Open giorgio79 opened 4 years ago
google_maps_flutter: ^1.0.4
The issue is replicable on latest flutter stable channel with google_maps_flutter: ^2.0.6
.
Used this code sample
I am also facing the same issue while opening the screen with SliverAppbar and ListView.builder(). On initial screen loading appbar lags like 30 FPS or even worse.
Has anyone found a solution for this yet? I have the same problem but only in release mode.
It seems like more generally resizing a GoogleMap
cause lag spikes. I'm also experiencing lag when animating the size of a GoogleMap
, both on iOS and Android.
SliverAppBar with a Google Map is lagging when I expand. Please see a video. Also confirmed on actual device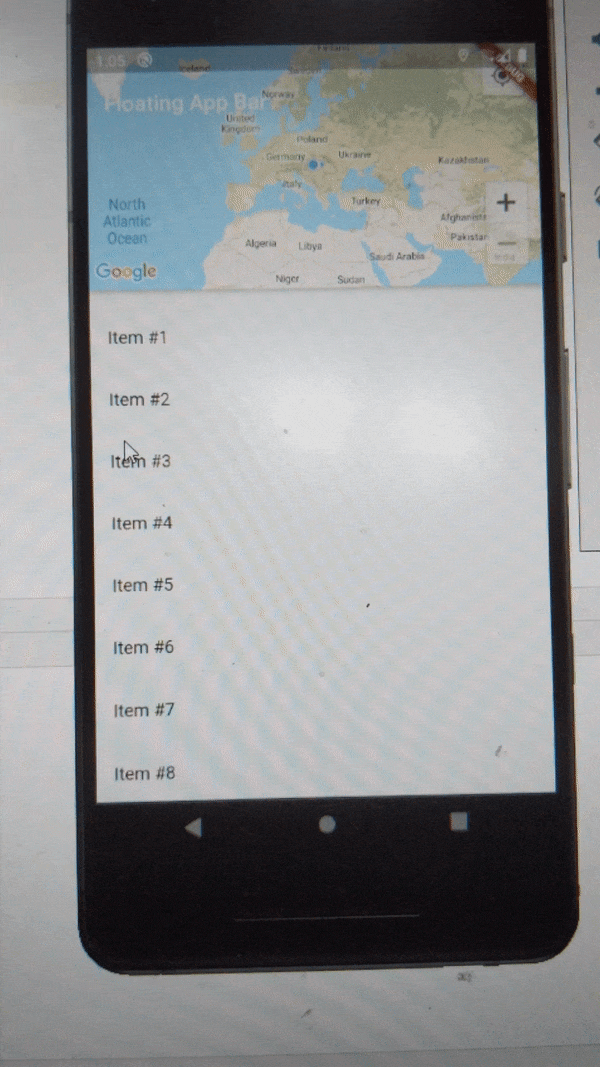
Here is the code
Output of flutter doctor