Closed Molay closed 5 years ago
My bad :(
https://stackoverflow.com/questions/15364517/pixelstoreigl-unpack-flip-y-webgl-true
there is no UNPACK_PREMULTIPLY_ALPHA_WEBGL and UNPACK_FLIP_Y_WEBGL in OpenGL ES.
In implementation, WebGL call OpenGL ES to implement js WebGL call, and for these two parameters, WebGL will process on CPU before call OpenGL ES API, which means WebGL using memcpy to flip the image(if flipY is true), then call glTexImage2D.
Summary:
js call gl.pixelStorei(gl.UNPACK_FLIP_Y_WEBGL, true). WebGL store it as m_unpackFlipY.
js call gl.texImage2D, WebGL check m_unpackFlipY, if true, flip the image in memory, then call glTexImage2D
I'll try to create a proxy to solve this problem.
I'm trying to use node-gles with three.js and its WebGLRenderTarget class:
but an error has occurred:
In the description of the page WebGLRenderingContext.pixelStorei, the second parameter of method WebGLRenderingContext.pixelStorei can be GLint, GLboolean or GLenum, however the line 3720 of binding/webgl_rendering_context.cc file seems to only support GLint:
https://github.com/google/node-gles/blob/b405d08f114b8a970bc83ec47e95c0786a6ec5e0/binding/webgl_rendering_context.cc#L3720
Maybe using Texture will also have the same problem? I will try it.Using textures will also have the same problem and more. I try to modify the "WebGLRenderingContext::PixelStorei" method to support gl.UNPACK_FLIP_Y_WEBGL with GLboolean value, but it didn't work at all, whatever true or false.(gl.UNPACK_FLIP_Y_WEBGL=false) Expectation: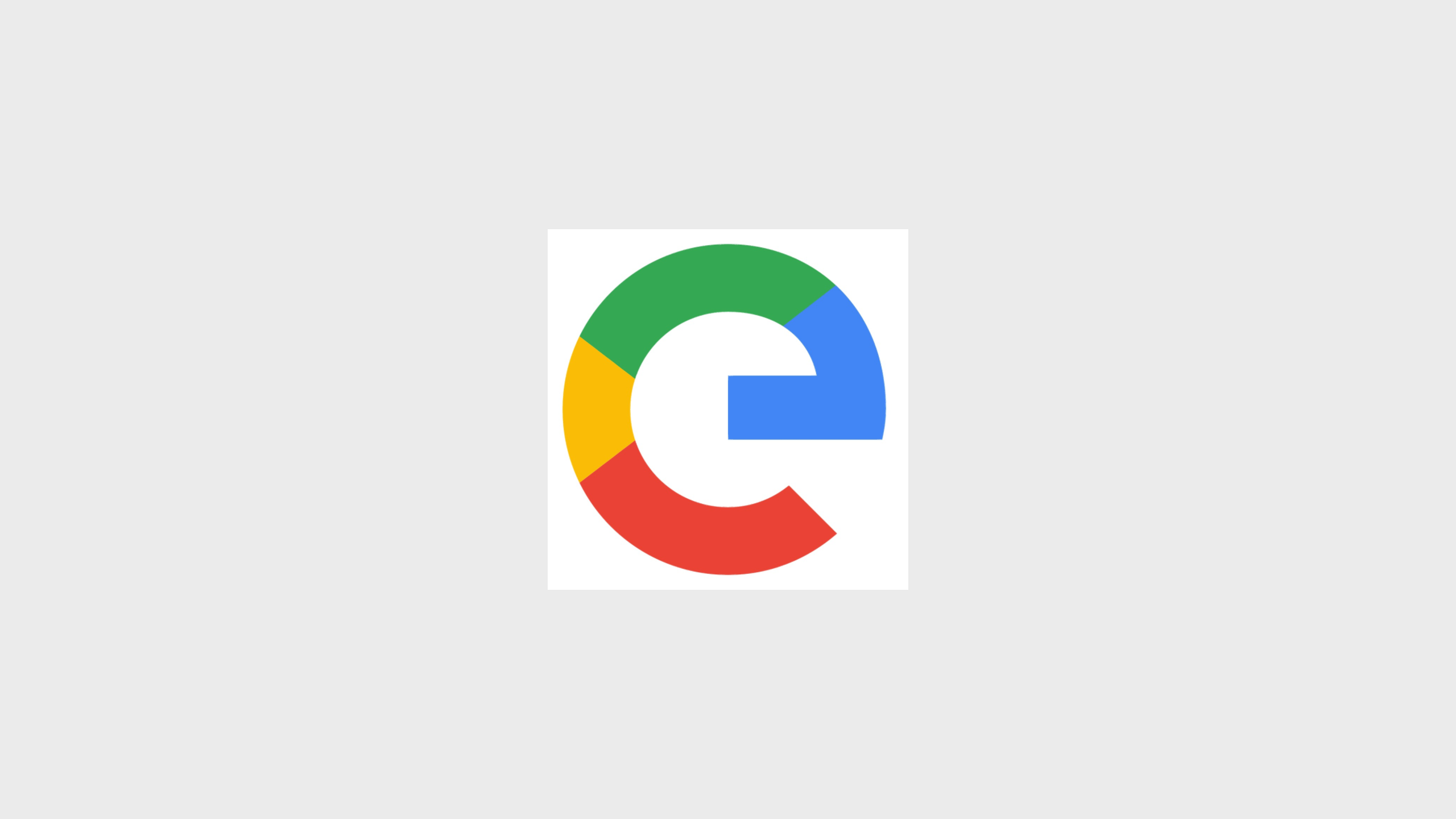
(gl.UNPACK_FLIP_Y_WEBGL=false) Result: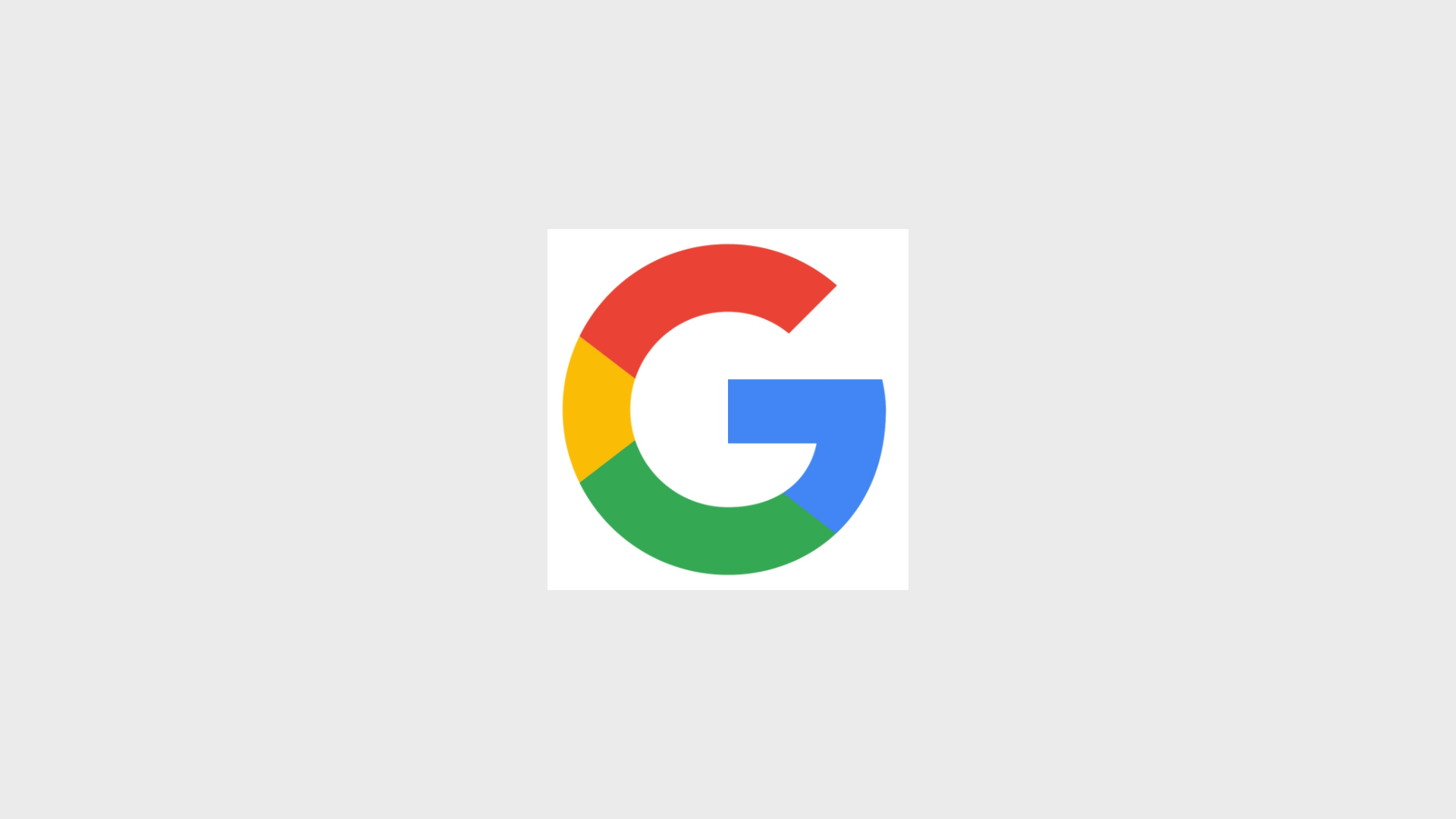
macOS 10.14.6, node.js 11.13.0, with node-gles 0.0.14 with three.js 0.107.0