Closed tortuetorche closed 3 years ago
Hi @claar and @stayallive,
As you know or not, I'm a member of this repository, so I can merge myself this pull request. But I wasn't a very active contributor until now, so if you're OK I can merge this one, otherwise let me know...
I've also send other pull requests to fix pending issues... Then, my goal is to support Bootstrap 5, if I find the time to do it 🤞
Cheers, Tortue Torche
Hi folks,
I'm going to merge my pending pull requests and then, after some testing, tag a 5.0.0 release 👍
Have a good day, Tortue Torche
Basic usage with Laravel and Bootstrap 4
Will produce: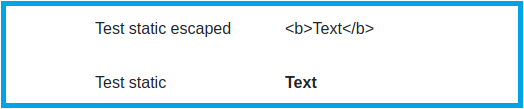
Advanced usage with Laravel and Bootstrap 4
If you populate your form, you should use the
forceValue()
method instead of thevalue()
method, like this:Will produce:
But the best way is to directly populate data which are already marked as safe HTML, like this:
So you don't have to use
value()
orforceValue()
methods in your views 👍HtmlString
class and safe HTML tagsBut what is
Illuminate\Support\HtmlString
class used in the code above? An instance of theHtmlString
class can potentially check whether a string it's been given should be treated as HTML or not.So when you do:
You tell to your Laravel views that the string given, which can contains HTML tags, is safe and shouldn't be escaped. In addition, you should use an HTML purifier to remove automagically unsafe HTML tags (e.g. https://github.com/mewebstudio/Purifier).
Enable or disable this feature with Laravel
In your former config file
config/former.php
, you can enable or disable this feature:Breaking change
⚠️ Important ⚠️: This pull request could be considered as a breaking change, but the behavior proposed here is much safer than the previous behavior.
Maybe we can add a config option to disable this new behavior?You can disable this new behavior with theescape_plaintext_value
former config option set tofalse
, see previous paragraph. But IMHO it should be enabled by default 🔒