Closed guidol70 closed 3 years ago
im pretty sure you can do the keyboard click noise using
SoundGenerator SND;
VICNoiseGenerator VICNoise;
void setup() {
SND.attach(VICNoise);
}
void kb_snd() {
VICNoise.setFrequency(900); //set 900 to whatever you want
delay(10); //also set 10 to whatever
VICNoise.setFrequency(0);
}
If you dont want it to delay the program for 10ms then you could probably set up a timer thing and have it poll the timer:
uint64_t tsv;
uint64_t timer() {
return millis() - tsv;
}
void resetTimer() {
tsv = millis();
}
Please take a look at Terminal.setColorForAttribute() new method. Unfortunately just colors defined in Color enum are usable, so no Orange, unless you are using VGA16Controller and you change the palette with VGA16Controller.setPaletteItem().
About sound on clik, with the last commit you may do this:
Terminal.onVirtualKey = [&](VirtualKey * vk, bool keyDown) {
if (keyDown)
Terminal.soundGenerator()->playSound(SquareWaveformGenerator(), (*vk == VirtualKey::VK_RETURN ? 1000 : 2000), 4);;
};
...or maybe this...
Terminal.onVirtualKey = [&](VirtualKey * vk, bool keyDown) {
if (keyDown)
Terminal.soundGenerator()->playSound(VICNoiseGenerator(), (*vk == VirtualKey::VK_RETURN ? 90 : 120), 10);;
Terminal.soundGenerator()->playSound(SquareWaveformGenerator(), (*vk == VirtualKey::VK_RETURN ? 1000 : 2000), 4);;
};
I'm not sure if Oric Atmos click is a square wave or just noise... ;-)
@fdivitto WOW - the code worked in the 1st place when inserted in my RunCPM :)
I used your "yellow-bright"-example from the SimpleTerminalOut.ino
Terminal.setColorForAttribute(CharStyle::Bold, Color::BrightYellow, false);
and the "maybe this" example from above:
Terminal.onVirtualKey = [&](VirtualKey * vk, bool keyDown) { if (keyDown) // Terminal.soundGenerator()->playSound(VICNoiseGenerator(), (*vk == VirtualKey::VK_RETURN ? 90 : 120), 10);; Terminal.soundGenerator()->playSound(SquareWaveformGenerator(), (*vk == VirtualKey::VK_RETURN ? 500 : 1000), 4);;
Compiled without errors in the first place ;) - for me a great experience now! Many Thanks!!
Now the longer part for me
[EDIT] Done - RunCPM v5.1 for TTGO VGA32 with keyclick & bold-text-color available at https://drive.google.com/file/d/1WzCwLKJTaCxvtC9XRImF_nTKi6-Jl3ph/view?usp=sharing
VGA16Controller.setPaletteItem() Please take a look at Terminal.setColorForAttribute() new method. Unfortunately just colors defined in Color enum are usable, so no Orange, unless you are using VGA16Controller and you change the palette with VGA16Controller.setPaletteItem().
@fdivitto maybe you should change for the example in the docu for SetPaletteItem from displayController.setPaletteItem(0, RGB(255, 0, 0)); to displayController.setPaletteItem(0, RGB888(255, 0, 0));
because on compile I did get the error RGB is not defined and it worked with RGB888 like in the vga16controller.cpp
// Set Color 15 to AMBER-Orange for Retro-Display
// https://rgbcolorcode.com/color/amber
// RGB(255,191,0)
DisplayController.setPaletteItem(15, RGB888(255, 191, 0));
@guidol70 just fixed thanks!!
@fdivitto I try to set the AMBER-color in the confgialog.h of the ANSI-Terminal for the VGA16Controller (if I use a VGA16Controller resolution).
But the command isnt recognized, because the DisplayController is tied to BitmappedDisplayController also when DisplayController is redefined with
case ResolutionController::VGA16Controller:
DisplayController = new fabgl::VGA16Controller;
I dont understand why I cant do
case ResolutionController::VGA16Controller:
DisplayController = new fabgl::VGA16Controller;
DisplayController->setPaletteItem(9, RGB888(255, 191, 0));
break;
Is that to early before
DisplayController->begin();
How could I archive this at this point only when a VGA16Controller is selected?
Do you got a idea/sourceline?
@fdivitto I did make me a terminal for my needs ;) I took the Terminal-parts out of RunCPM VGA32 and some parts of the FabGL-ANSI-Terminal to get a 80x25 Terminal using the VGA16Controller.
There I could set a part of my palette to orange ;)
Named this little the fork GLTerm - if anyone want to test its get at https://github.com/guidol70/RunCPM_VGA32/tree/main/GLTerm_VGA32
Hi,
as you wish - here is/are my feature-request(s):
1.) It would be nice if you could add an option to replace the font-bold-attribut with a different color (like bold is shown as (non-bright) orange while the foreground text-color is green) (see wordstar picture attachment) This is an option in the puTTY-Terminal for Windows/Linux. There is also the option to select the cursor-color ;)
2.) Another option I would like to see/hear would be the option for a key-click-sound like on the ATARI XL (same sound for every key) or a little bit better like on the Oric Atmos where the normal key have the samen sound, but the ENTER/RETURN-key does have another (deeper) sound ;)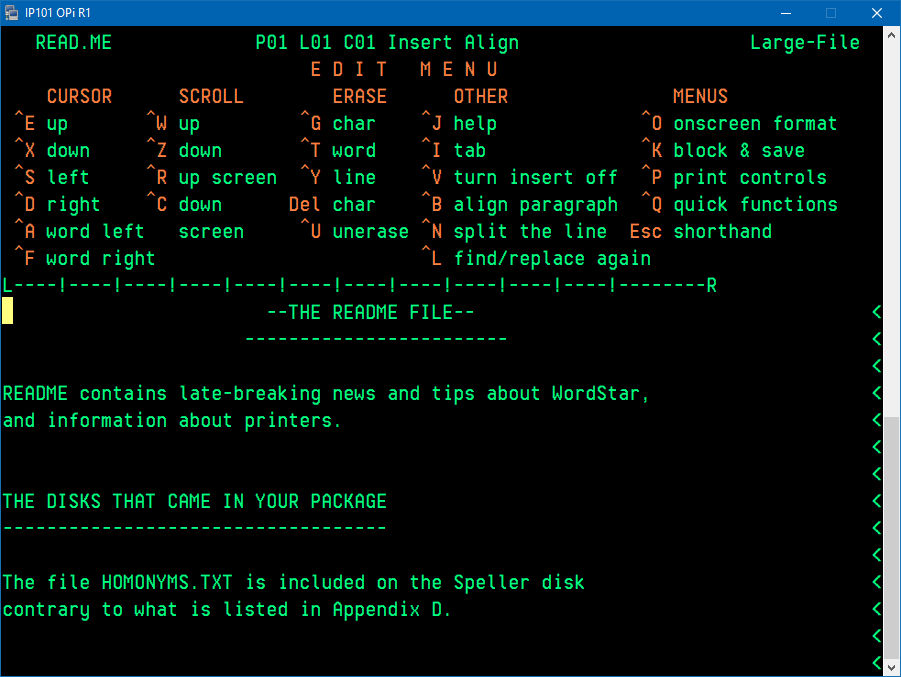