Open sufianetaouil opened 2 years ago
The coverage map containing null bytes is normal, that's because you haven't passed any -coverage_module
at that point. The debug log for offset 0x1220 looks correct, but not for the other one.
Some questions that might help
-call_convention
?Adding also @hardik05 who I see is author of your test target and might have some ideas.
Thank you for your reply @ifratric, completely removing the call convention argument did it!
It's a bit weird because I was following a Youtube tutorial, and the source code I used is provided by the creator, so the argument worked fine for him but not me, but hey, I can't complain, just glad I have it running.
Thank you again!
This is my first try of AFL, but I'm unable to have it running properly, here is the source of the program I'm fuzzing:
The main function is situated is at offset 0x1220 and ProcessImage is at 0x1060.
Here is the result of debug with offset 0x1220 (main function):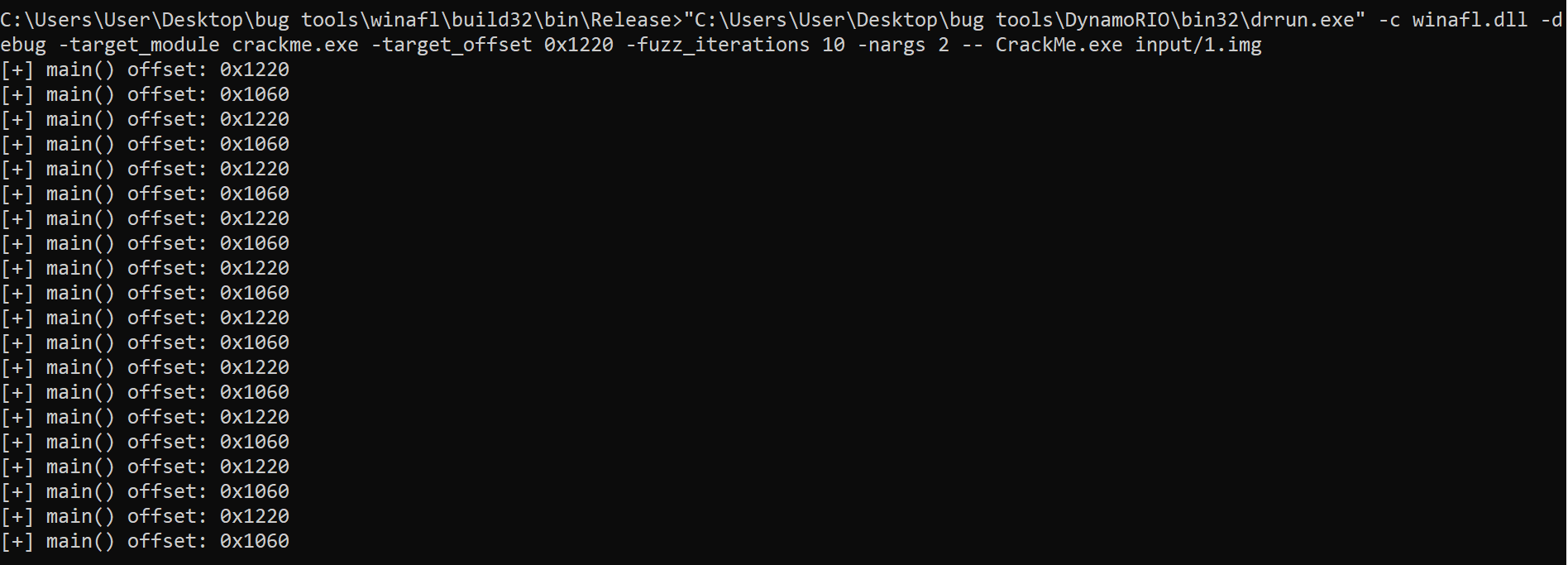
The coverage map is full of null bytes, seems wrong?
Here is the result of debug with offset 0x1060 (ProcessImage function):
The coverage map again contains only null bytes.
Running afl-fuzz against both offsets returns the following: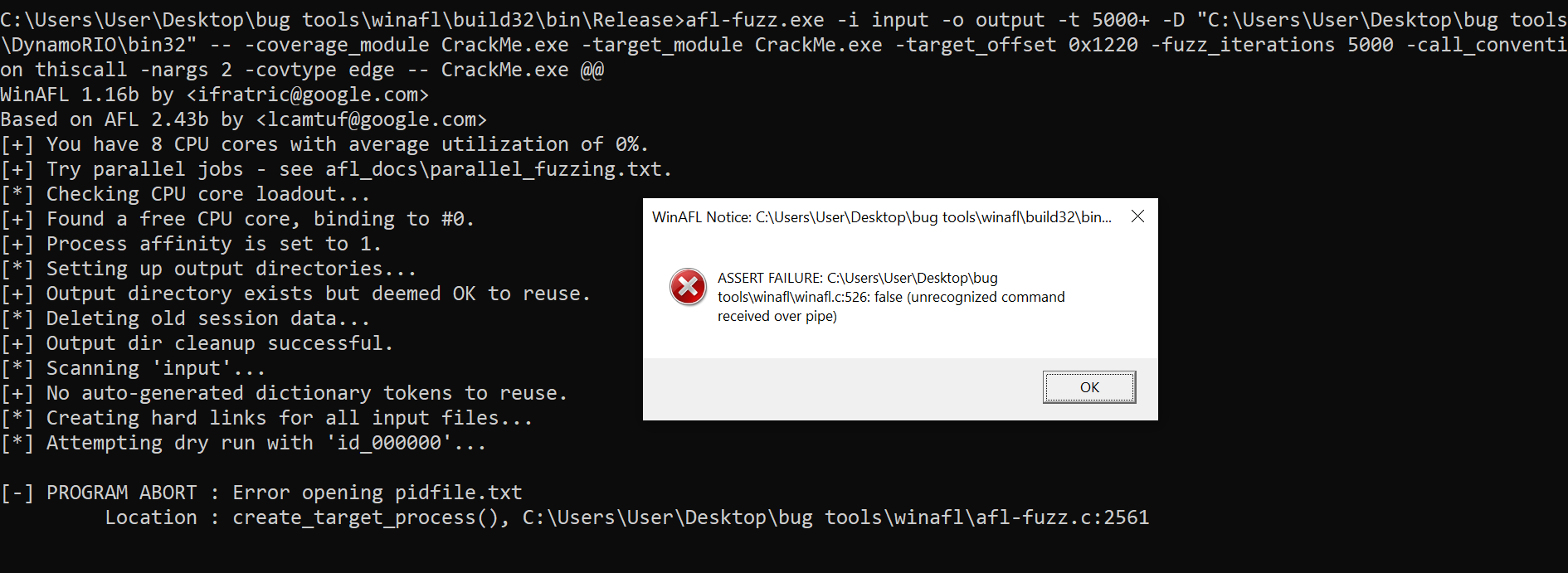
Here is the used command:
afl-fuzz.exe -i input -o output -t 5000+ -D "C:\Users\User\Desktop\bug tools\DynamoRIO\bin32" -- -coverage_module CrackMe.exe -target_module CrackMe.exe -target_offset 0x1060 -fuzz_iterations 5000 -call_convention thiscall -nargs 2 -covtype edge -- CrackMe.exe @@
Any ideas? Thank you