Open kareheavy opened 5 years ago
@kareheavy I guess it's a good question for StackOverflow. I would advise you to post it there as well as help there may come faster.
CC @clocksmith
Hi @kareheavy, This issue doesn't seem to describe a bug or a feature request. Please see https://flutter.dev/community for resources and asking questions like this. You may also get some help if you post it on Stack Overflow and if you need help with your code, please see https://www.reddit.com/r/flutterhelp/ Closing, as this isn't an issue with Flutter itself. If you disagree, please write in the comments and I will reopen it. Thank you
Try wrapping the your slider in a SizedBox widget, with a required height. Ugly- but works . SizedBox( height: 20, child: SliderTheme(...));
This thread has been automatically locked since there has not been any recent activity after it was closed. If you are still experiencing a similar issue, please open a new bug, including the output of flutter doctor -v
and a minimal reproduction of the issue.
This has been raised to me elsewhere. We can add support to adjust default padding similar to other widgets.
I want to change the height or padding, i dont know what property is,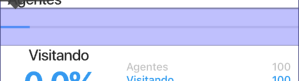
I use this class for remove the margins on both sides