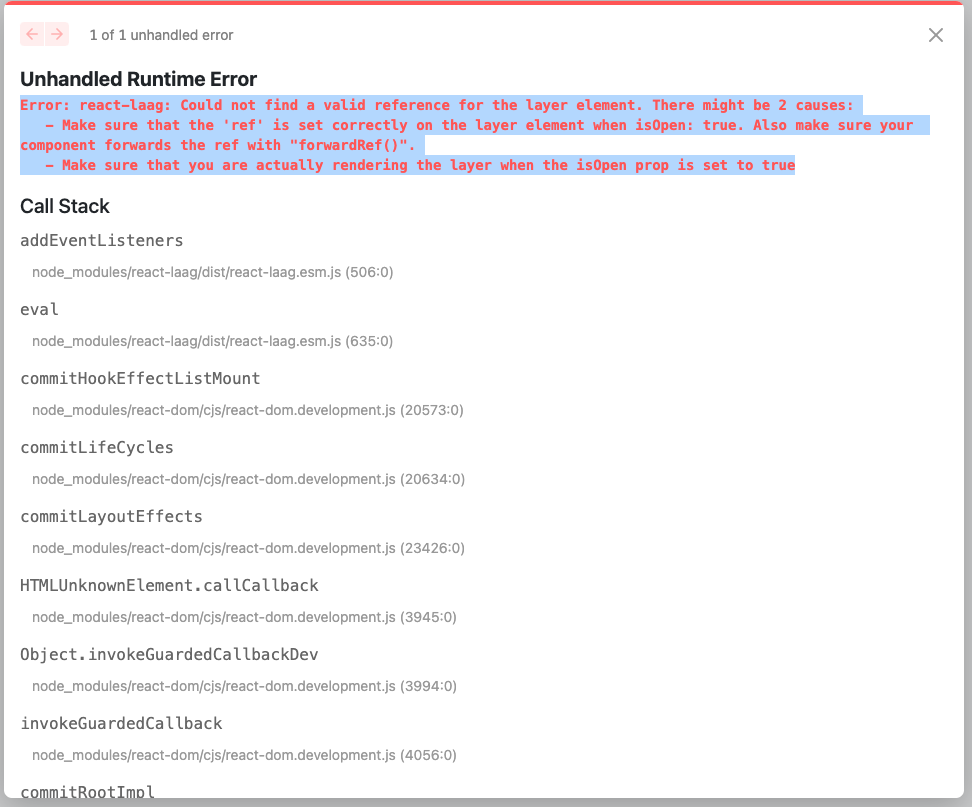
Expected behavior The tooltip is working without breaking when used inside a modal created with react-modal
Browser / OS (please complete the following information):