Closed xianyi11 closed 1 year ago
Hi, I run your code in my jupyter notebook, and there is nothing wrong.
The very tiny difference is that I copy the raw codes of cifar10_resnet.py into my notebook cell (as my notebook couldn't find ann2snn.sample_models somehow) . However, I don't think it's the key point :(
My suggestions are:
What's your version information? My pytorch is v1.13. And is your spikingjelly the newest?
_delete_all_unused_submodules is a method of torch.fx.GraphModule_. You can use fx_model=fx.symbolic_trace(model)
to get a torch.fx.GraphModule. And the call fx_model.delete_all_unused_submodules()
to check if there is any bug.
Thanks for your reply!!!!! as you said, the reason is my Pytorch version is too low to run the code. my pytorch version is 1.8.0. When I change it to 1.13.0, the error is gone.
When I run resnet18_cifar10.py in spikingjelly\spikingjelly\activation_based\ann2snn\examples\resnet18_cifar10.py, I encountered the error above. I run my code in jupyter notebook and my code is below. I only changed some path to fit my environment.
The error is: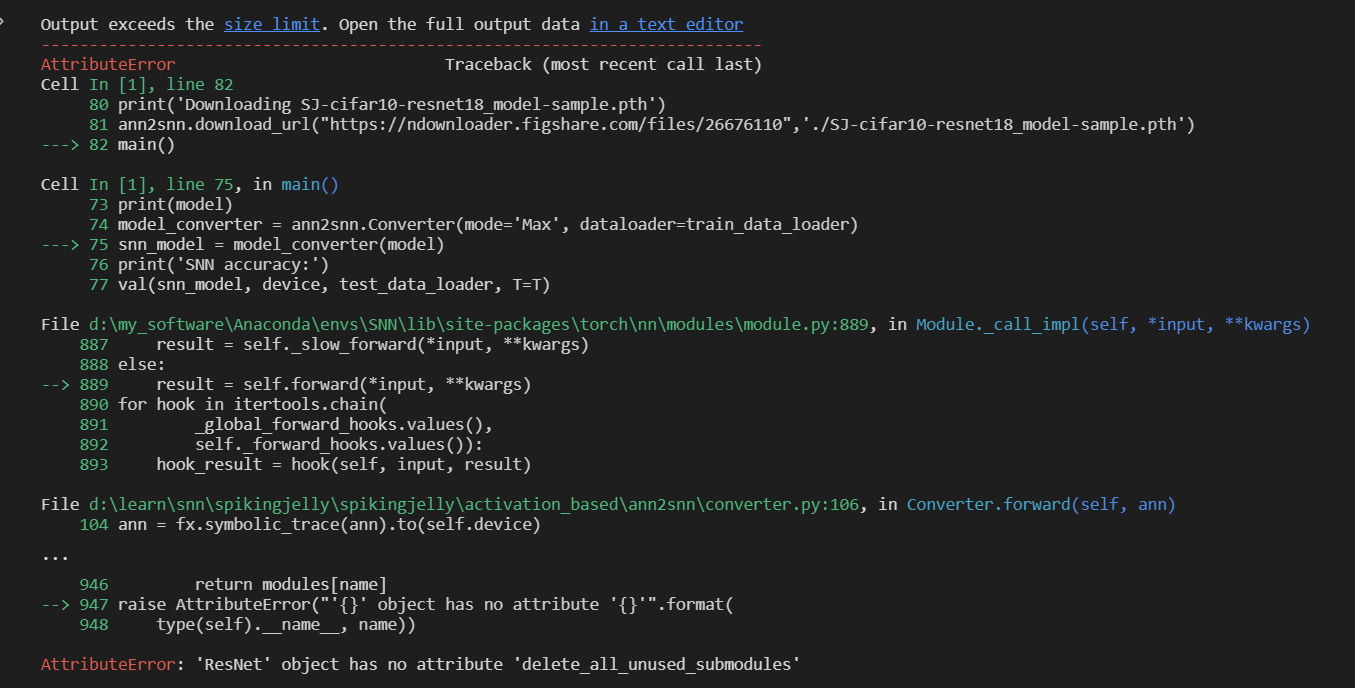
Thanks.