Open FirefoxMetzger opened 3 years ago
Any update on this? This issue is causing major issues for a project I'm on.
Not really sure which Gazebo version are you using @andyblarblar, but I backported some commits that should fix the issue https://github.com/gazebosim/gz-sensors/pull/383
Not really sure which Gazebo version are you using @andyblarblar, but I backported some commits that should fix the issue https://github.com/gazebosim/gz-sensors/pull/383
Yeah, sorry for not giving more details. I've been having issues in garden 7.6 binary release. I can try fortress as well to see if anything has changed, because I'm on Humble.
This seems to still be a problem in Gazebo Harmonic. I changed the resolution of my camera to a higher resolution and checked the camera_info message which now has an intrinsic matrix that doesn't make much sense (especially cx and cy).
header:
stamp:
sec: 7
nanosec: 992000000
frame_id: spawn_robot/base_link/boundingbox_camera
height: 1536
width: 2048
distortion_model: plumb_bob
d:
- 0.0
- 0.0
- 0.0
- 0.0
- 0.0
k:
- 277.0
- 0.0
- 160.0
- 0.0
- 277.0
- 120.0
- 0.0
- 0.0
- 1.0
r:
- 1.0
- 0.0
- 0.0
- 0.0
- 1.0
- 0.0
- 0.0
- 0.0
- 1.0
p:
- 277.0
- 0.0
- 160.0
- -0.0
- 0.0
- 277.0
- 120.0
- 0.0
- 0.0
- 0.0
- 1.0
- 0.0
binning_x: 0
binning_y: 0
roi:
x_offset: 0
y_offset: 0
height: 0
width: 0
do_rectify: false
Environment
Description
Steps to reproduce
Example World 1 (camera resolution 320x240 [default])
```xmlExample World 2 (camera resolution 640x480)
```xmlBoth worlds are identical except for the resolution of the camera. The camera resolution being different means that the projection matrix published under
/camera_info
should change; however, it doesn't. For both worlds it iswhereas for the second world, it should be
This leads to problems down the line because projections are off:
Projection World 1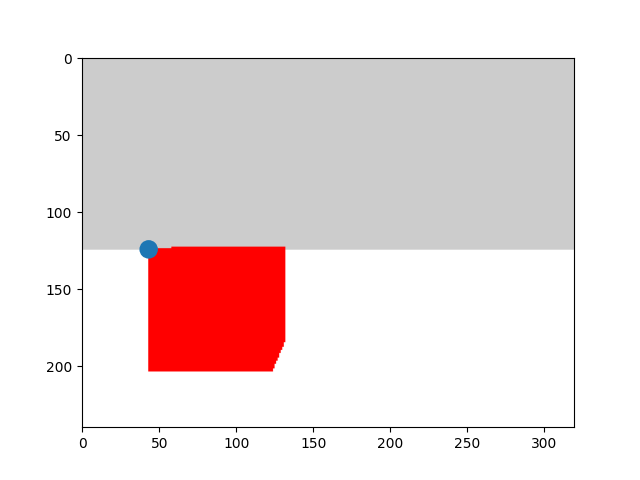
Projection World 2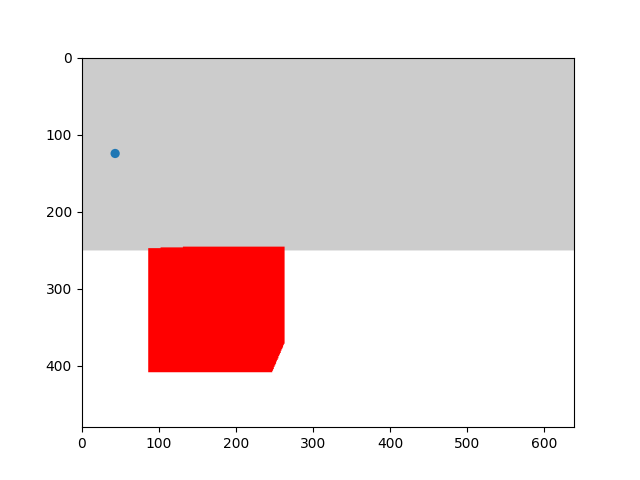
There are also two lower-level problems:
Here is the full code to produce the images above.
Code
```python from scenario import gazebo as scenario_gazebo import numpy as np import matplotlib.pyplot as plt from matplotlib.patches import Circle from scipy.spatial.transform import Rotation as R import ropy.transform as tf import ropy.ignition as ign def camera_parser(msg): image_msg = ign.messages.Image() image_msg.parse(msg[2]) image = np.frombuffer(image_msg.data, dtype=np.uint8) image = image.reshape((image_msg.height, image_msg.width, 3)) return image def camera_info_parser(msg): return ign.messages.CameraInfo().parse(msg[2]) gazebo = scenario_gazebo.GazeboSimulator(step_size=0.001, rtf=1.0, steps_per_run=1) assert gazebo.insert_world_from_sdf("./world1.sdf") gazebo.initialize() # Fix: available topics seem to only be updated at the end # of a step. This allows the subscribers to find the topic's # address gazebo.run(paused=True) # get extrinsic matrix camera = gazebo.get_world("camera_sensor").get_model("camera").get_link("link") cam_pos_world = np.array(camera.position()) cam_ori_world_quat = np.array(camera.orientation())[[1, 2, 3, 0]] cam_ori_world = R.from_quat(cam_ori_world_quat).as_euler("xyz") camera_frame_world = np.stack((cam_pos_world, cam_ori_world)).ravel() extrinsic_transform = tf.coordinates.transform(camera_frame_world) # scene objects box = gazebo.get_world("camera_sensor").get_model("box") with ign.Subscriber("/camera", parser=camera_parser) as camera_topic, ign.Subscriber( "/camera_info", parser=camera_info_parser ) as cam_info_topic: gazebo.run(paused=True) img = camera_topic.recv() # get intrinsic matrix cam_info = cam_info_topic.recv() rot = tf.coordinates.transform(np.array((0, 0, 0, -np.pi / 2, -np.pi / 2, 0))) projection = np.array(cam_info.projection.p).reshape((3, 4)) intrinsic_transform = np.matmul(projection, rot) # project corner box_corner = np.array(box.base_position()) + np.array((0.5, -0.5, 0.5)) pos_world = tf.homogenize(box_corner) pos_cam = np.matmul(extrinsic_transform, pos_world) pos_px_hom = np.matmul(intrinsic_transform, pos_cam) cube_pos_px = tf.cartesianize(pos_px_hom) # visualize fig, ax = plt.subplots(1) ax.imshow(img) ax.add_patch(Circle(cube_pos_px, radius=6)) plt.show() gazebo.close() ```To run this, a working
gym-ignition
andropy[ignition]
(not on pypi yet, but easy to install locally) installation are required.