Closed zoukie33 closed 4 years ago
It does indeed look right, I'm sorry that you are having trouble with this.
Could you try and log the name of the unexpected field (this should probably be part of the message btw. happy to take a pull request for that). You can do that by adding this error handler to express and observing the output in the terminal:
app.use(function (err, req, res, next) {
console.log('This is the invalid field ->', err.field)
next(err)
})
hello again, i've done what you told me, and i get: "This is the invalid field -> pic". but the file field in postman is goo and in the code too.
Hmm, interesting 🤔
Could it be that there is more than one muter attached as a middleware somehow? It's hard for me to tell since I can't see the entire code...
That's the only thing I can think of, otherwise, could you try and reduce this to the absolute minimal code that's required to show this behavior and upload somewhere, and then I could take a look at it 👀
Could it be that there is more than one muter attached as a middleware somehow? It's hard for me to tell since I can't see the entire code...
hum i dont really know, but i have jwt protection for this routes, and i have an invalid Access if i dont put this in my app.js :
app.use(upload.array());
app.use(express.static('public'));
app.use('/files', express.static('files'));
I've tried without upload.array(), not working and with .none() the same. this in my app.js can be the problem, but without that i can't access to the route.
for now i just uploaded the 3 files concerned about this problem, if you want i can make you a working version without all my routes (because if you run my api without everything behind this won't work ^^).
here the files https://ufile.io/c3yp2
my app is working on ubuntu server: Ubuntu 18.04.1 LTS
app.use(upload.array());
This line is probably the problem, it shouldn't be there as it will catch all files uploaded to any route and then complain that that they have the wrong field (since none was supplied to that function).
ok, so what can i do to fix that ? cause if i dont put it in my add.js, and post route who need an upload cant be reach if this is not here ... the solution can be do an app.use on multer + the configurations needed (storage etc), but how can i use it next in multiples routes ?
You should be able to remove the app.use(upload.array())
but keep the , upload.single('pic'),
part. Is there anything that doesn't work when you do that?
I've already try it, it i remove the app.use(upload.array()), there is a conflic with my jwt securrity, i have an error 401 (Invalid Access). All the routes who need an auth pass through this function:
var verifyToken=function (req, res,next) {
var token = req.body.token || req.query.token || req.headers['token'];
if (token) {
jwt.verify(token, config.secret, function (err, currUser) {
if (err) {
res.send(err);
} else {
req.currUser = currUser;
next();
}
});
}
else {
res.status(401).send("Invalid Access");
console.log("User rejeté");
}
};
but as you can see, in postman there is the token. did i need to put multer bnefore jwt auth ? It's not very safe in my case ... :/
ah, hmmm, is there a specific reason for providing the token in the body instead of as a header?
Specifically, it's best practice to name the header Authorization
and add the token as Bearer ${token}
I will try, I will come back to you as soon as possible
app.use(upload.array());
This line is probably the problem, it shouldn't be there as it will catch all files uploaded to any route and then complain that that they have the wrong field (since none was supplied to that function).
fixed the problem. Thanks a whole lot @LinusU
Let me help here if I can. I had same problem. 2 hours to understand the error. When I was sending the Postamn field as photo, I had there some space after inserting photo in form-data name field. so multer was returning the error every time as it wasn't able to match photo (some white space) with upload.single('photo') in my code. I solved this by changing the name from photo in only p, in Postamn and in my app upload.single('p') Too stupid error but so hard to detect.
I was having the same error message.
In my case the problem was that I was using "upload.single('productImage')" in Node JS and in Postman I was using 'prodImg' inside the file field.
I changed the file field name to 'productImage' so that would match "upload.single('productImage')".
Now I get no more error messages.
Closing as original thread went inactive. This is a common error, if you experience it yourself try some of these solutions and if you you're still stuck you'll get more visibility opening a new issue ❤️
This worked for me. I also add the field name in my component.ts
public uploader:FileUploader = new FileUploader({ url: 'http://localhost:3000/api/upload', itemAlias: 'yourFieldName', });
I had to change my usage of upload.array('files')
to upload.array('files[]')
i same problem. if create two file input for upload ( thumbnail and gallery input ) but send three file ( avatar or any other input name ) , give this error !
this method is not resolve if send correct input name ( thumbnail or gallery ) (err, req, res, next)
this method give error after send not send correct input name : (req, res, next)
!!!!!
please help me.
@webafra have you tried the approaches listed above? For instance my comment on Apr 12.
@webafra have you tried the approaches listed above? For instance my comment on Apr 12.
No, my inputs are different. For example, I send a picture to Avatar and a PDF file. In this case, Malter makes a mistake and does not allow the process to continue.
@webafra Please create a codesandbox.io example showing your issue. There is no way to assist you based on your description.
I got the same issue and solve it. Your input name should me the same as upload.single('inputName')
<input id="inputfield" type="file" name="upfile">
app.post('/api/fileanalyse', upload.single("upfile"), (req, res) => {
res.json({
"name": req.file.originalname,
"type": req.file.mimetype,
"size": req.file.size
})
})
I was also getting this error then i did this step
just removed this app.use(upload.array()); or put this line after registering all routes
i have same issue how can be solve please tell me if you know i am stucking whole day.
app.post('/upload', (req,res)=>{ upload(req, res, err =>{ if(err){ console.log(err) }else{ console.log(req.file) } })
@mdaijaj Stuck the whole day and can't be bothered to format the question?
Check out some of the answers above, such as https://github.com/expressjs/multer/issues/690#issuecomment-645977419
app.use(upload.array());
This line is probably the problem, it shouldn't be there as it will catch all files uploaded to any route and then complain that that they have the wrong field (since none was supplied to that function).
Thanks!
If anyone wants to upload multiple files using multer and getting unexpected field error, try replacing upload.array('image')
to upload.any('image')
.
Thanks @osadasami -> knowing the field name is the same as the upload.single('inputName')
saved me!
I had to change my usage of
upload.array('files')
toupload.array('files[]')
@jrschumacher Doesn't work in my case.uploadImage.array('photos', 10),
oruploadImage.array('photos[]', 10),
![]()
app.use(upload.array());
This line is probably the problem, it shouldn't be there as it will catch all files uploaded to any route and then complain that that they have the wrong field (since none was supplied to that function).
It saved my day! didn't help directly but I knew what to look for after that i.e overridden config!! Thanks a ton!
`router.post("/", upload.single("**avatar**"), async (req, res) => {
try {
// Upload image to cloudinary
const result = await cloudinary.uploader.upload(req.file.path);
// Create new user
let user = new User({
name: req.body.name,
**avatar**: result.secure_url,`
Look for the parameter avatar of the first line with the object user is avatar too!
If you're getting the error MulterError: Unexpected field
and are stuck, read what others have suggested in this thread. In particular:
[]
to the input name if it there are multiple files (thanks to @jrschumacher)fields(fields)
method, where fields
is an array of objects with a name
, and optionally maxCount
, key.maxCount
. If you're using a middleware function that allows multiple files to be uploaded (array()
or fields()
), make sure the number of files uploaded doesn't exceed maxCount
.I've compiled a list of common reasons for this error (including solutions) and turned it into an article: 📚 Fix "Unexpected field" Error From Multer
Hopefully you fix the error and finally have working file uploads in Node.js using multer. 🌟
app.use(upload.array());
This line is probably the problem, it shouldn't be there as it will catch all files uploaded to any route and then complain that that they have the wrong field (since none was supplied to that function).
Ewana aha urandokoye musaza
I am having a similar problem but my api service does not have a html form since i intend to use it using flutter.
I encountered this error after 24hours of headache and fixing and scattering my code and was finally able to solve this, I discovered that multer stopped working with newer versions of some packaging, Mine was with express-jwt (with the newer version not taking function as the middleware) so what i did was install the older version and voila it worked for me.
If anyone wants to upload multiple files using multer and getting unexpected field error, try replacing
upload.array('image')
toupload.any('image')
.
This worked for me, Thank you so much
Hello, i've tried to upload a file with postman, but i get an "MulterError: Unexpected field", i search in all issues and i see all the time this is the field name. But for me i put the good filedname in postman and in my code.
here my code (sorry for screens, my first time posting code in issues):
here my postman request: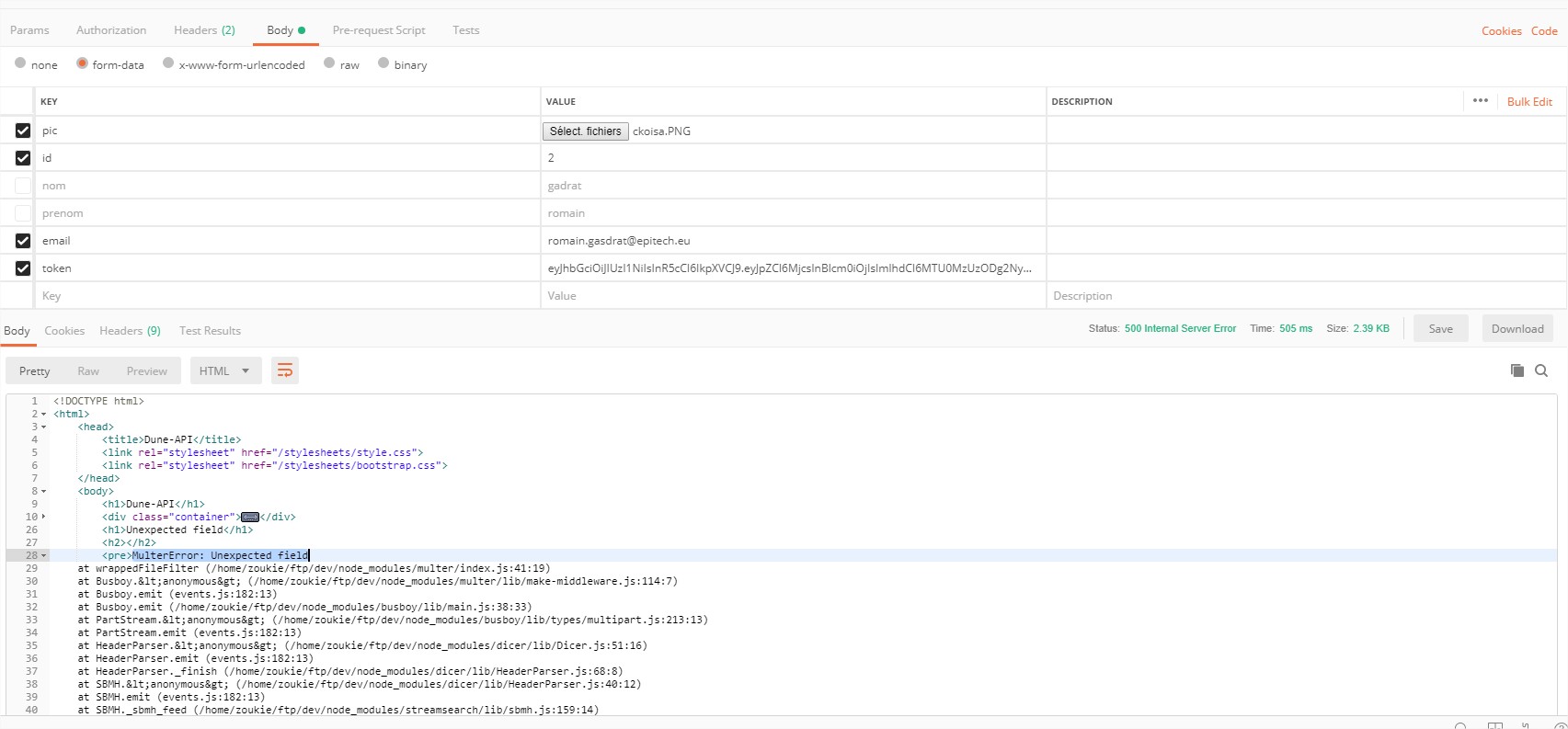
Thank your for the help.