OSGL (Open Source General Library) is a set Java tool kit libraries. It is designed for concise and expressive coding.
This is the first article about OSGL art of coding series, and we will introduce how to use OSGL tool Img utility to manipulate images.
Stream based operation framework
The Img utility applied a stream based operation framework so that we can concatenate multiple operations to an image and the final result will be a combination of operations.
Before we start, let's take a look at our three original images, and we call them img1, img2 and img3:
img1
img2
img3
Get the image sources
We will use the following factory method to get the images in the following part of this blog:
There are two different types of resizing, first one is resize by giving absolute width and height of the new image dimension; and the second is to resize by a factor which applied to both widht and height.
OSGL (Open Source General Library) is a set Java tool kit libraries. It is designed for concise and expressive coding.
This is the first article about OSGL art of coding series, and we will introduce how to use OSGL tool Img utility to manipulate images.
Stream based operation framework
The Img utility applied a stream based operation framework so that we can concatenate multiple operations to an image and the final result will be a combination of operations.
Before we start, let's take a look at our three original images, and we call them
img1
,img2
andimg3
:img1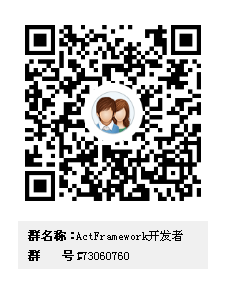
img2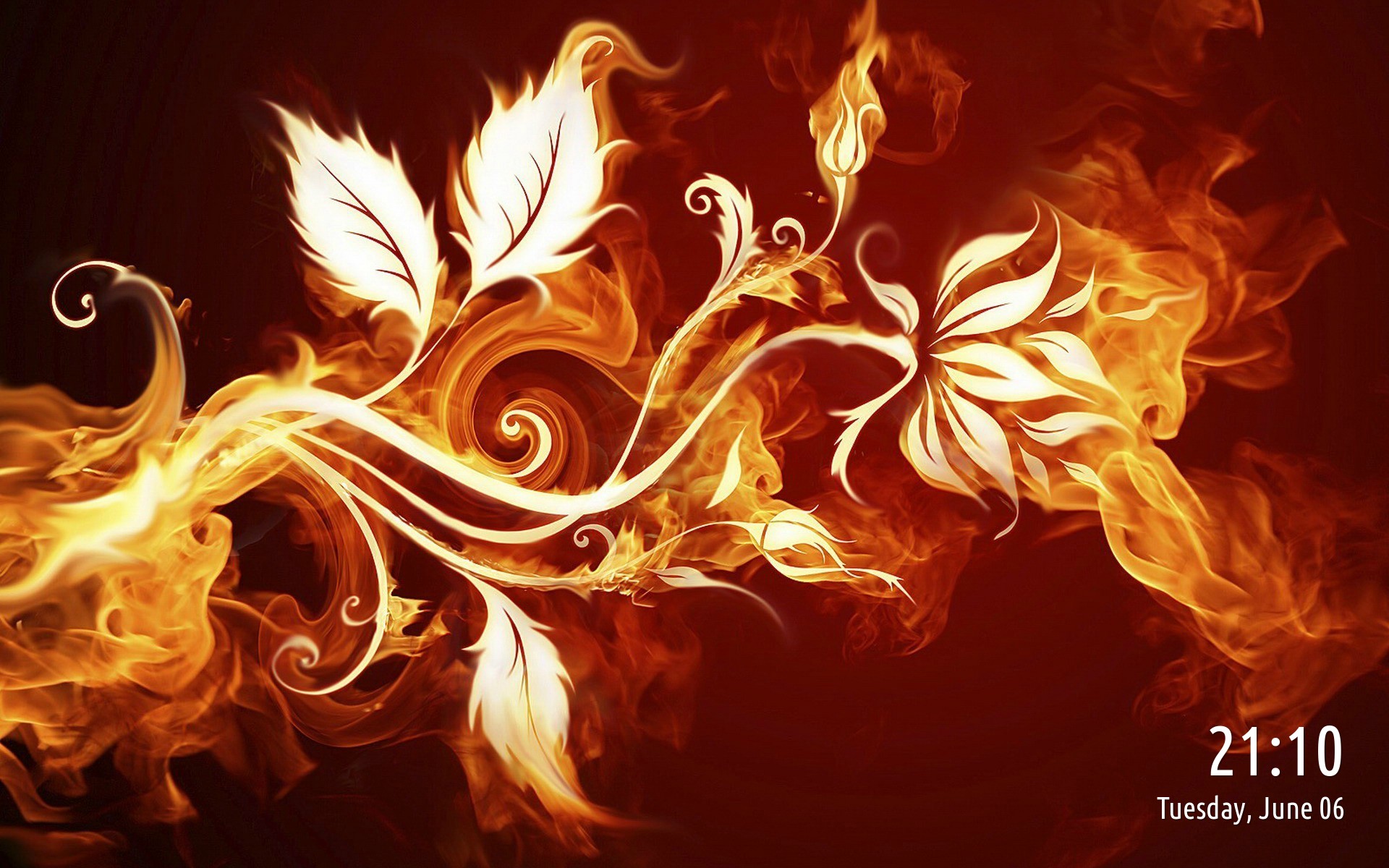
img3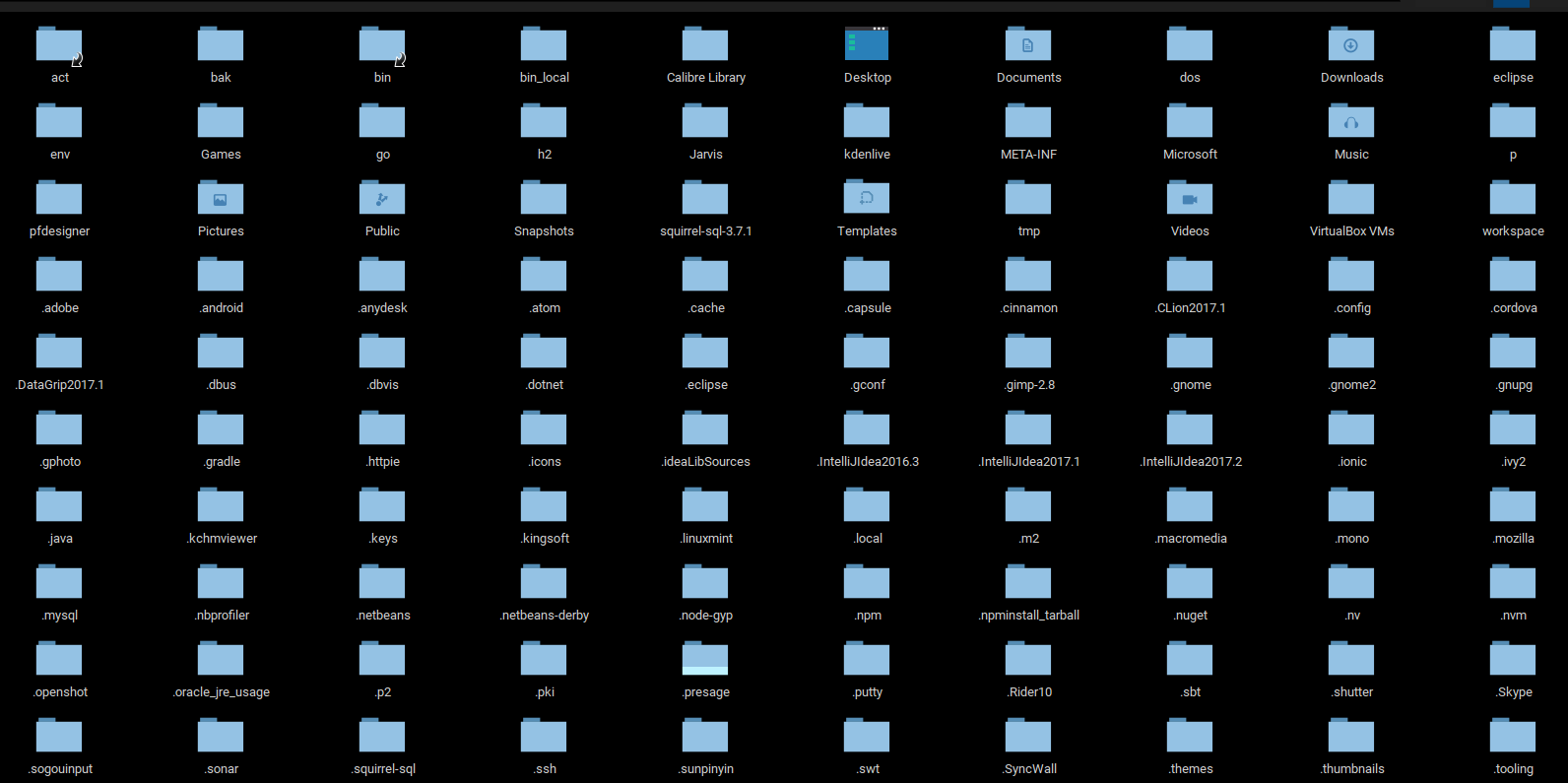
Get the image sources
We will use the following factory method to get the images in the following part of this blog:
1. Crop image
We will crop img1 starting from
(30, 30)
to(100, 100)
, here is how we did it:2. Resize image
There are two different types of resizing, first one is resize by giving absolute width and height of the new image dimension; and the second is to resize by a factor which applied to both widht and height.
We still use
img1
as the testbed:2.1 resize to given height and width:
2.2 resize by scale
We can also shrink image by scale using
resize
operator. This time we will operateimg2
instead ofimg1
2.3 resize and keep ratio
Sometimes we want to specify the width or height but still want to keep the height/width ratio, here is how we achieve it:
3. Watermark an image
To add a watermark to an image with default settings:
It also support water mark with different settings, e.g, the following code put watermark 200 pixels up and applied it with dark gray colour:
4. Flipping
We support flip image horizontally (default) or vertically
5. Blurring
Checkout blur with different levels and their effects:
6. Concatenate images
Concatenate veritically without scale adjustment:
Concatenate three images:
Concatenate three images with a different organization:
7. Multiple processing with pipeline:
Summary
This blog demonstrates how to use OSGL Img utility to process your images including:
To get OSGL project add the following dependency to your
pom.xml
file:At the moment the
osgl-tool.version
is1.10.1
.