Closed maxenko closed 2 years ago
Are you sure this is related to MozJpegSharp?
Thoughts:
What would be helpful to further diagnose your problem
@georg-jung thank you for quick response, I will investigate further based on your suggestions.
It works perfect under windows, so I am not entirely sure why resizing would affect it. Its likely the linux library like you're saying.
I am using standard .net for resizing:
let resizeByWidth (img:System.Drawing.Image) w =
let _w = (float)w
let _ratio = _w / (float)img.Width
let _h = Math.Max( 1.0, (float)img.Height * _ratio)
(new Bitmap(img, (int)_w, (int)_h)) :> System.Drawing.Image
which is called from:
let saveMozJpgThumb toPath stream resX =
async {
use img = Image.FromStream stream
use resized = resizeByWidth img resX
return! writeMozJpg resized toPath
}
Looking into other suggestions now, thank you.
It works perfect under windows, so I am not entirely sure why resizing would affect it
What I'm saying is that I could imagine that the resizing causes the pixelation on Linux, not saving using MozJpegSharp. Resizing can be done in many different ways. See i.e. here. Now, the code you posted might see how/which way resizing is done as an implementation detail. And the implementations of GDI+ on Windows and libgdiplus on Linux differ. Hope this helps.
@georg-jung you're correct, this has nothing to do with your library. Apologies for false alarm. I think the defaults are different between implementations.
This works:
let resizeByWidth (img:System.Drawing.Image) w =
let _w = (float)w
let _ratio = _w / (float)img.Width
let _h = Math.Max( 1.0, (float)img.Height * _ratio)
let bmp = new Bitmap((int)_w, (int)_h)
use g = Graphics.FromImage(bmp)
g.InterpolationMode <- InterpolationMode.High
let rect = new System.Drawing.Rectangle(0, 0, (int)_w, (int)_h)
g.DrawImage(img, rect);
bmp
I am running into pixilation issue when using MozJpegSharp from within docker deployment via standard MS template which is Debian.
When running compression from within VS on windows - results are good. However after deploying the same code to Docker, compressing any image appears to cause fairly noticeable pixilation.
I've tried changing parameters, but the results are the same.
Windows: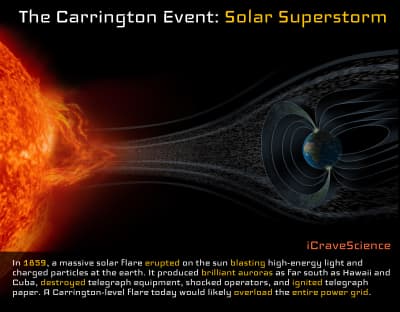
Linux: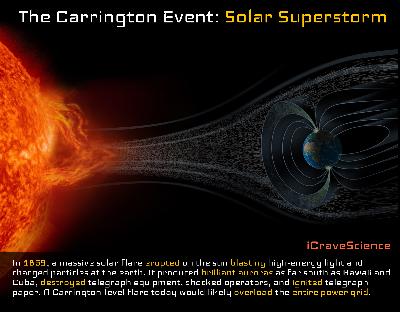
Source file: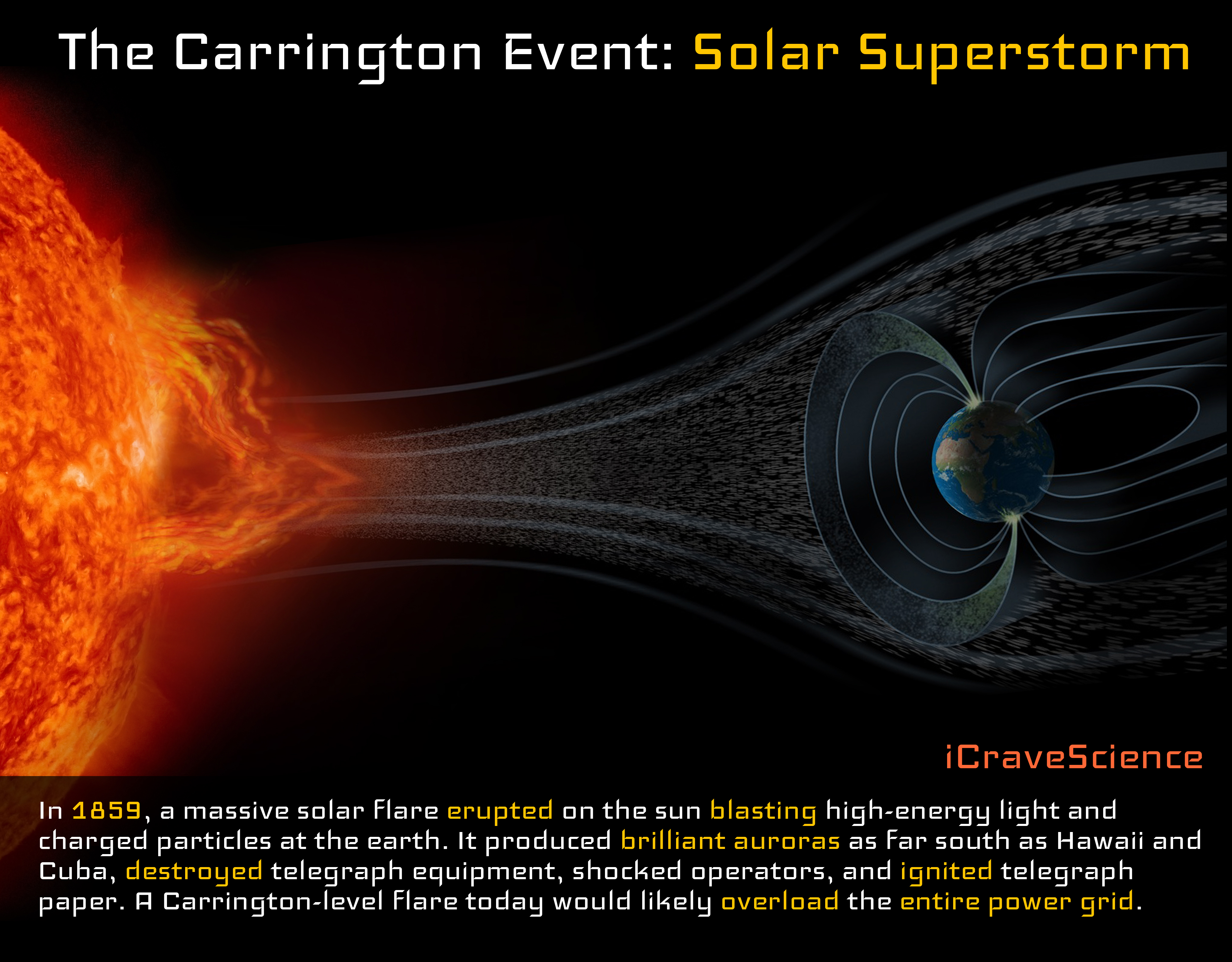
Any suggestions appreciated.
Encoding with (F#):
Dockerfile: