Closed ZainlessBrombie closed 5 years ago
The problem is that you have named a function the same as a variable. snoise
is the name of your simplex noise lookup function and of your variable containing the value of the simplex noise lookup. Because they have the same name, the shader doesn't compile.
On my system I get the following error message.
0:1250(24): error: no function with name 'm_snoise'
0:1250(24): error: operands to arithmetic operators must be numeric
0:1250(23): error: operands to arithmetic operators must be numeric
0:1250(22): error: operands to arithmetic operators must be numeric
0:1250(16): error: no matching function for call to `atan(error)'; candidates are:
0:1250(16): error: float atan(float)
0:1250(16): error: vec2 atan(vec2)
0:1250(16): error: vec3 atan(vec3)
0:1250(16): error: vec4 atan(vec4)
0:1250(16): error: float atan(float, float)
0:1250(16): error: vec2 atan(vec2, vec2)
0:1250(16): error: vec3 atan(vec3, vec3)
0:1250(16): error: vec4 atan(vec4, vec4)
0:1250(16): error: operands to arithmetic operators must be numeric
The corresponding lines from the shader dump are:
1249: float m_snoise=(atan((((m_snoise((vec3(m_xcoord, m_ycoord, m_zcoord)*10.0))*2.0)-1.0)*3.0))+1.0);
1250: float m_test=(atan((((m_snoise((vec3(m_xcoord, m_ycoord, m_zcoord)*10.0))*2.0)-1.0)*3.0))+1.0);
To fix this issue, either rename your function, or rename the snoise
variable.
@clayjohn If some GLSL shader compilers have trouble with this, the Godot shader language compiler should probably throw an error if a variable has the same name as a function.
@Calinou That is good idea. Maybe you could rename the issue to say that?
@clayjohn I think a new issue should be created about that; it could become confusing if this issue was renamed. I'll close this one when the other issue is opened.
Edit: Done, see https://github.com/godotengine/godot/issues/27363.
Godot version: 3.1 stable
OS/device including version: Linux Ubuntu 18, 4.15.0-46-generic, GTX 1060, Driver 410.48
Issue description: I was writing a shader that was supposed to render a planet. The shader is highly complex, computing a simplex noise for every fragment, as it was only supposed to be used for cinematic rendering. When calling the simplex noise function twice instead of once (and not using the result nor references), the whole screen glitches out. Could it have been that the shader hit some execution time threshold?
Steps to reproduce: Use the shader below on a sphere with 1000 radial segments and 1000 rings:
This is what the glitch looks like: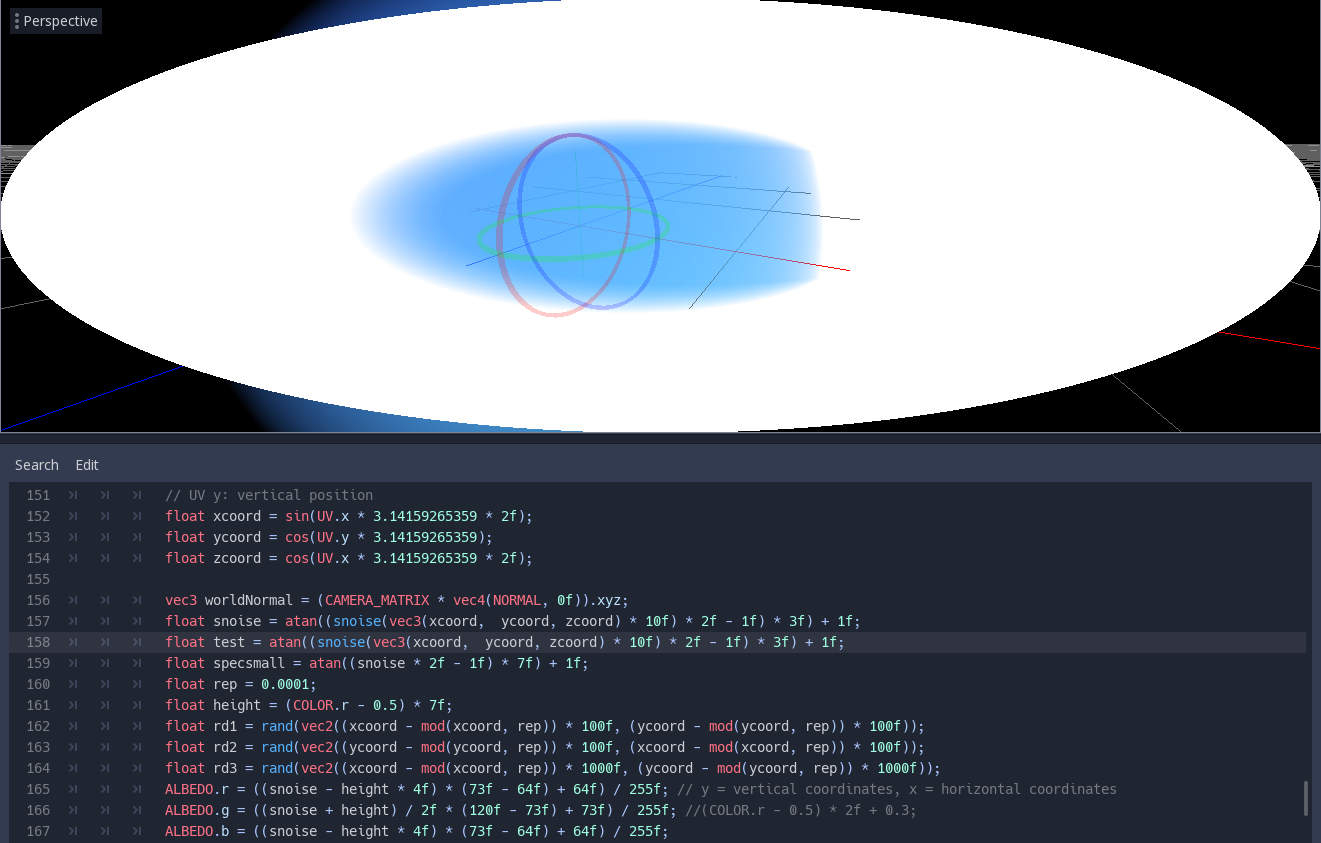