Closed AsDeadAsADodo closed 11 months ago
why no answer?
you mean this?
currently I'm developing a CSG WASM tool in CPP that is suitable for three.js. The inspiration comes from "three-bvh-csg". There are still many issues to address at the moment, but the overall situation is promising.
currently I'm developing a CSG WASM tool in CPP that is suitable for three.js. The inspiration comes from "three-bvh-csg". There are still many issues to address at the moment, but the overall situation is promising.
Thata is cool, looking fwd seeing it when it is ready for public.
Did you also check manifold csg library ? It is cpp and has wasm, very fast and already does very well.
I'd appreciate if users submitted fiddles or live examples in the future so these issues are easier to test with new releases. Over the last several releases of three-mesh-bvh and three-bvh-csg there have been improvements to the stability of these types of operations so I'm going to close this unless it can be shown that it's still an issue.
The inspiration comes from "three-bvh-csg".
Looking forward to seeing it!
I'll just skip the set up part ,directly show the pics and codes.
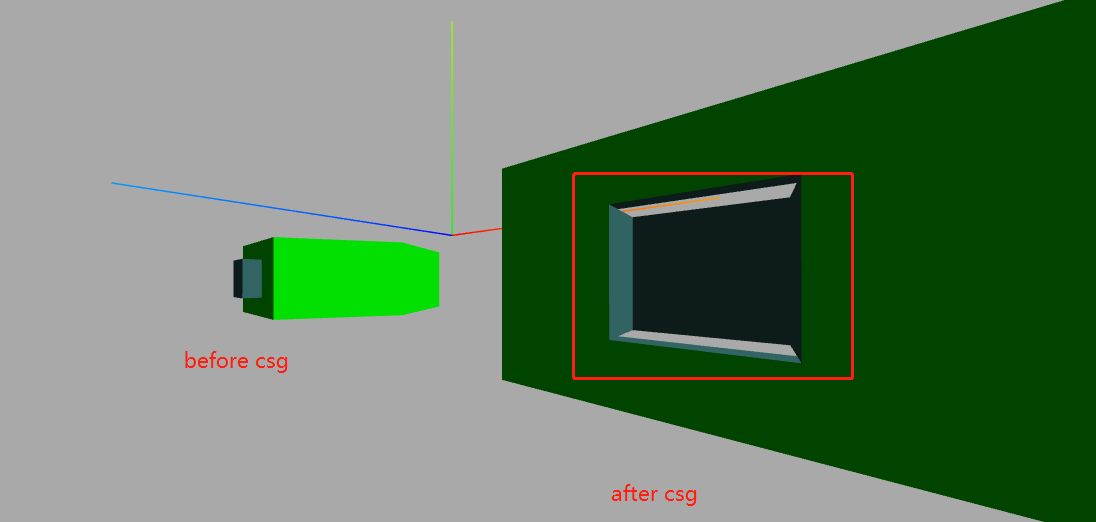
After csg substract , the result also obtain some faces and material of the box.
Console print
Trianlges are coplanar which does not support an output edge