// current getCachedColor func
func getCachedColorCurrent(p Attribute) *Color {
colorsCacheMu.Lock()
defer colorsCacheMu.Unlock()
c, ok := colorsCache[p]
if !ok {
c = New(p)
colorsCache[p] = c
}
return c
}
// modified getCachedColor func
func getCachedColorAfter(p Attribute) *Color {
if c, ok := colorsCache[p]; ok {
return c
}
colorsCacheMu.Lock()
defer colorsCacheMu.Unlock()
c, ok := colorsCache[p]
if !ok {
c = New(p)
colorsCache[p] = c
}
return c
}
func BenchmarkGetCachedColorCurrent(b *testing.B) {
for i := 0; i < b.N; i++ {
getCachedColorCurrent(Underline)
}
}
func BenchmarkGetCachedColorAfter(b *testing.B) {
for i := 0; i < b.N; i++ {
getCachedColorAfter(Underline)
}
}
reduce unnecessary mutex lock if instance already created.
refer: http://marcio.io/2015/07/singleton-pattern-in-go/
Benchmark result: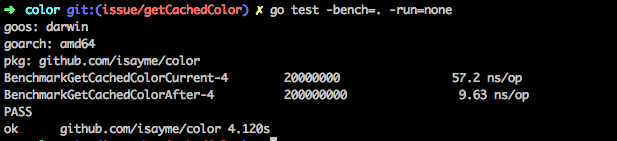
Benchmark code