Closed 3point14guy closed 7 years ago
The first step is probably to check that you're sending the right data in your request. You can do this by loading your game in the browser, opening up Chrome dev tools, and clicking the Network tab. Then, play the game until it sends the PATCH request, click on the request, and click "Preview".
Here's a screenshot of (of someone else's project) to illustrate: http://imgur.com/a/wsB5n
What does your data look like? Does it match what the API expects, as described in the README here: https://github.com/ga-wdi-boston/game-project-api?
okay. so it doesn't like how i am putting in my data. I am trying to create variables in my game logic file that I can use in the files where I call the GET. I am having difficulty stumbling on a combination that works.
If I move my GET request to my game logic I can it to work now, but not when they are in separate files.
OK! I can get the updates working. I changed to grouping the (index, letter and gameOver) under a single variable and did not pass them directly in the PATCH (not GET!!) request. Thanks for looking CALEB!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
Can you post what you see in the preview tab for the PATCH request?
{game: {id: 4542, cells: ["x", "", "", "", "x", "", "", "x", "o"], over: false,…}} game : {id: 4542, cells: ["x", "", "", "", "x", "", "", "x", "o"], over: false,…}
I got it now! Trying to get those variables from my game logic is part of what wasn’t working.!
On Jul 16, 2017, at 1:16 PM, Caleb Pearce notifications@github.com wrote:
Can you post what you see in the preview tab for the PATCH request?
— You are receiving this because you modified the open/close state. Reply to this email directly, view it on GitHub https://github.com/ga-wdi-boston/game-project/issues/847#issuecomment-315623248, or mute the thread https://github.com/notifications/unsubscribe-auth/AbgZp86piNwZCnBRY7tkGlcZvNkIBwMoks5sOkWEgaJpZM4OZKBT.
I have literally been at this all day and keep thinking I've got it but, then no.
I am capturing my game id and the patch request has the same game id.
I am capturing the locations of the moves, the values of the moves, and the status of the game as a boolean.
console log shows that I am going through my api code and sending my AJAX request, but it comes back 404.
I have gone through the closed issues and looked at many that were similar to mine, but the light bulb isn't lighting.
I did google searches and most my searches don't find info in a similar format to what we are doing here. I followed many of the examples and looking at them and the issue queue has allowed me to get this far.
I think the problem is in the way I am sending the data, but I don't know what is wrong about it.
Here is the console log: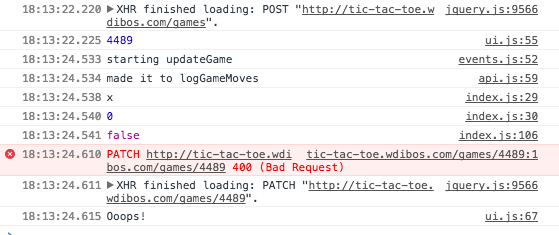
Here is my code:
Thanks for your insights!