Open mschneeweiss opened 5 years ago
implementation 'com.facebook.litho:litho-core:0.27.0' implementation 'com.facebook.litho:litho-widget:0.27.0' compileOnly "com.facebook.litho:litho-annotations:0.27.0" kapt 'com.facebook.litho:litho-processor:0.27.0' implementation "com.facebook.litho:litho-sections-core:0.27.0" implementation "com.facebook.litho:litho-sections-widget:0.27.0" compileOnly "com.facebook.litho:litho-sections-annotations:0.27.0" kapt "com.facebook.litho:litho-sections-processor:0.27.0"
Result: Children can get clipped.
Without padding (Text components get ellipsized correctly):
With padding (Text components clipped on the right edge):
Padding of Recycler should be accounted for when measuring its children. No unexpected clipping of children.
Code for the example shown in screenshots.
package com.example.lithorecyclerpaddingbug import android.graphics.Color import android.os.Bundle import android.text.TextUtils import androidx.appcompat.app.AppCompatActivity import com.facebook.litho.ComponentContext import com.facebook.litho.LithoView import com.facebook.litho.sections.Children import com.facebook.litho.sections.SectionContext import com.facebook.litho.sections.annotations.GroupSectionSpec import com.facebook.litho.sections.annotations.OnCreateChildren import com.facebook.litho.sections.common.SingleComponentSection import com.facebook.litho.sections.widget.RecyclerCollectionComponent import com.facebook.litho.widget.Text class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val context = ComponentContext(this) val component = RecyclerCollectionComponent.create(context) .section(MySection.create(SectionContext(context))) .leftPaddingDip(32f) .rightPaddingDip(32f) .build() setContentView(LithoView.create(context, component)) } } @GroupSectionSpec internal object MySectionSpec { @OnCreateChildren fun onCreateChildren( context: SectionContext ): Children = Children.create() .apply { for (i in 1..5) child(createTextComponentSection(context, i)) } .build() private fun createTextComponentSection(context: SectionContext, i: Int) = SingleComponentSection.create(context) .component( Text.create(context) .backgroundColor(Color.LTGRAY) .maxLines(1) .ellipsize(TextUtils.TruncateAt.END) .text("$i very long text which should be ellipsized since it's obviously very long") .build() ) }
any updates on this issue?
Version
Issues and Steps to Reproduce
Result: Children can get clipped.
Example
Without padding (Text components get ellipsized correctly):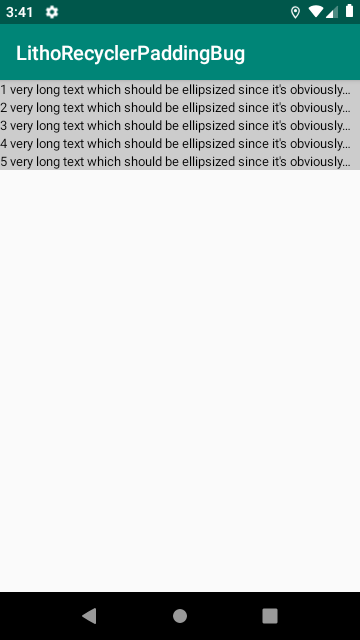
With padding (Text components clipped on the right edge):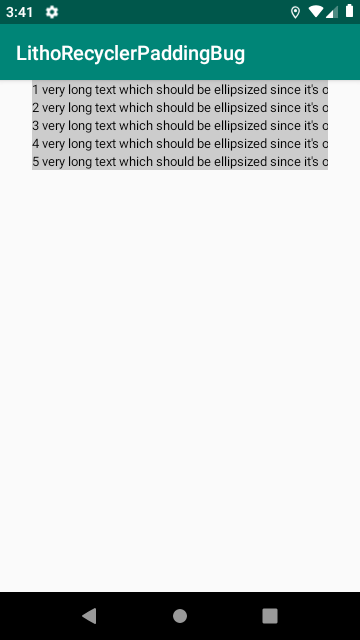
Expected Behavior
Padding of Recycler should be accounted for when measuring its children. No unexpected clipping of children.
Link to Code
Code for the example shown in screenshots.