Open fangchuan opened 1 year ago
@Skylion007 thoughts?
Well, I haved digged into this problem, one cue is that the equirect_depth_image seems been stitched by 6 cubemap perspective images. Then I generate 6 cubic faces from the equirect_depth_image and unproject these cubic subviews' depth images to pointclouds, they looks ok, But the precision seems not good, the depth is likely been truncated cause of inappropriate depth scale or depth image format:
Finally, I merged 6 pointclouds generated by cubic subviews and get the following pointcloud:
I also confirmed that the depth map of equirectangular images is not correct, which I fixed this bug for python implementation in https://github.com/facebookresearch/habitat-lab/pull/517 You can see the details explanation here.
If I remembered correctly, they changed the implementation from python to magnum, so the bug might be included during that process. But I'm not familiar with magnum so I can't help with this.
Hi, I have rendered rgb/depth/semantic image from equirect_color_sensor/equirect_depth_sensor/equirect_semantic_sensor respectively. The rgb image seems fine, but I the value of depth image probably be wrong. Specificly,
code of step 1:
code of step 2 and 3:
code of step 3:
configuration: habitat-sim: 0.2.0 platform: ubuntu18.04
But the pointcloud is totally twisted, I want you to help me figure out if there is any problems during my processing codes. @aclegg3 @0mdc @dhruvbatra Thanks in advance! the output pointcloud of scene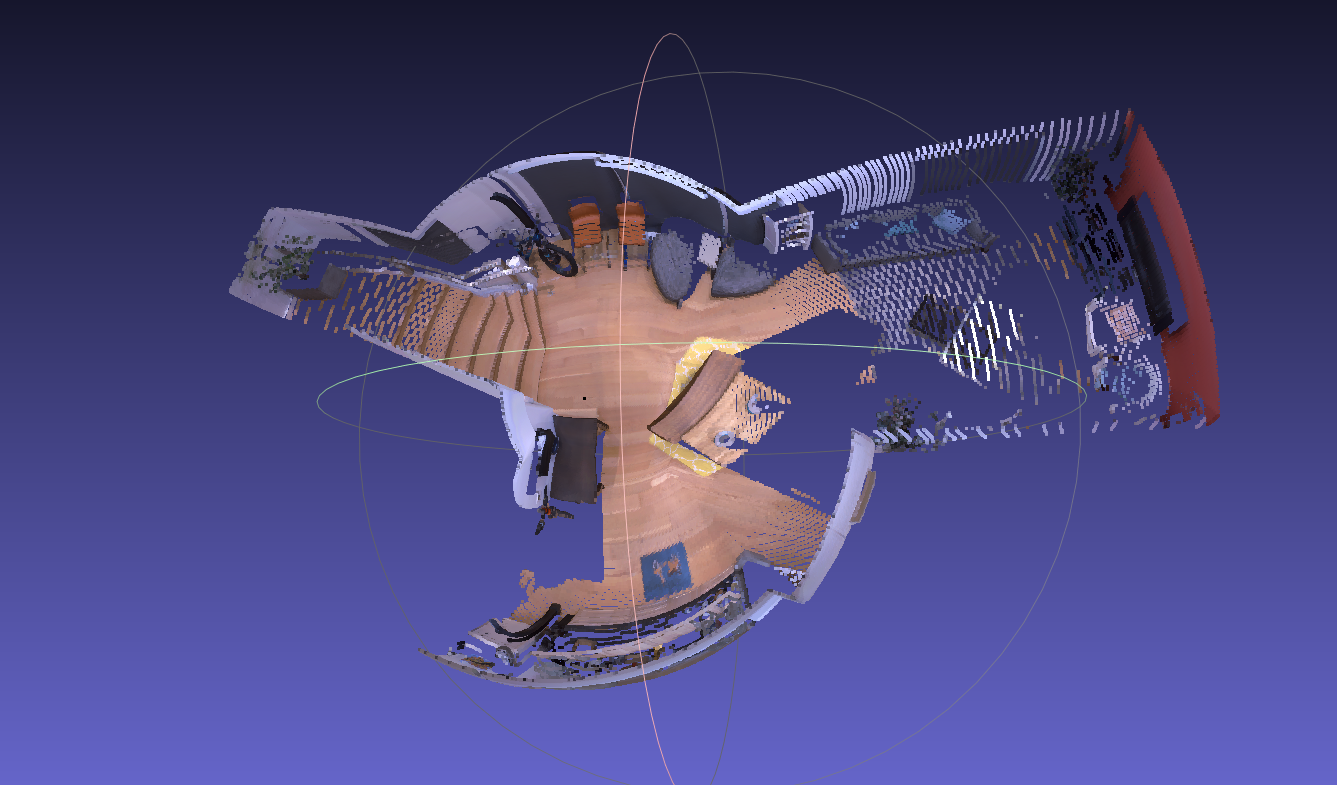
frl_apartment_0
: