Closed kaustubh-sadekar closed 3 years ago
This happens because pixels end up living on the edge of the faces. They will go away if you increase your blur_radius
.
@gkioxari Thank you so much for solving my query. It is now clear to me why this error occurs. I also tried changing the blur_radius
. I am facing the error discussed in #342 and #327. Unlike #342 I need to render the texture using the TextureAtlas method as I have more than one texture file.
We are working on a fix for the issue of differentiability with TextureAtlas. The fix will be published soon.
🐛 Bugs / Unexpected behaviors
Unexpected white pixels are observed when rendering certain objects from different viewpoints. I changed the gamma parameter to verify if it is because of the opacity factory but the white pixels persist for any value of gamma.
Images for different values of gamma are shared here: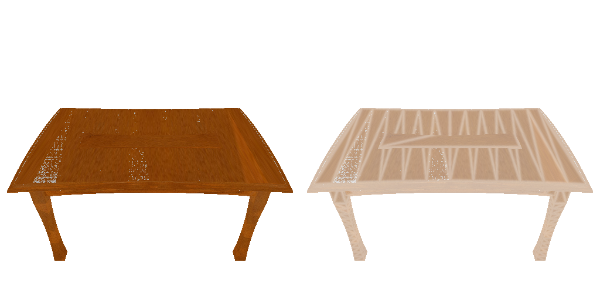
If I change the camera distance, the number of unexpected white pixels reduce, as shown in the following image: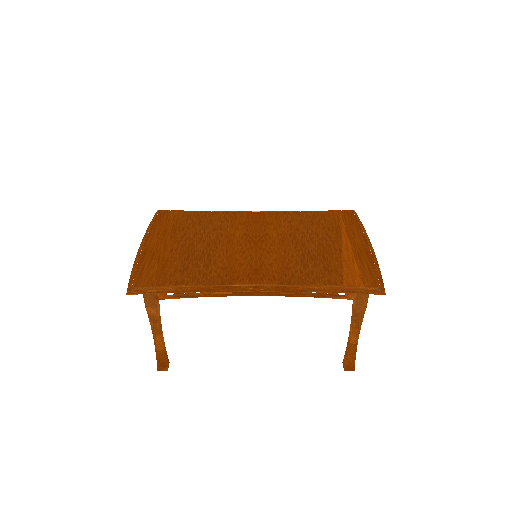
I am unable to understand why this is happening and how to solve the problem.
I am sharing the code and 3D models to reproduce the issue.
Instructions To Reproduce the Issue:
Link to the 3d model is provided here: data_folder.zip
Once again I would like to thank the contributors for their active guidance and kind support.