Open thoffart opened 5 years ago
This is caused by invoking _textPainter.layout() in the text_element.dart file.
I don't know somehow, the maxWidth passed to it is negative. And inside of this function, clamp() is called with clamp(minWidth, maxWidth), of which minWidth is set to 0.0 as default. caused this problem.
So, I just simply added a condition to get around this. Hope this can help and the team fix this in the future.
if (maxWidth == null || maxWidth >= 0) { _textPainter.layout(maxWidth: maxWidth?.toDouble() ?? double.infinity); } else { _textPainter.layout(maxWidth: double.infinity); }
This is caused by invoking _textPainter.layout() in the text_element.dart file. I don't know somehow, the maxWidth passed to it is negative. And inside of this function, clamp() is called with clamp(minWidth, maxWidth), of which minWidth is set to 0.0 as default. caused this problem. So, I just simply added a condition to get around this. Hope this can help and the team fix this in the future.
if (maxWidth == null || maxWidth >= 0) { _textPainter.layout(maxWidth: maxWidth?.toDouble() ?? double.infinity); } else { _textPainter.layout(maxWidth: double.infinity); }
I came around with the same solution a time ago, but instead of setting the maxWidth
to infinity when it's value is negative, I convert it to his absolute value. I am having no issues with this solution in my project, that is in production a couple of months.
still no work arround or fix with this?
The pie chart breaks when the legend position is set to the bottom and the arc position is set to automatic. This seems to be related to the outside arc overlapping the legend at the bottom. Causing this bug to depend on the bounds and chart values.
Environment
Screenshot: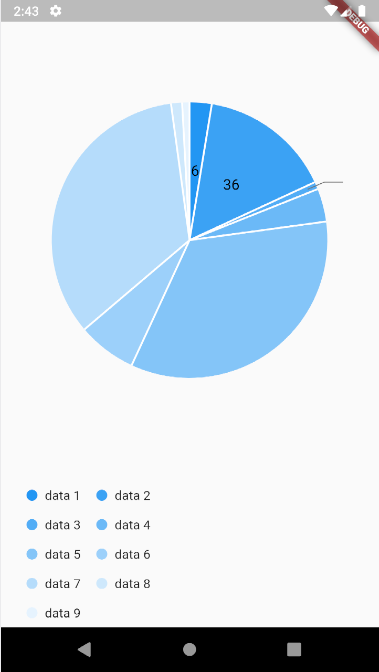
Error:
main.dart:
pie-chart-widget.dart: