Closed marrickb closed 1 year ago
Thanks for submitting this issue @marrickb! I will try to find time to look at this asap, but in the meantime could you provide the exact versions of the key packages you're using, namely aeolus
and iris
please?
Hi Denis, thanks for the reply and I am happy to tell you that you've already managed to solve the issue (and sorry for not thinking about this in the first place). I updated iris and aeolus (was still using versions 3.0.2 and 4.13, respectively..) to the latest versions, and was pleased to see the result:
That's fantastic, thanks for the update! Love when issues are solved this easily :smile:
In plotting the transformed wind vectors (not using the divergent component) in tidally-locked coordinates, I can notice some misaligned wind vectors between the two coordinate systems, especially over the gyres. In the first image using geographical coordinates, we can see the gyre structure clearly in the winds. After doing the coordinate transformations and rotating the winds, the second image shows the disappearing of one of the gyre structures. I am currently struggling to find the cause of this. The coordinate transformations rely on the aeolus operations 'rotate_winds_to_tidally_locked_coordinates' and 'regrid_to_tidally_locked_coordinates', and a script to reproduce these plot can be found below. The NetCDF file to test the script can be downloaded from https://github.com/marrickb/netcdf_files.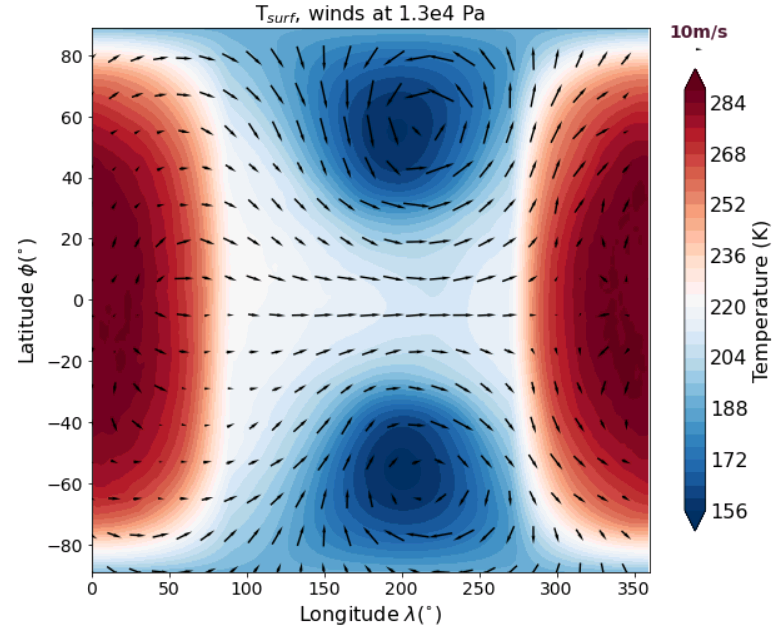