Closed Dsantra92 closed 4 years ago
Its not an issue with the for loop as such
As @shashankp pointed out, you need to keep all assignments and modifications (+=
) within a single cell - otherwise reactivity can't work.
So:
begin
s = 1
for i in 1:10
s += i
end
s
end
but this still won't work, because for
introduces a scope. If you assign to s
within the for
loop, then you are assigning to a local variable, also called s
, that is only defined within that loop. If you want to read more, this is called an ambiguous assignment inside a soft scope:
https://docs.julialang.org/en/v1/manual/variables-and-scoping/#Local-Scope
Confusingly, Pluto falls in the category "non-interactive"
The solution is to use local
:
s = begin
local s = 1
for i in 1:10
s += i
end
s
end
or:
s = begin
local my_s = 1
for i in 1:10
my_s += i
end
my_s
end
But for
and while
loops have so-called "functional" alternatives. The big ones are map
, filter
, reduce
and sum
:
begin
s = 1
s += sum(1:10) do i
i
end
end
Functional programming works very nicely together with Pluto - try it out!
Sorry for all that strange syntax!
@fonsp @shashankp thank you for this wonderful clarification.
The notebook throws a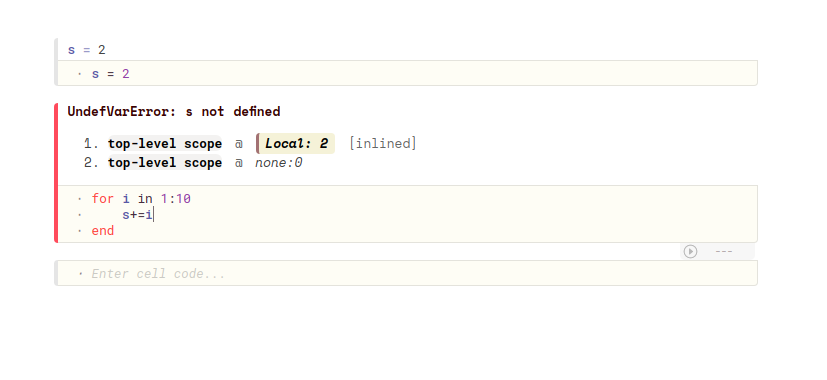
UndefVarError
while accessing the global variables inside the for loop.