Open SunboX opened 2 years ago
Here are both projects to try out: https://we.tl/t-lFjg2oIFPK
btw., the issue number is 404 🙈
Ciao @SunboX I am sorry for answering you so late. I do not have the hardware to replicate your setup now, but I suspect there may be a wrong timing configuration in the esp8266. Please try to tweak SWBB_READ_DELAY and SWBB_BIT_WIDTH on the ESP8266 side (the right value may be shorter or longer, start with SWBB_READ_DELAY, if you don't see packets after tweaking it you may need to go back to its original value and try to tweak SWBB_BIT_WIDTH). Here to edit those values: https://github.com/gioblu/PJON/blob/master/src/strategies/SoftwareBitBang/Timing.h#L198-L201 Try also different combinations of pins it may work better. Let me know how it goes and if you need any support.
@gioblu Thanks for your reply! Both were ESP32, not ESP8266. But thanks, I will try this and let you know the result!
Sorry @SunboX in this case you should modify the constants here: https://github.com/gioblu/PJON/blob/master/src/strategies/SoftwareBitBang/Timing.h#L207
@gioblu Great! Seems I have success using this configuration on both ESP32 using pin 25:
/* Heltech WiFi LoRa ESP32, generic ESP32 --------------------------------- */
#if defined(ESP32)
#if SWBB_MODE == 1
/* Added full support to MODE 1 - 28/06/2018
Working on pin: 12 and 25 */
#define SWBB_BIT_WIDTH 46
#define SWBB_BIT_SPACER 112
#define SWBB_ACCEPTANCE 40
#define SWBB_READ_DELAY -10
#endif
#endif
I was using this example: https://github.com/gioblu/PJON/tree/master/examples/ARDUINO/Local/SoftwareBitBang/PJONLocal/NetworkAnalysis
and uncommented for both (sender and receiver)
#define SWBB_MODE 1
and changed the pin for both to:
bus.strategy.set_pin(25);
Output:
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 570B
Bandwidth: 1710B/s
Data throughput: 1140B/s
Packets sent: 57
Fail (no acknowledge from receiver): 1
Busy (Channel is busy or affected by interference): 104
Delivery success rate: 98.25 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
Bandwidth: 1740B/s
Data throughput: 1160B/s
Packets sent: 58
Fail (no acknowledge from receiver): 0
Busy (Channel is busy or affected by interference): 0
Delivery success rate: 100.00 %
---------------------
Packet Overhead: 10B - Total: 580B
@SunboX I don't have a way to test it now, but your test result shows it is working perfectly. I am not sure how I did not spot this in the last test round.
@SunboX can I ask you to execute a test that would help me to verify your config? I would need to know if your config works also when ESP32 transmits to or receives from Arduino Nano/Uno or Mega. This would verify that the configuration found works properly in both cases (ESP to ESP and ESP to arduino)
Hey, yes. That's no problem. I should have some Arduino in one of my boxes. I can have a look in some hours which ones I have and can tell you.
Ok, I have those to work with:
Please tell me which one I should use and please send me the test code (GitHub Gist or so). I will send you the results.
Thank you very much @SunboX The Arduino duemilanove is OK. You can use the networkAnalysis sketch: https://github.com/gioblu/PJON/tree/master/examples/ARDUINO/Local/SoftwareBitBang/NetworkAnalysis
@gioblu Yesterday I tested the UNO (for some reason I could not flash my Duemilanove with macOS Monterey) together with the ESP32. It did not work. ;) I will investigate when I have a bit more time.
try to remove the delay(1) from the main loop, put the send() to a counter to trigger it, and move all the serial output onto an event or counter to trigger it.
you have alot happening in the main loop that might impact update() from running freely as bitbang requires.
you are using send() very frequently which is non-blocking and has its own repeats, you might be maxing out a buffer or the bandwidth available on the wire.
Hi,
I'm running a simple test, two ESP32 connected with one cable (GPIO 25 <-> GPIO 25), GND and 5V are also connected directly.
This is the code running on ESP32 "A":
And this is the code running on ESP32 "B":
Both are compiled and uploaded using PlatformIO with this ini file:
The Oszilloskope is showing some communication: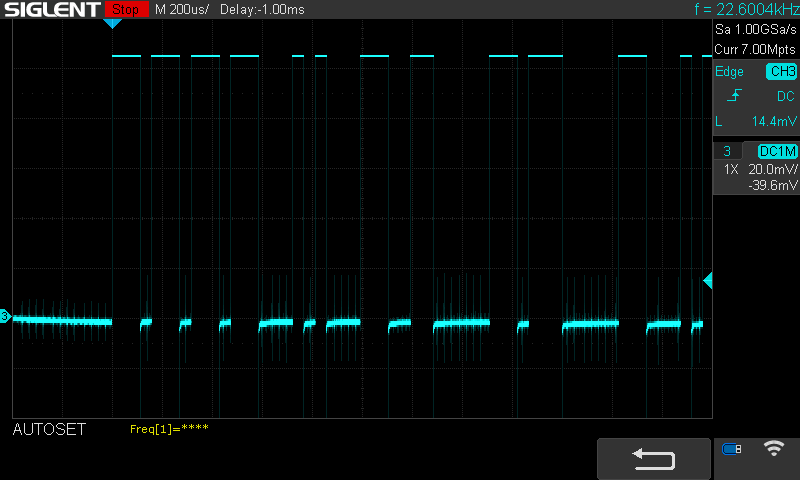
But no packet is arriving at any of both ESP32. Console log:
What's wrong? Why does no packet arrive?