Closed boukmi closed 4 years ago
Any help please?
We're closing this issue because it seems to be a question or request for help rather than a clearly reproducible bug, regression or new feature request.
Good places to ask questions or work through problems where you're more likely to get a fast response include:
Thanks for using Flutter!
Closing based on this comment
This thread has been automatically locked since there has not been any recent activity after it was closed. If you are still experiencing a similar issue, please open a new bug, including the output of flutter doctor -v
and a minimal reproduction of the issue.
Hello everybody,
I'm trying to add support for a new language in my Flutter application by following this tutorial but unfortunately that does not seem to work.
I've added a new localisations delegate:
I have't added all the translations yet, I wanted to make sure that it's working first.
This is the
app.dart
fileAnd
pubspec.yaml
I get an exception when I try to change app language to the newly added one (the third option on the screenshot)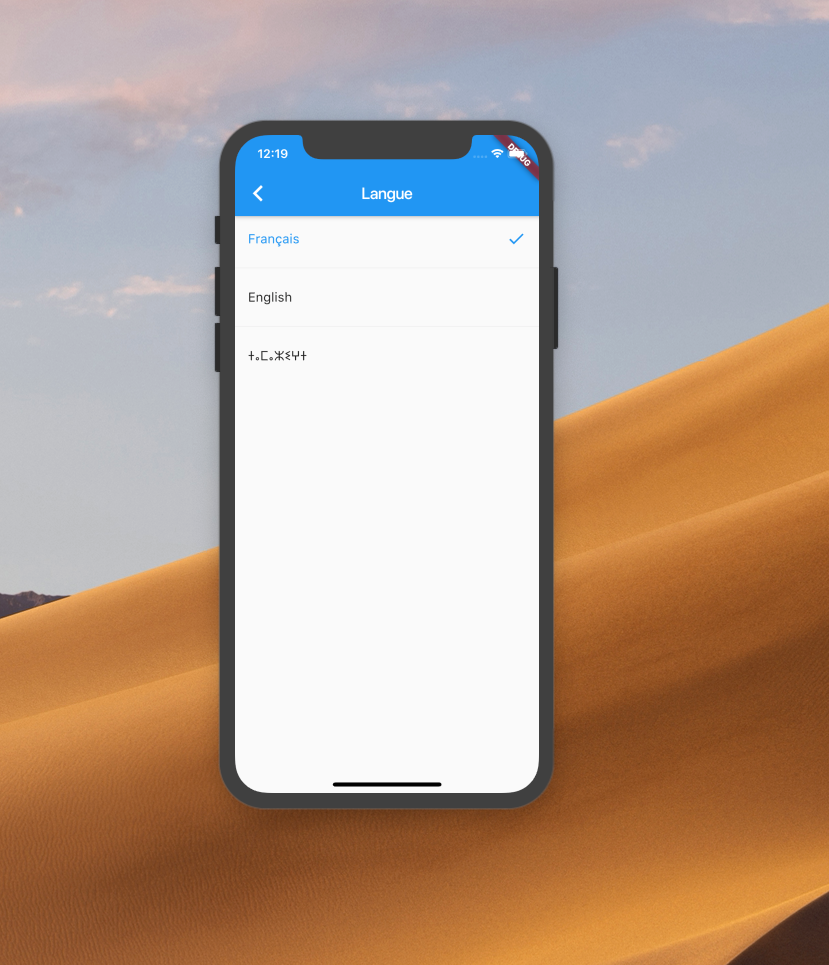
flutter analyze
:flutter doctor -v
Thank you so much for your help