Closed dkarv closed 1 year ago
This happens because on the first load of a webview it loads the whole chromium engine and that blocks Compose's applyChanges from happening. It is something I want to fix but haven't found a workaround for yet. Out of interest, does setting a Modifier.alpha(0.99f)
fix the problem for you?
Setting the alpha on the WebView fixed it on 3 out of 6 devices we tried. It works:
Does not work:
Based on the data it seems the fix does not work on Android 10 and 11 (we did not test Android 12).
I have similar issues with the following snippet:
val state = rememberWebViewState("https://www.notion.so")
val navigator = rememberWebViewNavigator()
Column(Modifier.background(Color.Red)) {
Row(
modifier = Modifier
.height(56.dp)
.fillMaxWidth(),
horizontalArrangement = Arrangement.Start,
verticalAlignment = Alignment.CenterVertically
) {
IconButton(onClick = {}) {
Icon(
Icons.Default.ArrowBack,
contentDescription = "go back"
)
}
}
WebView(
state = state,
navigator = navigator,
onCreated = {
it.settings.javaScriptEnabled = true
}
)
}
@bentrengrove Could you confirm that it's the same root cause?
Here is a project reproducing the issue: https://github.com/nicolashaan/accompanist-webview-issue-repro
I have just encountered a similar issue. I notice that setting a height on the WebView fixes the problem, e.g.
WebView( modifier = Modifier.height(300.dp),
I'm not a Compose expert, but this behaviour makes me assume that Column doesn't render its content until all Composables are finished measuring. Since the WebView is still loading it doesn't yet know its height, so this blocks the parent Column from rendering.
This issue is stale because it has been open 30 days with no activity. Remove stale label or comment or this will be closed in 5 days.
This issue is stale because it has been open 30 days with no activity. Remove stale label or comment or this will be closed in 5 days.
I found by chance similar issue described on StackOverflow. One answer suggested to explicitly set the WebView's layer type to SOFTWARE like this:
webView.setLayerType(View.LAYER_TYPE_SOFTWARE, null)
This solved all visible issue for us.
Here is the original answer: https://stackoverflow.com/a/75247593
With webView.setLayerType(View.LAYER_TYPE_SOFTWARE, null)
, the content of webView doesn't show after load for me. Still looking for other solution
This solves the issue:
AndroidView( modifier = Modifier .fillMaxWidth() .weight(1f), factory = { WebView(context).apply { setLayerType(View.LAYER_TYPE_SOFTWARE, null) webViewClient = WebViewClient() loadUrl(item.link) } })
setLayerType(View.LAYER_TYPE_SOFTWARE, null)
does not work if the content has GIFs
I know this issue is closed but my search lead me here first, so I want to make it easier for others to find this.
It seems like to correct solution is to set clipToOutline = true
on the webview instance. See the issue tracker for reference and the source code for the current recommendation.
@bentrengrove would you agree with that?
Yes, I believe that is correct
Below code worked for me:
AndroidView(modifier = modifier, factory = {
WebView(it).apply {
//setBackgroundColor(Color.Blue.toArgb())
clipToOutline = true
}
}, update = {
it.loadDataWithBaseURL(null, html, "text/html", "UTF-8", "")
})
Description While the Accompanist WebView is loading a url (we show a CircularProgressIndicator) it stops other Composable content from showing up. The other content is just white/blank. Please checkout this screencast of the issue: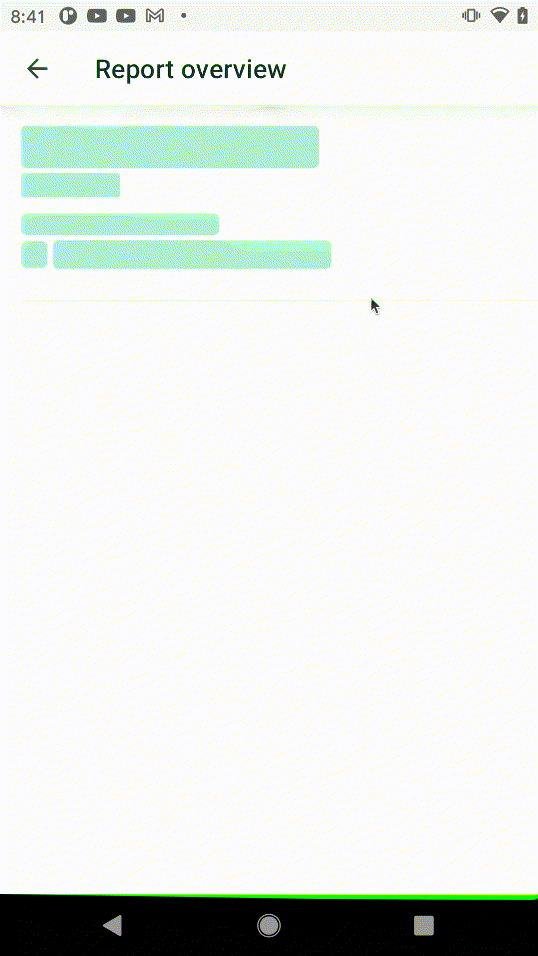
Steps to reproduce
Expected behavior
Additional context The content above is not hidden or covered. It shows up as supposed in the Layout Inspector, it is just not shown on the real screen: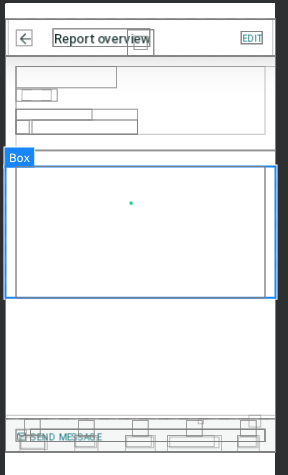
It seems like the WebView is internally blocking the other Compose rendering.
Compose Version: 1.3.1 Accompanist Version: 0.27.1
The WebView creation:
The other content is in a Column, together with the WebView. I'm happy about any help or workarounds for this issue. I can provide more information if needed.