Open tofoli opened 6 years ago
As you can see I started only the first one on the list, but the events are returning to everyone.
A gif is the continuation of the other
Follow the source code
import React, { Component } from 'react'; import { View } from 'react-native' import TorrentStreamer from 'react-native-torrent-streamer' import Item from './Item'; export default class App extends Component { render() { return ( <View> <Item magnet="magnet:?xt=urn:btih:401b95a6de2ee3c99a166f77344e20eede090d78&dn=The.Big.Bang.Theory.S11E15.The.Novelization.Correlation.720p.AMZN.WEBRip.DDP5.1.x264-NTb%5Brartv%5D&tr=http%3A%2F%2Ftracker.trackerfix.com%3A80%2Fannounce&tr=udp%3A%2F%2F9.rarbg.me%3A2710&tr=udp%3A%2F%2F9.rarbg.to%3A2710" /> <Item magnet="magnet:?xt=urn:btih:6b82b6e3a2549de82f76071a0b59e370d4070338&dn=The.Big.Bang.Theory.S11E14.The.Separation.Triangulation.720p.AMZN.WEBRip.DDP5.1.x264-NTb%5Brartv%5D&tr=http%3A%2F%2Ftracker.trackerfix.com%3A80%2Fannounce&tr=udp%3A%2F%2F9.rarbg.me%3A2710&tr=udp%3A%2F%2F9.rarbg.to%3A2710" /> <Item magnet="magnet:?xt=urn:btih:773f1f4c2e6d354dab798e4a7d4d8179746bcecd&dn=The.Big.Bang.Theory.S11E13.WEB-DL.x264-ION10&tr=http%3A%2F%2Ftracker.trackerfix.com%3A80%2Fannounce&tr=udp%3A%2F%2F9.rarbg.me%3A2710&tr=udp%3A%2F%2F9.rarbg.to%3A2710" /> <Item magnet="magnet:?xt=urn:btih:772837cd094496818b21116332aed7385d609f75&dn=The.Big.Bang.Theory.S11E12.WEB-DL.x264-RARBG&tr=http%3A%2F%2Ftracker.trackerfix.com%3A80%2Fannounce&tr=udp%3A%2F%2F9.rarbg.me%3A2710&tr=udp%3A%2F%2F9.rarbg.to%3A2710" /> </View> ) } }
import React, { Component } from 'react'; import { View, Text, TouchableHighlight } from 'react-native' import TorrentStreamer from 'react-native-torrent-streamer' export default class Item extends Component { constructor(props) { super(props); this.state = { progress: 0, buffer: 0, downloadSpeed: 0, seeds: 0, }; this.onError = this.onError.bind(this); this.onStatus = this.onStatus.bind(this); this.onReady = this.onReady.bind(this); this.onStop = this.onStop.bind(this); this._handleStart = this._handleStart.bind(this); this._handleStop = this._handleStop.bind(this); } componentWillMount() { TorrentStreamer.addEventListener('error', this.onError) TorrentStreamer.addEventListener('status', this.onStatus) TorrentStreamer.addEventListener('ready', this.onReady) TorrentStreamer.addEventListener('stop', this.onStop) } onError(e) { console.log(e) } onStatus({ progress, buffer, downloadSpeed, seeds }) { this.setState({ progress, buffer, downloadSpeed, seeds }) } onReady(data) { console.log('ready', data); } onStop(data) { console.log('stop', data) } render() { const { progress, buffer, downloadSpeed, seeds } = this.state return ( <View style={{margin: 20}}> <TouchableHighlight onPress={this._handleStart.bind(this)} > <Text >Start Torrent!</Text> </TouchableHighlight> <TouchableHighlight onPress={this._handleStop.bind(this)} > <Text >Stop Torrent!</Text> </TouchableHighlight> {buffer ? <Text>Buffer: {buffer}</Text> : null} {downloadSpeed ? <Text>Download Speed: {(downloadSpeed / 1024).toFixed(2)} Kbs/seg</Text> : null} {progress ? <Text>Progress: {parseFloat(progress).toFixed(2)}</Text> : null} {seeds ? <Text>Seeds: {seeds}</Text> : null} </View> ); } private _handleStart() { TorrentStreamer.start(this.props.magnet); } _handleStop() { this.setState({ progress: 0, buffer: 0, downloadSpeed: 0, seeds: 0 }, () => { TorrentStreamer.stop() }); } }
As you can see I started only the first one on the list, but the events are returning to everyone.
A gif is the continuation of the other
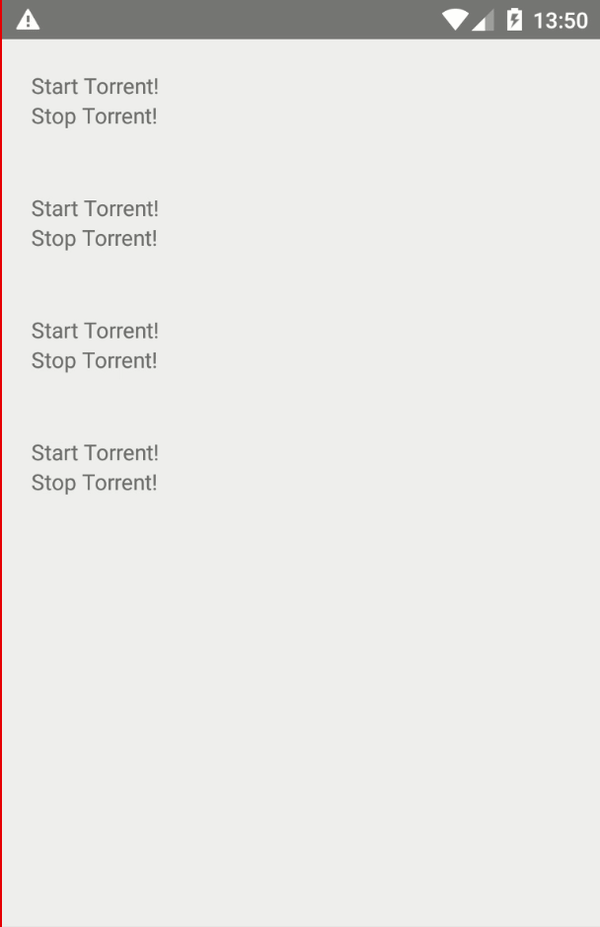
Follow the source code