Open thisisreallife opened 3 years ago
like #338 suggests, maybe I should tried increase the fourier_order
parameter or set a holiday date range.
I tried to increase the fourier_order
to 15-30 but it does not help... Set a holidy/window is unrealistic in my true business scenario.
Background
I perform seasonal-trend decomposation on timeseries. In my scenario, I cared about 3 different components, which are trend/weekly(7 days)/monthly(30.5 days as prophet doc suggests) components.
After we decompose each component, we may want to get the volume between and within each component. For example, we may want to compare weekly and monthly seasonality to tell which one is more important. For a particular seasonality component, take weekyly seasonality as example, we may need to know the strength of each weekday.
In prophet, we can draw decomposation with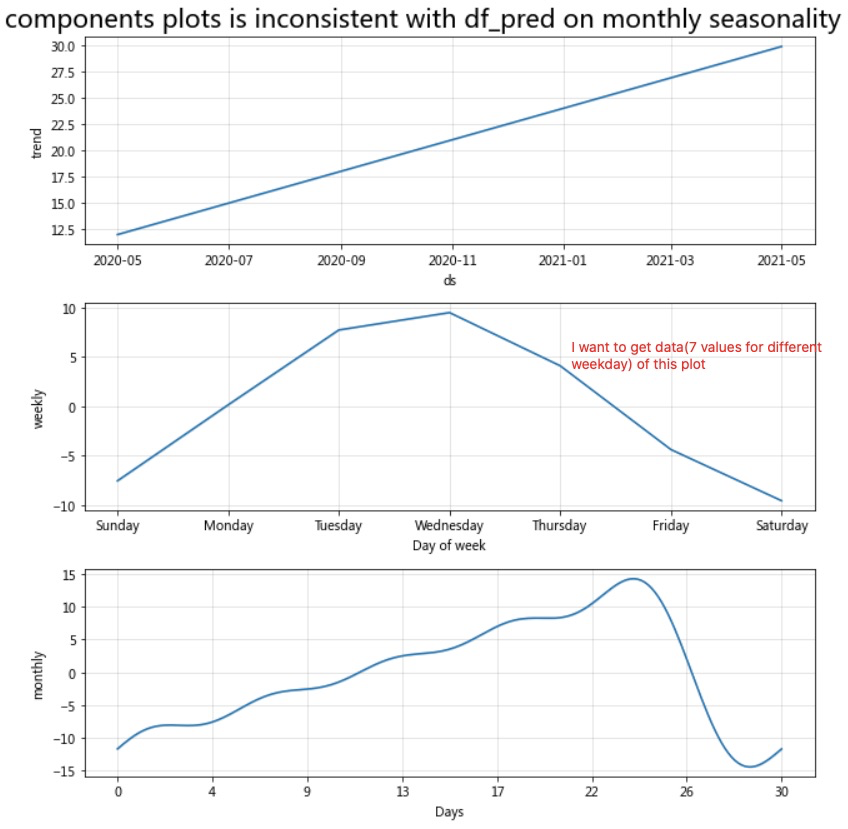
plot_components
method. I want to get the data points in each component subplot so that I can know estimated volume of each weekday/day of month.I make a synthetic dataset to reproduce my findings. The ground truth of monthly seasonality is linear, but the predicted value is confusing.
My attempt
I have no idea of getting data points in each component plot, so I tried to calculate them based on
df_pred = m.predict(future)
.It may work fine with built-in weekly seasonality
I think I can get data points of weekly seasonality componet using this code:
If we compare the code output and weekly component plot, we can find they are consistent.
Does not work with self-define monthly seasonality
Then I calculate monthly seasonality from
df_pred
too.However, I find inconsistent result on monthly seasonality:
df_pred.monthly
Summary of my question
m.add_seasonality('monthly', period= 30.5,fourier_order = 5)
). So that I get a wrong monthly component plot.m.plot_components
treat built-in weekly seasonality and self-define monthly seasonality differently.