Closed rishiagarwal2000 closed 1 year ago
@rishiagarwal2000 Thanks for the bug report.
First, it is important to note that internally Habitat connects to the Bullet C++ API, not PyBullet (which embeds much of its multi-body dynamics support directly into bindings layers and C Bullet integration). Therefore, the expectation of similar default behavior is somewhat unrealistic.
I've looked into this and noticed a couple of things.
sim.step_world()
excludes a time-step so uses the default of 1/60. I assume pybullet steps at the physics timestep of 1/240, so this would explain the first inconsistency. I'm not sure what pybullet sim would look like if it continued simulation. :thinking: # check removal and auto-creation
joint_motor_settings = habitat_sim.physics.JointMotorSettings(
position_target=0.0,
position_gain=1.0,
velocity_target=0.0,
velocity_gain=1.0,
max_impulse=1000.0,
)
existing_motor_ids = ao.existing_joint_motor_ids
for motor_id in existing_motor_ids:
ao.remove_joint_motor(motor_id)
ao.create_all_motors(joint_motor_settings)
btMultiBodyJointMotor
interface. I'm not completely sure how this difference will affect stability.Finally, I suggest you try out your simulations interactively in the python viewer.
Make the following small edit to the examples/viewer.py file at line 546:
elif key == pressed.T:
# load URDF
fixed_base = alt_pressed
urdf_file_path = "data/test_assets/urdf/amass_male.urdf" #or your own filepath
aom = self.sim.get_articulated_object_manager()
ao = aom.add_articulated_object_from_urdf(
urdf_file_path, fixed_base, 1.0, 1.0, True
)
ao.translation = (
self.default_agent.scene_node.transformation.transform_point(
[0.0, 1.0, -1.5]))
# check removal and auto-creation
joint_motor_settings = habitat_sim.physics.JointMotorSettings(
position_target=0.0,
position_gain=1.0,
velocity_target=0.0,
velocity_gain=1.0,
max_impulse=1000.0,
)
existing_motor_ids = ao.existing_joint_motor_ids
for motor_id in existing_motor_ids:
ao.remove_joint_motor(motor_id)
ao.create_all_motors(joint_motor_settings)
Then run it like:
python examples/viewer.py --stage-requires-lighting --scene data/test_assets/scenes/simple_room.glb
Press 'm'
to enter GRAB mouse mode and then click and drag the skeleton to play around with it like so:
https://user-images.githubusercontent.com/1445143/236301486-70676f66-5754-4c14-8b86-8c1769c0e033.mp4
With your file (default) simulation is unstable :frowning_face: :
https://user-images.githubusercontent.com/1445143/236301559-2f9f074b-e80c-4c38-aa42-b23ac956b374.mp4
With your file inertia loaded from URDF it is very stiff, but stable:
https://user-images.githubusercontent.com/1445143/236301684-ccd60e89-a9c2-489a-ae36-b5e03f24ba18.mp4
With your file inertia loaded from URDF but also scaled to 0.001 (from 1) we get what we might expect:
https://user-images.githubusercontent.com/1445143/236301912-cd326ecb-d32b-4454-9a04-9ea83cf7ac4a.mp4
Thank you so much @aclegg3. This is super helpful and clarifies a lot of questions. I appreciate your elaborate reply. I will try to incorporate these suggestions and if I face any further issues, I will post them here.
Habitat-Sim version
main
🐛 Bug
I tried simulating a urdf from here in habitat-sim and pybullet. The outputs for the simulation are attached. Apparently, the simulations differ significantly which seems unexpected to me because habitat-sim uses the same pybullet simulation internally. Also, the simulation is highly unstable. I am not sure if this is a bug, or if I am using it incorrectly. It would be great if you could help me with this.
Steps to Reproduce
Pybullet snippet
Habitat-sim snippet
settings.py imported by habitat-sim snippet
Expected behavior
Bullet simulation (expected)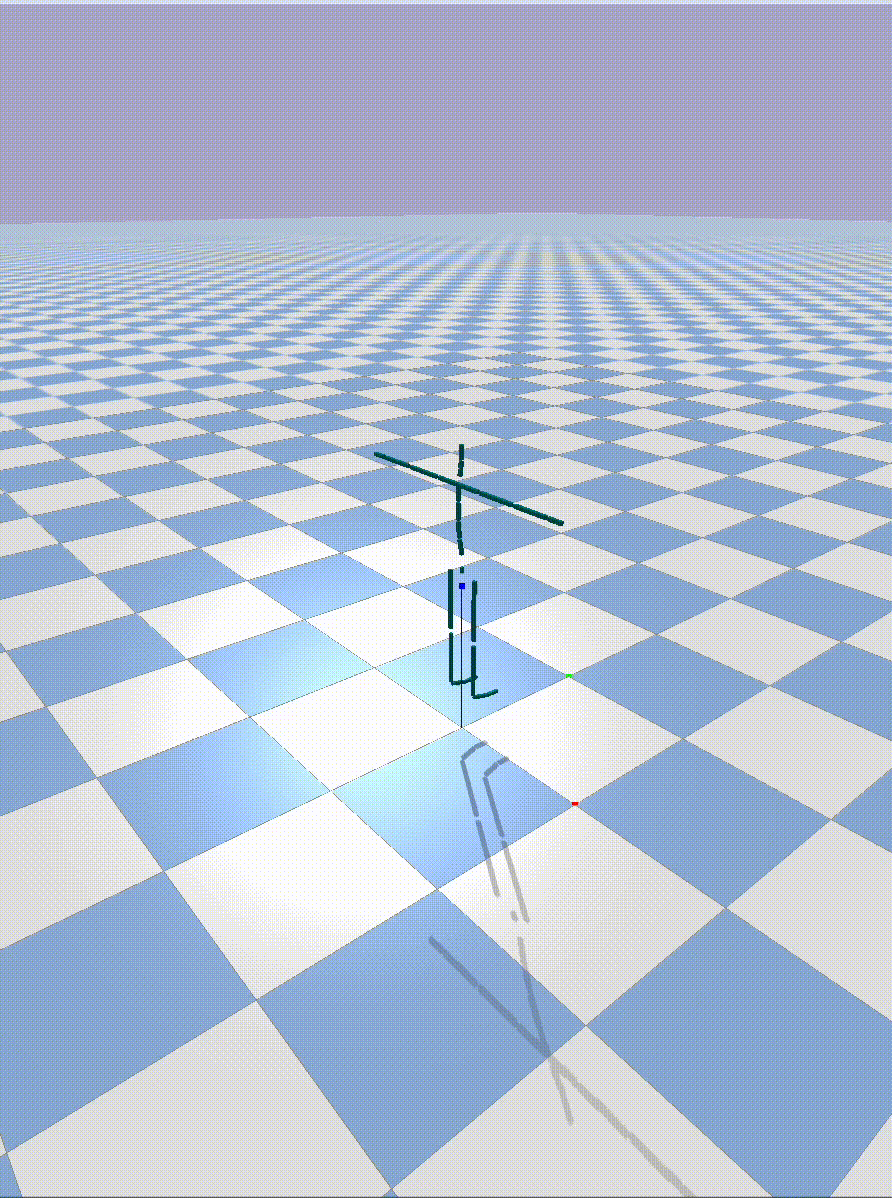
Habitat sim (unstable and way different)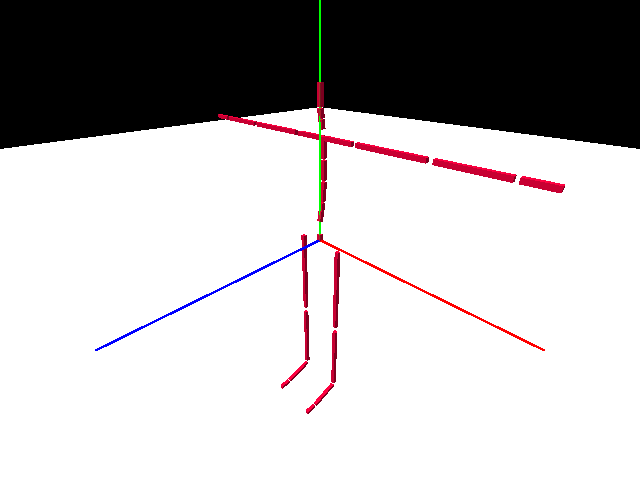
Additional context
System Info
You can run the script with:
conda
,pip
, source): pip