Open banjianpan opened 4 years ago
can you share minimal reproducible code?
Yeah!! heres the minimal that will produce the error above:
import React from 'react';
import { StyleSheet, Text, View, Button } from 'react-native';
import {decode, encode} from 'base-64'
import {firebase} from './firebase-config'
import { Renderer, TextureLoader } from 'expo-three'
if (!global.btoa) { global.btoa = encode }
if (!global.atob) { global.atob = decode }
let counter = 0;
let usercount = -1;
const fb_operations = () =>{
let db = firebase.firestore()
let doc1 = db.collection("count_collection").doc("count_document")
doc1.get()
.then(doc => {
console.log('got document')
if(!doc.exists){
console.log('No such document!');
}else{
console.log("inside else");
usercount = doc.get("count");
console.log(usercount);
usercount++;
console.log(usercount);
doc1.update({
count: usercount
});
}
})
}
export default function App() {
return (
<View style={styles.container}>
<Text>Open up App.js to start working on your app!</Text>
<Button title='increment count' onPress={() => fb_operations()}/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
});
It's weird because although I never even use anything in the expo-three package just importing the package would cause the error after about a minute and 7 seconds. This piece of code basically is updating a field variable in firebase's firestore, so essentially it's a counter app with firestore as the storage. Regardless of importing the package or not, I am still able to update the "count" field in firestore. The main difference now is that when I do import the expo-three package I get the error after a minute and 7 seconds, but without importing the expo-three package, I simply do not get the error. I don't really know which package is creating the error (either the firebase, or expo-three), but I know the combination of the two produces the error above.
Were you guys able to reproduce the error I got above?
Hi, old issue but this problem is still here and I'm facing it 😅
Expo SDK 51 Firebase 11.0.0 expo-three 8.0.0
Any expo-three import anywhere in the code will automaticaly throw the cast error after 1 minutes and 2 seconds for me.
Found a Workaround as I mention in #183 :)
Currently I am able to display a cube on my react native project using this package as well other packages regarding 3d objects. Below the view that displays the cube, I have a button that will update a count in firebase's firestore. However after about a minute and 7 seconds I get this error: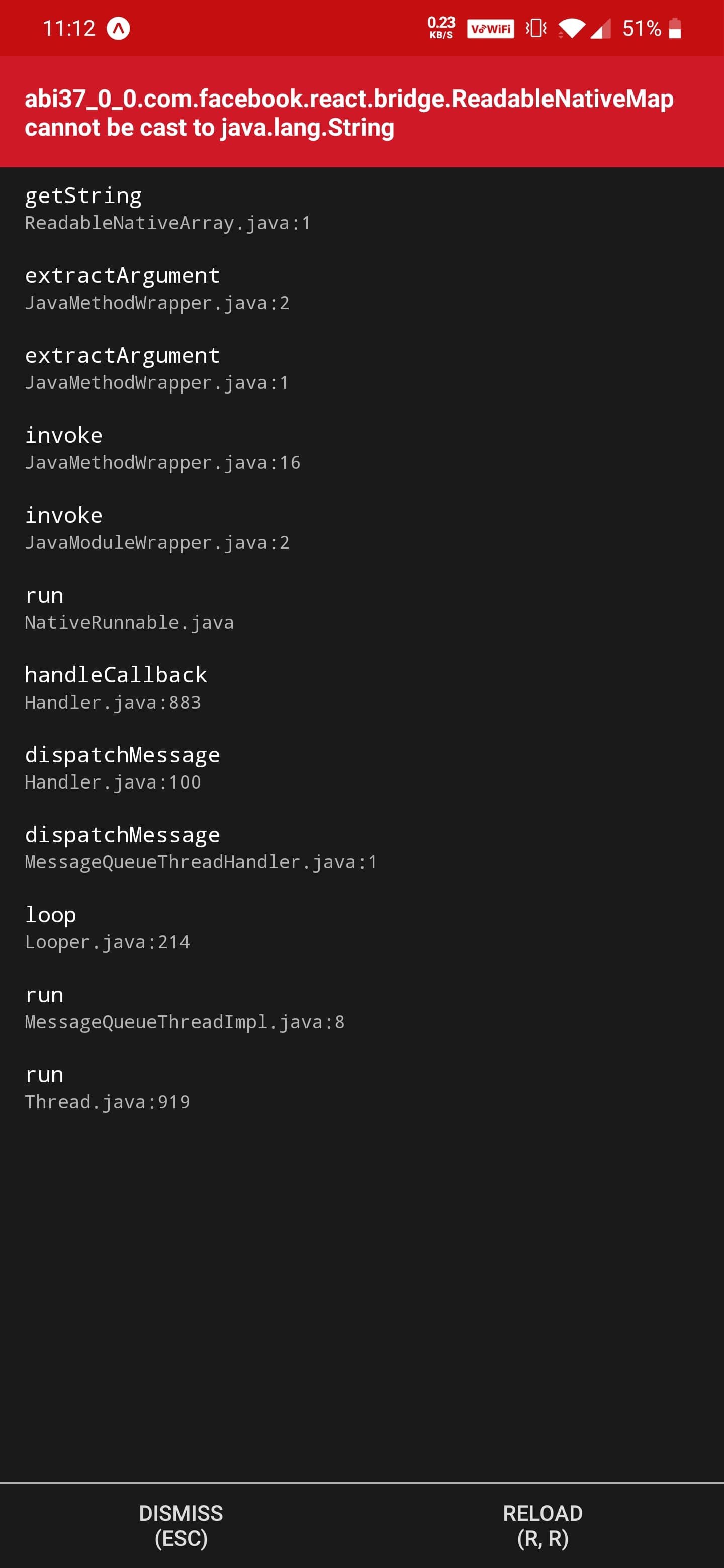
I then tried to isolate the problem and I came to the conclusion that the problem was when I imported this package, expo-three. Without the import of expo-three I am still able to update firestore with no error, but with the import of expo-three, I get the error shown above after about a minute and 7 seconds. However, if I completely get rid of firebase and keep the expo-three package, I do not get the error. So something is causing this error to appear when this package is used in conjunction with the firebase package. Any ideas on how to fix this?