Open woojintaek opened 1 year ago
I am trying to use Froala editor in Next.js, but I am getting an error during the build process. Is there a solution to this problem?
import "froala-editor/js/froala_editor.pkgd.min"; import FroalaEditor from "react-froala-wysiwyg"; export default function MyFroalaEditor({ tag, config, model, onModelChange }: any) { return <FroalaEditor tag={tag} config={config} model={model} onModelChange={onModelChange} />; }
import dynamic from "next/dynamic"; import { useState } from "react"; import froalaConfig from "@/commons/froala/froalaConfig"; import "froala-editor/css/froala_style.min.css"; import "froala-editor/css/froala_editor.pkgd.min.css"; const MyFroalaEditor = dynamic(() => import("./froala"), { ssr: false, }); export default function SampleFroalaPage() { const [editorState, setEditorState] = useState({ model: "" }); return ( <div> <MyFroalaEditor tag="textarea" model={editorState.model} config={froalaConfig.config} onModelChange={(newModel: any) => { setEditorState({ ...editorState, model: newModel, }); }} /> </div> ); }
const froalaConfig = { config: { placeholderText: "placeholderText", charCounterCount: true, toolbarButtons: { moreText: { buttons: [ "bold", "italic", "underline", "strikeThrough", "subscript", "superscript", "fontFamily", "fontSize", "textColor", "backgroundColor", "inlineClass", "inlineStyle", "clearFormatting", ], align: "left", buttonsVisible: 3, }, moreParagraph: { buttons: [ "alignLeft", "alignCenter", "formatOLSimple", "alignRight", "alignJustify", "formatOL", "formatUL", "paragraphFormat", "paragraphStyle", "lineHeight", "outdent", "indent", "quote", ], align: "left", buttonsVisible: 3, }, moreRich: { buttons: [ "insertLink", "insertImage", "insertVideo", "insertTable", "emoticons", "fontAwesome", "specialCharacters", "embedly", "insertFile", "insertHR", ], align: "left", buttonsVisible: 3, }, moreMisc: { buttons: ["undo", "redo", "fullscreen", "print", "getPDF", "spellChecker", "selectAll", "html", "help"], align: "right", buttonsVisible: 2, }, }, }, }; export default froalaConfig;
"next": "13.3.1", "react-froala-wysiwyg": "^4.0.18",
const nextConfig = { distDir: BUILD_DIR, trailingSlash: true, compiler: { styledComponents: true, }, }; module.exports = nextConfig;
{ "env": { "browser": true, "es6": true, "node": true }, "extends": [ "eslint:recommended", "plugin:react/recommended", "airbnb", "airbnb-typescript", "plugin:prettier/recommended" ], "overrides": [ ], "parser": "@typescript-eslint/parser", "parserOptions": { "project": "./tsconfig.json", "ecmaFeatures": { "jsx": true }, "ecmaVersion": "latest", "sourceType": "module" }, "plugins": ["react", "react-hooks", "prettier"], "rules": { "react/react-in-jsx-scope": 0, "react/prefer-stateless-function": 0, "react/jsx-filename-extension": 0, "react/jsx-one-expression-per-line": 0, "no-nested-ternary": 0, "react/jsx-props-no-spreading": 0, "import/no-extraneous-dependencies": "off", "no-param-reassign": [ "error", { "props": false }], "no-console": ["error", { "allow": ["warn", "error","info"] }] }, "settings": { "import/resolver": { "typescript": { "alwaysTryTypes": true } } } }
{ "compilerOptions": { "target": "es5", "lib": ["dom", "dom.iterable", "esnext"], "allowJs": true, "skipLibCheck": true, "strict": true, "forceConsistentCasingInFileNames": true, "noEmit": true, "esModuleInterop": true, "module": "esnext", "moduleResolution": "node", "resolveJsonModule": true, "isolatedModules": true, "jsx": "preserve", "incremental": true, "paths": { "@/*": ["./src/*"] } }, "include": ["next-env.d.ts", "**/*.ts", "**/*.tsx", "**/*.js", "**/*.jsx"], "exclude": ["node_modules"] }
@woojintaek Did you eventually get this fixed I am facing the same issue now
@woojintaek 안녕하세요! 혹시 해당 문제 해결하셨나요? 저도 똑같은 문제를 겪고 있습니다..
Question
I am trying to use Froala editor in Next.js, but I am getting an error during the build process. Is there a solution to this problem?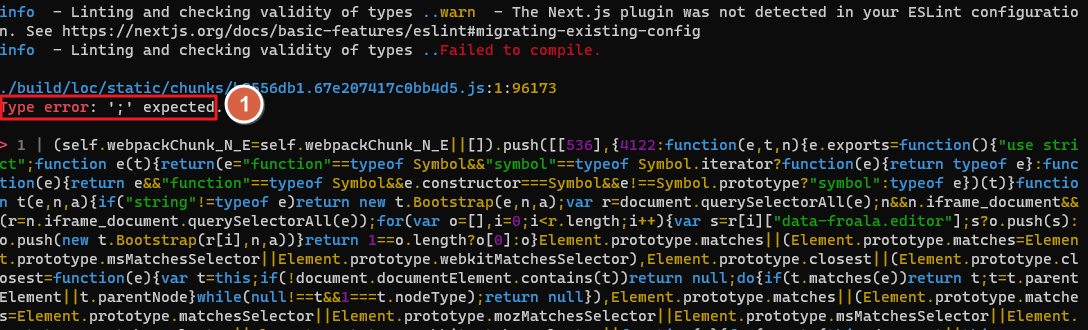
froala.tsx
sample_froala.tsx
froalaConfig.ts
package.json
next.config.js
eslint.json
tsconfig.json