Open cristianoccazinsp opened 4 years ago
I was able to work around this issue by creating a custom component as shown below, but it would great to see a proper fix for this.
import * as React from 'react';
import { TextInput } from 'react-native';
type Props = React.ComponentProps<typeof TextInput>;
const UndoTextInput: React.FC<Props> = ({ value, onChangeText, ...rest }) => {
const lastValue = React.useRef<string>();
const textInput = React.useRef<TextInput>(null);
const handleChangeText = (textValue: string) => {
lastValue.current = textValue;
onChangeText && onChangeText(textValue);
};
React.useEffect(() => {
if (value !== lastValue.current) {
lastValue.current = value;
textInput.current?.setNativeProps({ text: value });
}
}, [value]);
return (
<TextInput {...rest} ref={textInput} onChangeText={handleChangeText} />
);
};
export default UndoTextInput;
We are already using a UI library that wraps TextInput, so using the above doesn't really work for us.
Hey there, it looks like there has been no activity on this issue recently. Has the issue been fixed, or does it still require the community's attention? This issue may be closed if no further activity occurs. You may also label this issue as a "Discussion" or add it to the "Backlog" and I will leave it open. Thank you for your contributions.
This is still an issue.
I'm also seeing the occasional EXC_BAD_ACCESS "Attempted to dereference garbage pointer" error. @mhoran or someone please add the discussion
label so that the stale-bot won't close this issue!
This issue is stale because it has been open 180 days with no activity. Remove stale label or comment or this will be closed in 7 days.
Still an issue.
This issue is stale because it has been open 180 days with no activity. Remove stale label or comment or this will be closed in 7 days.
Confirmed still an issue but the workaround continues to work. Perhaps the fix for #27693 as proposed by @gedu in #39385 will help, but I'm not sure.
This is still an issue in react-native 0.73.4 / Expo v50. In addition, the workaround posted above needed value
added as a dependency to useEffect
to resolve a race condition.
This is how my fix looks like.
Seems that it fix the issue, if you can ping and make my PR more visible so it can be review and merged will be awesome.
If the steps I made aren't correct pleas send it again. I use the value
and onChange
props.
https://github.com/facebook/react-native/assets/1676818/48266bb1-2c16-4468-9278-bbf29ddab391
This issue is stale because it has been open 180 days with no activity. Remove stale label or comment or this will be closed in 7 days.
Still an issue.
I'm proposing another solution: https://github.com/facebook/react-native/pull/39385#issuecomment-2189249312
Please provide all the information requested. Issues that do not follow this format are likely to stall.
Description
Setting
value
, ordefaultValue
withonChange
will cause iOS undo/redo keyboard feature (mostly on iPads) to break or rarely work. Additionally, it may cause a native crash from time to time.Basically, the undo button gets enabled for half a second, and then gets disabled again. The issue will not happen if
value
ordefaultValue
is not set. I suspect this is related to the code that sends the JS value to native which in turn clears/updates the native input.React Native version:
Run
react-native info
in your terminal and copy the results here.Steps To Reproduce
<TextInput value={this.state.value}>
somewhere.Expected Results
Either undo/redo should not be possible, or it should behave just as if a native input was used (do not clear the stack after each component update)
Snack, code example, screenshot, or link to a repository:
The issue can be observed even with the sample code from the docs:
Additionally, the following crash will happen from time to time, presumably from this poor undo/redo handling of the component, or even Apple's source code.
Sample video: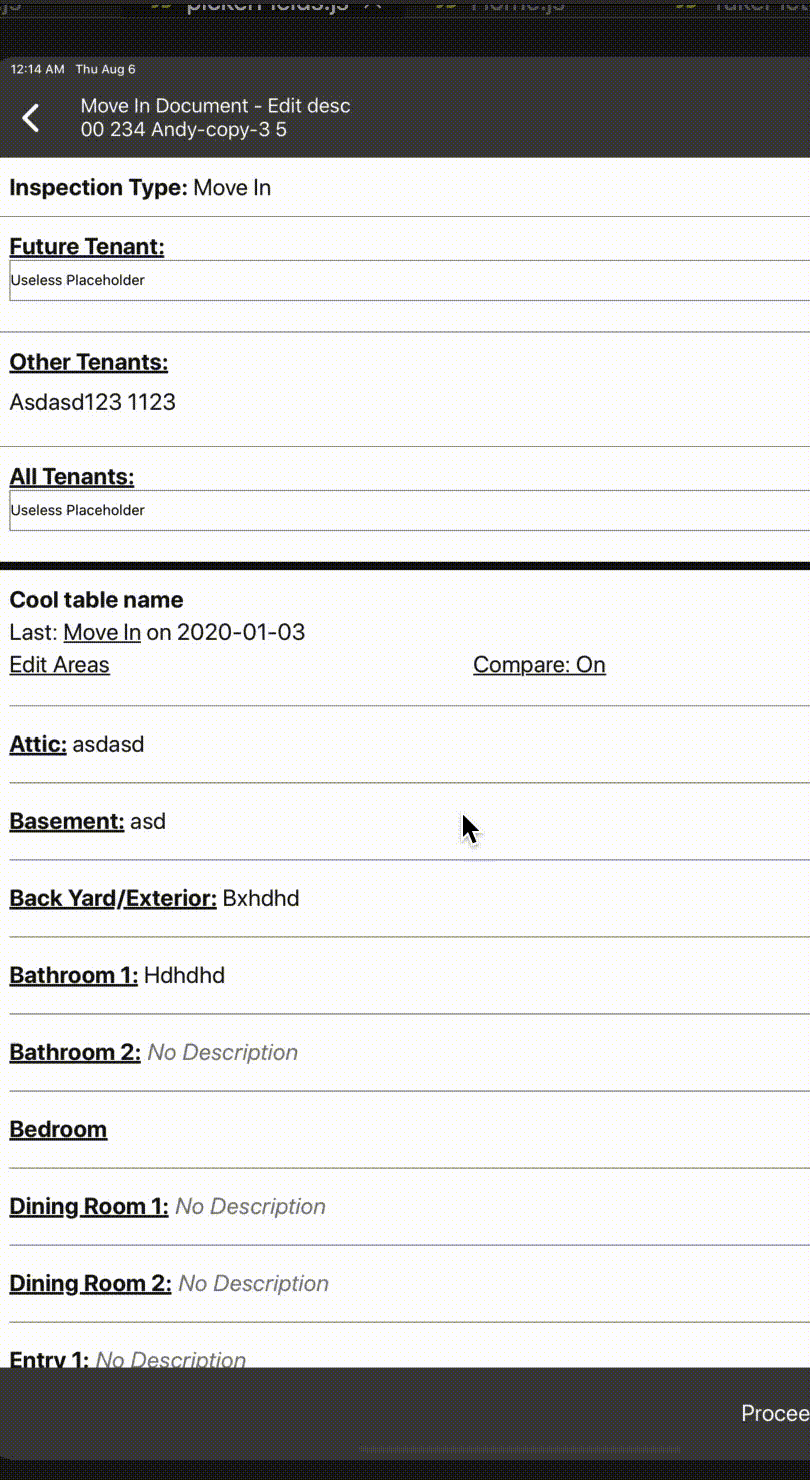